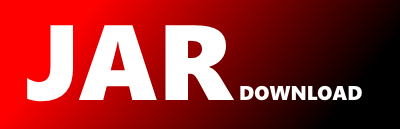
edu.cmu.tetradapp.editor.GeneralizedSemImParamsEditor Maven / Gradle / Ivy
///////////////////////////////////////////////////////////////////////////////
// For information as to what this class does, see the Javadoc, below. //
// Copyright (C) 1998, 1999, 2000, 2001, 2002, 2003, 2004, 2005, 2006, //
// 2007, 2008, 2009, 2010, 2014, 2015, 2022 by Peter Spirtes, Richard //
// Scheines, Joseph Ramsey, and Clark Glymour. //
// //
// This program is free software; you can redistribute it and/or modify //
// it under the terms of the GNU General Public License as published by //
// the Free Software Foundation; either version 2 of the License, or //
// (at your option) any later version. //
// //
// This program is distributed in the hope that it will be useful, //
// but WITHOUT ANY WARRANTY; without even the implied warranty of //
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the //
// GNU General Public License for more details. //
// //
// You should have received a copy of the GNU General Public License //
// along with this program; if not, write to the Free Software //
// Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA //
///////////////////////////////////////////////////////////////////////////////
package edu.cmu.tetradapp.editor;
import edu.cmu.tetrad.sem.GeneralizedSemIm;
import edu.cmu.tetrad.sem.GeneralizedSemPm;
import edu.cmu.tetrad.util.NumberFormatUtil;
import edu.cmu.tetradapp.util.DoubleTextField;
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import java.awt.*;
import java.text.NumberFormat;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
/**
* Edits the parameters of the SemIm using a graph workbench.
*/
class GeneralizedSemImParamsEditor extends JPanel {
/**
* Font size for parameter values in the graph.
*/
private static final Font SMALL_FONT = new Font("Dialog", Font.PLAIN, 10);
/**
* The SemPm being edited.
*/
private final GeneralizedSemIm semIm;
/**
* The PM being edited.
*/
private final GeneralizedSemPm semPm;
/**
* Constructs a SemPm graphical editor for the given SemIm.
*/
public GeneralizedSemImParamsEditor(GeneralizedSemIm semIm, Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy