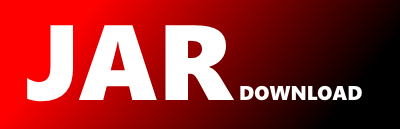
io.github.cdklabs.cdkpipelines.github.GitHubActionRole Maven / Gradle / Ivy
package io.github.cdklabs.cdkpipelines.github;
/**
* (experimental) Creates or references a GitHub OIDC provider and accompanying role that trusts the provider.
*
* This role can be used to authenticate against AWS instead of using long-lived AWS user credentials
* stored in GitHub secrets.
*
* You can do this manually in the console, or create a separate stack that uses this construct.
* You must cdk deploy
once (with your normal AWS credentials) to have this role created for you.
*
* You can then make note of the role arn in the stack output and send it into the Github Workflow app via
* the gitHubActionRoleArn
property. The role arn will be arn:<partition>:iam::<accountId>:role/GithubActionRole
.
*
* @see https://docs.github.com/en/actions/deployment/security-hardening-your-deployments/configuring-openid-connect-in-amazon-web-services
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.98.0 (build 00b106d)", date = "2024-06-02T18:14:52.312Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = io.github.cdklabs.cdkpipelines.github.$Module.class, fqn = "cdk-pipelines-github.GitHubActionRole")
public class GitHubActionRole extends software.constructs.Construct {
protected GitHubActionRole(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected GitHubActionRole(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public GitHubActionRole(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull io.github.cdklabs.cdkpipelines.github.GitHubActionRoleProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* (experimental) Reference an existing GitHub Actions provider.
*
* You do not need to pass in an arn because the arn for such
* a provider is always the same.
*
* @param scope This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IOpenIdConnectProvider existingGitHubActionsProvider(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(io.github.cdklabs.cdkpipelines.github.GitHubActionRole.class, "existingGitHubActionsProvider", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IOpenIdConnectProvider.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required") });
}
/**
* (experimental) The role that gets created.
*
* You should use the arn of this role as input to the gitHubActionRoleArn
* property in your GitHub Workflow app.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IRole getRole() {
return software.amazon.jsii.Kernel.get(this, "role", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
}
/**
* (experimental) A fluent builder for {@link io.github.cdklabs.cdkpipelines.github.GitHubActionRole}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private final io.github.cdklabs.cdkpipelines.github.GitHubActionRoleProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new io.github.cdklabs.cdkpipelines.github.GitHubActionRoleProps.Builder();
}
/**
* (experimental) The GitHub OpenId Connect Provider. Must have provider url https://token.actions.githubusercontent.com
. The audience must be sts:amazonaws.com
.
*
* Only one such provider can be defined per account, so if you already
* have a provider with the same url, a new provider cannot be created for you.
*
* Default: - a provider is created for you.
*
* @return {@code this}
* @param provider The GitHub OpenId Connect Provider. Must have provider url https://token.actions.githubusercontent.com
. The audience must be sts:amazonaws.com
. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder provider(final software.amazon.awscdk.services.iam.IOpenIdConnectProvider provider) {
this.props.provider(provider);
return this;
}
/**
* (experimental) A list of GitHub repositories you want to be able to access the IAM role.
*
* Each entry should be your GitHub username and repository passed in as a
* single string.
* An entry owner/repo
is equivalent to the subjectClaim repo:owner/repo:*
.
*
* For example, `['owner/repo1', 'owner/repo2'].
*
* @return {@code this}
* @param repos A list of GitHub repositories you want to be able to access the IAM role. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder repos(final java.util.List repos) {
this.props.repos(repos);
return this;
}
/**
* (experimental) The name of the Oidc role.
*
* Default: 'GitHubActionRole'
*
* @return {@code this}
* @param roleName The name of the Oidc role. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder roleName(final java.lang.String roleName) {
this.props.roleName(roleName);
return this;
}
/**
* (experimental) A list of subject claims allowed to access the IAM role.
*
* See https://docs.github.com/en/actions/deployment/security-hardening-your-deployments/about-security-hardening-with-openid-connect
* A subject claim can include *
and ?
wildcards according to the StringLike
* condition operator.
*
* For example, ['repo:owner/repo1:ref:refs/heads/branch1', 'repo:owner/repo1:environment:prod']
*
* @return {@code this}
* @param subjectClaims A list of subject claims allowed to access the IAM role. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder subjectClaims(final java.util.List subjectClaims) {
this.props.subjectClaims(subjectClaims);
return this;
}
/**
* (experimental) Thumbprints of GitHub's certificates.
*
* Every time GitHub rotates their certificates, this value will need to be updated.
*
* Default value is up-to-date to June 27, 2023 as per
* https://github.blog/changelog/2023-06-27-github-actions-update-on-oidc-integration-with-aws/
*
* Default: - Use built-in keys
*
* @return {@code this}
* @param thumbprints Thumbprints of GitHub's certificates. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder thumbprints(final java.util.List thumbprints) {
this.props.thumbprints(thumbprints);
return this;
}
/**
* @return a newly built instance of {@link io.github.cdklabs.cdkpipelines.github.GitHubActionRole}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public io.github.cdklabs.cdkpipelines.github.GitHubActionRole build() {
return new io.github.cdklabs.cdkpipelines.github.GitHubActionRole(
this.scope,
this.id,
this.props.build()
);
}
}
}