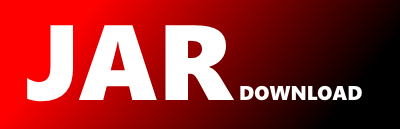
io.github.cdklabs.cdk.codepipeline.extensions.PipelineWithChangeControl Maven / Gradle / Ivy
Show all versions of cdk-codepipeline-extensions Show documentation
package io.github.cdklabs.cdk.codepipeline.extensions;
/**
* (experimental) A pipeline with a change controller.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.72.0 (build 4b8828b)", date = "2022-12-24T00:13:28.729Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = io.github.cdklabs.cdk.codepipeline.extensions.$Module.class, fqn = "@cdklabs/cdk-codepipeline-extensions.PipelineWithChangeControl")
public class PipelineWithChangeControl extends software.constructs.Construct {
protected PipelineWithChangeControl(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected PipelineWithChangeControl(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public PipelineWithChangeControl(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull io.github.cdklabs.cdk.codepipeline.extensions.PipelineWithChangeControlProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* (experimental) A fluent builder for {@link io.github.cdklabs.cdk.codepipeline.extensions.PipelineWithChangeControl}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final io.github.cdklabs.cdk.codepipeline.extensions.PipelineWithChangeControlProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new io.github.cdklabs.cdk.codepipeline.extensions.PipelineWithChangeControlProps.Builder();
}
/**
* (experimental) The calendar used for determining time windows.
*
* @return {@code this}
* @param changeControlCalendar The calendar used for determining time windows. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder changeControlCalendar(final io.github.cdklabs.cdk.codepipeline.extensions.Calendar changeControlCalendar) {
this.props.changeControlCalendar(changeControlCalendar);
return this;
}
/**
* (experimental) The schedule on which to check the calendar.
*
* @return {@code this}
* @param changeControlCheckSchedule The schedule on which to check the calendar. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder changeControlCheckSchedule(final software.amazon.awscdk.services.events.Schedule changeControlCheckSchedule) {
this.props.changeControlCheckSchedule(changeControlCheckSchedule);
return this;
}
/**
* (experimental) The name of the pipeline.
*
* @return {@code this}
* @param pipelineName The name of the pipeline. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder pipelineName(final java.lang.String pipelineName) {
this.props.pipelineName(pipelineName);
return this;
}
/**
* (experimental) The terms in the alarm descriptions to search for.
*
* These if the alarms containing those search terms are in ALARM,
* the stage transition will be closed.
*
* @return {@code this}
* @param searchTerms The terms in the alarm descriptions to search for. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder searchTerms(final java.util.List searchTerms) {
this.props.searchTerms(searchTerms);
return this;
}
/**
* (experimental) The AWS CodeCommit repository to be used as the source stage.
*
* @return {@code this}
* @param sourceRepository The AWS CodeCommit repository to be used as the source stage. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder sourceRepository(final software.amazon.awscdk.services.codecommit.IRepository sourceRepository) {
this.props.sourceRepository(sourceRepository);
return this;
}
/**
* (experimental) The role used for running the pipeline.
*
* Default: - A new role is created when the pipeline is created.
*
* @return {@code this}
* @param pipelineRole The role used for running the pipeline. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder pipelineRole(final software.amazon.awscdk.services.iam.IRole pipelineRole) {
this.props.pipelineRole(pipelineRole);
return this;
}
/**
* @returns a newly built instance of {@link io.github.cdklabs.cdk.codepipeline.extensions.PipelineWithChangeControl}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public io.github.cdklabs.cdk.codepipeline.extensions.PipelineWithChangeControl build() {
return new io.github.cdklabs.cdk.codepipeline.extensions.PipelineWithChangeControl(
this.scope,
this.id,
this.props.build()
);
}
}
}