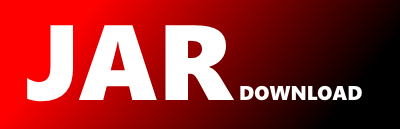
edu.cmu.tetradapp.model.VcpcRunner Maven / Gradle / Ivy
///////////////////////////////////////////////////////////////////////////////
// For information as to what this class does, see the Javadoc, below. //
// Copyright (C) 1998, 1999, 2000, 2001, 2002, 2003, 2004, 2005, 2006, //
// 2007, 2008, 2009, 2010, 2014, 2015, 2022 by Peter Spirtes, Richard //
// Scheines, Joseph Ramsey, and Clark Glymour. //
// //
// This program is free software; you can redistribute it and/or modify //
// it under the terms of the GNU General Public License as published by //
// the Free Software Foundation; either version 2 of the License, or //
// (at your option) any later version. //
// //
// This program is distributed in the hope that it will be useful, //
// but WITHOUT ANY WARRANTY; without even the implied warranty of //
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the //
// GNU General Public License for more details. //
// //
// You should have received a copy of the GNU General Public License //
// along with this program; if not, write to the Free Software //
// Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA //
///////////////////////////////////////////////////////////////////////////////
package edu.cmu.tetradapp.model;
import edu.cmu.tetrad.data.Knowledge;
import edu.cmu.tetrad.graph.*;
import edu.cmu.tetrad.search.IndependenceTest;
import edu.cmu.tetrad.search.test.MsepTest;
import edu.cmu.tetrad.search.utils.GraphSearchUtils;
import edu.cmu.tetrad.search.utils.MeekRules;
import edu.cmu.tetrad.search.work_in_progress.VcPc;
import edu.cmu.tetrad.util.Parameters;
import edu.cmu.tetrad.util.TetradSerializableUtils;
import edu.cmu.tetradapp.util.IndTestType;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
/**
* Extends AbstractAlgorithmRunner to produce a wrapper for the PC algorithm.
*
* @author josephramsey
*/
public class VcpcRunner extends AbstractAlgorithmRunner
implements IndTestProducer {
private static final long serialVersionUID = 23L;
private Graph dag;
private IndependenceFactsModel independenceFactsModel;
private Graph trueGraph;
// private Vcpc vcpc = null;
private Set vcpcAdjacent;
private Set vcpcApparent;
private Set vcpcDefinite;
//============================CONSTRUCTORS============================//
/**
* Constructs a wrapper for the given DataWrapper. The DataWrapper must contain a DataSet that is either a DataSet
* or a DataSet or a DataList containing either a DataSet or a DataSet as its selected model.
*/
public VcpcRunner(DataWrapper dataWrapper, Parameters params) {
super(dataWrapper, params, null);
}
public VcpcRunner(DataWrapper dataWrapper, Parameters params, KnowledgeBoxModel knowledgeBoxModel) {
super(dataWrapper, params, knowledgeBoxModel);
}
public VcpcRunner(IndependenceFactsModel indModel, GraphWrapper graphWrapper, Parameters params) {
super(graphWrapper.getGraph(), params);
this.dag = graphWrapper.getGraph();
this.independenceFactsModel = indModel;
}
/**
* Constucts a wrapper for the given /** Constucts a wrapper for the given EdgeListGraph.
*/
public VcpcRunner(Graph graph, Parameters params) {
super(graph, params);
}
/**
* Constucts a wrapper for the given EdgeListGraph.
*/
public VcpcRunner(Graph graph, Parameters params, KnowledgeBoxModel knowledgeBoxModel) {
super(graph, params, knowledgeBoxModel);
}
/**
* Constucts a wrapper for the given EdgeListGraph.
*/
public VcpcRunner(GraphWrapper graphWrapper, Parameters params) {
super(graphWrapper.getGraph(), params);
}
/**
* Constucts a wrapper for the given EdgeListGraph.
*/
public VcpcRunner(GraphWrapper graphWrapper, Parameters params, KnowledgeBoxModel knowledgeBoxModel) {
super(graphWrapper.getGraph(), params, knowledgeBoxModel);
}
/**
* Constucts a wrapper for the given EdgeListGraph.
*/
public VcpcRunner(GraphSource graphWrapper, Parameters params, KnowledgeBoxModel knowledgeBoxModel) {
super(graphWrapper.getGraph(), params, knowledgeBoxModel);
}
public VcpcRunner(GraphSource graphWrapper, Parameters params, IndependenceFactsModel model) {
super(graphWrapper.getGraph(), params);
this.independenceFactsModel = model;
}
/**
* Constucts a wrapper for the given EdgeListGraph.
*/
public VcpcRunner(GraphSource graphWrapper, Parameters params) {
super(graphWrapper.getGraph(), params);
}
public VcpcRunner(DagWrapper dagWrapper, Parameters params) {
super(dagWrapper.getDag(), params);
}
public VcpcRunner(DagWrapper dagWrapper, Parameters params, KnowledgeBoxModel knowledgeBoxModel) {
super(dagWrapper.getDag(), params, knowledgeBoxModel);
}
public VcpcRunner(SemGraphWrapper dagWrapper, Parameters params) {
super(dagWrapper.getGraph(), params);
}
public VcpcRunner(SemGraphWrapper dagWrapper, Parameters params, KnowledgeBoxModel knowledgeBoxModel) {
super(dagWrapper.getGraph(), params, knowledgeBoxModel);
}
public VcpcRunner(DataWrapper dataWrapper, GraphWrapper graphWrapper, Parameters params) {
super(dataWrapper, params, null);
this.trueGraph = graphWrapper.getGraph();
}
public VcpcRunner(DataWrapper dataWrapper, GraphWrapper graphWrapper, Parameters params, KnowledgeBoxModel knowledgeBoxModel) {
super(dataWrapper, params, knowledgeBoxModel);
this.trueGraph = graphWrapper.getGraph();
}
public VcpcRunner(IndependenceFactsModel model, Parameters params) {
super(model, params, null);
}
public VcpcRunner(IndependenceFactsModel model, Parameters params, KnowledgeBoxModel knowledgeBoxModel) {
super(model, params, knowledgeBoxModel);
}
/**
* Generates a simple exemplar of this class to test serialization.
*
* @see TetradSerializableUtils
*/
public static VcpcRunner serializableInstance() {
return new VcpcRunner(Dag.serializableInstance(), new Parameters());
}
//===================PUBLIC METHODS OVERRIDING ABSTRACT================//
public void execute() {
Knowledge knowledge = (Knowledge) getParams().get("knowledge", new Knowledge());
VcPc vcpc = new VcPc(getIndependenceTest());
vcpc.setKnowledge(knowledge);
vcpc.setMeekPreventCycles(this.isMeekPreventCycles());
vcpc.setDepth(getParams().getInt("depth", -1));
if (this.independenceFactsModel != null) {
vcpc.setFacts(this.independenceFactsModel.getFacts());
}
Graph graph = vcpc.search();
if (getSourceGraph() != null) {
LayoutUtil.arrangeBySourceGraph(graph, getSourceGraph());
} else if (knowledge.isDefaultToKnowledgeLayout()) {
GraphSearchUtils.arrangeByKnowledgeTiers(graph, knowledge);
} else {
LayoutUtil.defaultLayout(graph);
}
setResultGraph(graph);
setVcpcFields(vcpc);
}
public IndependenceTest getIndependenceTest() {
if (this.dag != null) {
return new MsepTest(this.dag);
}
Object dataModel = getDataModel();
if (dataModel == null) {
dataModel = getSourceGraph();
}
IndTestType testType = (IndTestType) (getParams()).get("indTestType", IndTestType.FISHER_Z);
return new IndTestChooser().getTest(dataModel, getParams(), testType);
}
public Graph getGraph() {
return getResultGraph();
}
public IndependenceFactsModel getIndependenceFactsModel() {
return this.independenceFactsModel;
}
/**
* @return the names of the triple classifications. Coordinates with
*/
public List getTriplesClassificationTypes() {
List names = new ArrayList<>();
names.add("Ambiguous Triples");
return names;
}
/**
* @return the list of triples corresponding to getTripleClassificationNames
.
*/
public List> getTriplesLists(Node node) {
List> triplesList = new ArrayList<>();
Graph graph = getGraph();
triplesList.add(GraphUtils.getAmbiguousTriplesFromGraph(node, graph));
return triplesList;
}
public Set getAdj() {
return new HashSet<>(this.vcpcAdjacent);
}
public Set getAppNon() {
return new HashSet<>(this.vcpcApparent);
}
public Set getDefNon() {
return new HashSet<>(this.vcpcDefinite);
}
public boolean supportsKnowledge() {
return true;
}
public MeekRules getMeekRules() {
MeekRules meekRules = new MeekRules();
meekRules.setMeekPreventCycles(this.isMeekPreventCycles());
meekRules.setKnowledge((Knowledge) getParams().get("knowledge", new Knowledge()));
return meekRules;
}
@Override
public String getAlgorithmName() {
return "VCPC";
}
//========================== Private Methods ===============================//
private boolean isMeekPreventCycles() {
Parameters params = getParams();
if (params instanceof Parameters) {
return params.getBoolean("MeekPreventCycles", false);
}
return false;
}
private void setVcpcFields(VcPc vcpc) {
this.vcpcAdjacent = vcpc.getAdjacencies();
this.vcpcApparent = vcpc.getApparentNonadjacencies();
this.vcpcDefinite = vcpc.getDefiniteNonadjacencies();
List vcpcNodes = getGraph().getNodes();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy