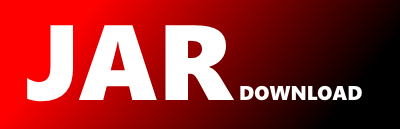
edu.cmu.tetradapp.editor.AbstractSearchEditor Maven / Gradle / Ivy
///////////////////////////////////////////////////////////////////////////////
// For information as to what this class does, see the Javadoc, below. //
// Copyright (C) 1998, 1999, 2000, 2001, 2002, 2003, 2004, 2005, 2006, //
// 2007, 2008, 2009, 2010, 2014, 2015, 2022 by Peter Spirtes, Richard //
// Scheines, Joseph Ramsey, and Clark Glymour. //
// //
// This program is free software; you can redistribute it and/or modify //
// it under the terms of the GNU General Public License as published by //
// the Free Software Foundation; either version 2 of the License, or //
// (at your option) any later version. //
// //
// This program is distributed in the hope that it will be useful, //
// but WITHOUT ANY WARRANTY; without even the implied warranty of //
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the //
// GNU General Public License for more details. //
// //
// You should have received a copy of the GNU General Public License //
// along with this program; if not, write to the Free Software //
// Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA //
///////////////////////////////////////////////////////////////////////////////
package edu.cmu.tetradapp.editor;
import edu.cmu.tetrad.data.DataModel;
import edu.cmu.tetrad.data.Knowledge;
import edu.cmu.tetrad.graph.EdgeListGraph;
import edu.cmu.tetrad.graph.Graph;
import edu.cmu.tetrad.graph.LayoutUtil;
import edu.cmu.tetrad.util.JOptionUtils;
import edu.cmu.tetrad.util.Parameters;
import edu.cmu.tetrad.util.TetradLogger;
import edu.cmu.tetrad.util.TetradSerializable;
import edu.cmu.tetradapp.model.AlgorithmRunner;
import edu.cmu.tetradapp.util.GraphHistory;
import edu.cmu.tetradapp.util.IndTestType;
import edu.cmu.tetradapp.util.WatchedProcess;
import edu.cmu.tetradapp.workbench.DisplayEdge;
import edu.cmu.tetradapp.workbench.DisplayNode;
import edu.cmu.tetradapp.workbench.GraphWorkbench;
import javax.swing.*;
import java.awt.*;
import java.awt.event.InputEvent;
import java.awt.event.KeyEvent;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.io.CharArrayWriter;
import java.io.IOException;
import java.io.PrintWriter;
import java.rmi.MarshalledObject;
import java.util.ArrayList;
/**
* Abstract base class for a number of search editors. The advantage of extending this class, in case you were
* wondering, is that it will handle threading for you, provide a stop button for algorithm, and do logging. The execute
* button used must be getExecuteButton(), or else logging won't work.
*
* @author josephramsey
*/
public abstract class AbstractSearchEditor extends JPanel implements GraphEditable, IndTestTypeSetter {
/**
* The algorithm wrapper being viewed.
*/
private final AlgorithmRunner algorithmRunner;
/**
* The button one clicks to executeButton the algorithm.
*/
private final JButton executeButton = new JButton();
/**
* The label for the result graph workbench.
*/
private final String resultLabel;
/**
* History of graph edits.
*/
private final GraphHistory graphHistory = new GraphHistory();
/**
* True if the warning message that previously defined knowledge is being used has already been shown and doesn't
* need to be shown again.
*/
boolean knowledgeMessageShown;
/**
* The workbench displaying the result workbench.
*/
private GraphWorkbench workbench;
/**
* The scrollpange for the result workbench.
*/
private JScrollPane workbenchScroll;
//============================CONSTRUCTOR===========================//
AbstractSearchEditor(AlgorithmRunner algorithmRunner, String resultLabel) {
if (algorithmRunner == null) {
throw new NullPointerException();
}
if (resultLabel == null) {
throw new NullPointerException();
}
this.algorithmRunner = algorithmRunner;
this.resultLabel = resultLabel;
setup(resultLabel);
}
/**
* Sets the name of this editor.
*/
public final void setName(String name) {
String oldName = getName();
super.setName(name);
firePropertyChange("name", oldName, getName());
}
//========================== Public Methods required by GraphEditable ======//
/**
* @return the work bench of null if there isn't one.
*/
public GraphWorkbench getWorkbench() {
return this.workbench;
}
void setWorkbench(GraphWorkbench graphWorkbench) {
this.workbench = graphWorkbench;
}
/**
* @return the graph.
*/
public Graph getGraph() {
if (this.workbench != null) {
return this.workbench.getGraph();
}
return new EdgeListGraph();
}
/**
* Not supported.
*/
public void setGraph(Graph g) {
this.workbench.setGraph(g);
throw new UnsupportedOperationException("Cannot set the graph on a search editor.");
}
/**
* Returns a list of all the SessionNodeWrappers (TetradNodes) and SessionNodeEdges that are model components for
* the respective SessionNodes and SessionEdges selected in the workbench. Note that the workbench, not the
* SessionEditorNodes themselves, keeps track of the selection.
*
* @return the set of selected model nodes.
*/
public java.util.List getSelectedModelComponents() {
java.util.List selectedComponents =
this.workbench.getSelectedComponents();
java.util.List selectedModelComponents =
new ArrayList<>();
for (Component comp : selectedComponents) {
if (comp instanceof DisplayNode) {
selectedModelComponents.add(
((DisplayNode) comp).getModelNode());
} else if (comp instanceof DisplayEdge) {
selectedModelComponents.add(
((DisplayEdge) comp).getModelEdge());
}
}
return selectedModelComponents;
}
//===========================PROTECTED METHODS==========================//
/**
* Not supported.
*/
public void pasteSubsession(java.util.List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy