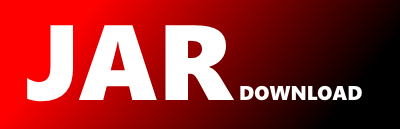
de.gold.scim.common.response.ErrorResponse Maven / Gradle / Ivy
The newest version!
package de.gold.scim.common.response;
import java.util.Collections;
import java.util.Optional;
import com.fasterxml.jackson.databind.JsonNode;
import de.gold.scim.common.constants.AttributeNames;
import de.gold.scim.common.constants.HttpStatus;
import de.gold.scim.common.constants.SchemaUris;
import de.gold.scim.common.exceptions.InternalServerException;
import de.gold.scim.common.exceptions.ResponseException;
import de.gold.scim.common.exceptions.ScimException;
import lombok.Getter;
import lombok.extern.slf4j.Slf4j;
/**
* author Pascal Knueppel
* created at: 14.10.2019 - 20:58
*
* represents a SCIM error response
*/
@Slf4j
public class ErrorResponse extends ScimResponse
{
/**
* the exception that should be turned into a SCIM error response
*/
@Getter
private ScimException scimException;
public ErrorResponse(JsonNode responseNode)
{
super(responseNode);
setSchemas(Collections.singletonList(SchemaUris.ERROR_URI));
this.scimException = new ResponseException(getDetail().orElse(null), getStatus(), getScimType().orElse(null));
}
public ErrorResponse(ScimException scimException)
{
super(null);
this.scimException = scimException;
if (HttpStatus.INTERNAL_SERVER_ERROR == getHttpStatus())
{
log.error(scimException.getMessage(), scimException);
}
else
{
log.debug(scimException.getMessage(), scimException);
}
setSchemas(Collections.singletonList(SchemaUris.ERROR_URI));
setStatus(scimException.getStatus());
setDetail(scimException.getDetail());
setScimType(scimException.getScimType());
}
/**
* The HTTP status code (see Section 6 of [RFC7231]) expressed as a JSON string. REQUIRED.
*/
public int getStatus()
{
return getIntegerAttribute(AttributeNames.RFC7643.STATUS).orElseThrow(() -> {
return new InternalServerException("the http 'status' is a mandatory attribute", null, null);
});
}
/**
* The HTTP status code (see Section 6 of [RFC7231]) expressed as a JSON string. REQUIRED.
*/
public void setStatus(int status)
{
setAttribute(AttributeNames.RFC7643.STATUS, status);
}
/**
* A SCIM detail error keyword. See Table 9. OPTIONAL.
*/
public Optional getScimType()
{
return getStringAttribute(AttributeNames.RFC7643.SCIM_TYPE);
}
/**
* A SCIM detail error keyword. See Table 9. OPTIONAL.
*/
public void setScimType(String scimType)
{
setAttribute(AttributeNames.RFC7643.SCIM_TYPE, scimType);
}
/**
* A detailed human-readable message. OPTIONAL.
*/
public Optional getDetail()
{
return getStringAttribute(AttributeNames.RFC7643.DETAIL);
}
/**
* A detailed human-readable message. OPTIONAL.
*/
public void setDetail(String detail)
{
setAttribute(AttributeNames.RFC7643.DETAIL, detail);
}
/**
* {@inheritDoc}
*/
@Override
public int getHttpStatus()
{
return scimException.getStatus();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy