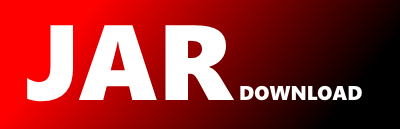
io.github.cdimascio.dotenv.Dotenv.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-dotenv Show documentation
Show all versions of java-dotenv Show documentation
Environment based config for the JVM
The newest version!
/**
* Copyright (c) Carmine DiMascio 2017 - 2020
* License: MIT
*/
package io.github.cdimascio.dotenv
import io.github.cdimascio.dotenv.internal.DotenvParser
import io.github.cdimascio.dotenv.internal.DotenvReader
import java.util.Collections
/**
* Dotenv
* @see The complete dotenv documentation
*/
abstract class Dotenv {
/**
* The dotenv instance
*/
companion object Instance {
/**
* Configure dotenv
* @return A dotenv builder
*/
@JvmStatic
fun configure(): DotenvBuilder = DotenvBuilder()
/**
* Load the the contents of .env into the virtual environment.
* Environment variables in the host environment override those in .env
*/
@JvmStatic
fun load(): Dotenv = DotenvBuilder().load()
}
/**
* Returns the value for the specified environment variable
*
* @param envName The environment variable name
*/
abstract operator fun get(envName: String): String?
/**
* Returns all environment entries
*/
abstract fun entries(): Set
/**
* Returns all environment entries filtered by {@link DotenvEntriesFilter}
*/
abstract fun entries(filter: DotenvEntriesFilter): Set
/**
* Returns the value for the environment variable, or the default value if absent
*
* @param envName The environment variable name
* @param defValue The default value
*/
fun get(envName: String, defValue: String): String {
return get(envName) ?: defValue
}
}
/**
* Dotenv exception
*/
class DotEnvException(message: String) : Exception(message)
/**
* Constructs a new DotenvBuilder
*/
class DotenvBuilder internal constructor() {
private var filename = ".env"
private var directoryPath = "./"
private var systemProperties = false
private var throwIfMissing = true
private var throwIfMalformed = true
/**
* Sets the directory containing the .env file
* @param path The path
*/
fun directory(path: String = directoryPath) = apply {
directoryPath = path
}
/**
* Sets the name of the .env file. The default is .env
* @param name The filename
*/
fun filename(name: String = ".env") = apply {
filename = name
}
/**
* Do not throw an exception when .env is missing
*/
fun ignoreIfMissing()= apply {
throwIfMissing = false
}
/**
* Do not throw an exception when .env is malformed
*/
fun ignoreIfMalformed() = apply {
throwIfMalformed = false
}
/**
* Adds environment variables into system properties
*/
fun systemProperties() = apply {
systemProperties = true
}
/**
* Load the contents of .env into the virtual environment
*/
fun load(): Dotenv {
val reader = DotenvParser(
DotenvReader(directoryPath, filename),
throwIfMalformed,
throwIfMissing)
val env = reader.parse()
if (systemProperties) {
env.forEach { System.setProperty(it.first, it.second) }
}
return DotenvImpl(env)
}
}
enum class DotenvEntriesFilter {
DECLARED_IN_ENV_FILE
}
/**
* A dotenv entry containing a key, value pair
*/
data class DotenvEntry(val key: String, val value: String)
private class DotenvImpl(envVars: List>) : Dotenv() {
private val map = envVars.associateBy({ it.first }, { it.second })
private val set: Set = Collections.unmodifiableSet(
buildEnvEntries().map { DotenvEntry(it.key, it.value) }.toSet()
)
override fun entries() = set
override fun entries(filter: DotenvEntriesFilter) = map.map { DotenvEntry(it.key, it.value) }.toSet()
override fun get(envName: String): String? = System.getenv(envName) ?: map[envName]
private fun buildEnvEntries(): Map {
val envMap = map.toMap(mutableMapOf())
System.getenv().entries.forEach {
envMap[it.key] = it.value
}
return envMap
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy