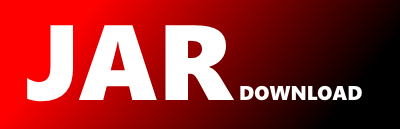
io.github.coffeelibs.maven.jextract.SourcesMojo Maven / Gradle / Ivy
package io.github.coffeelibs.maven.jextract;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import java.io.File;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
@SuppressWarnings({"unused", "MismatchedReadAndWriteOfArray"})
@Mojo(name = "sources", defaultPhase = LifecyclePhase.GENERATE_SOURCES, threadSafe = true, requiresOnline = true)
public class SourcesMojo extends AbstractMojo {
@Parameter(defaultValue = "${project}")
private MavenProject project;
/**
* Path to the jextract
binary.
*/
@Parameter(property = "jextract.executable", required = true)
private String executable;
/**
*
* - --include-macro
* - name of constant macro to include
*
*/
@Parameter(property = "jextract.headerFile", required = true)
private String headerFile;
/**
*
* - --target-package
* - target package for specified header files
*
*/
@Parameter(property = "jextract.targetPackage", required = true)
private String targetPackage;
/**
*
* - --header-class-name
* - name of the header class
*
*/
@Parameter(property = "jextract.headerClassName", required = false)
private String headerClassName;
/**
*
* - -I
* - specify include files path
*
*/
@Parameter(property = "jextract.headerSearchPaths", required = false)
private String[] headerSearchPaths;
/**
*
* - -D
* - C preprocessor macro
*
*/
@Parameter(property = "jextract.cPreprocessorMacros", required = false)
private String[] cPreprocessorMacros;
/**
*
* - --include-function
* - name of function to include
*
*/
@Parameter(property = "jextract.includeFunctions", required = false)
private String[] includeFunctions;
/**
*
* - --include-constant
* - name of macro or enum constant to include
*
*/
@Parameter(property = "jextract.includeConstants", required = false)
private String[] includeConstants;
/**
*
* - --include-struct
* - name of struct definition to include
*
*/
@Parameter(property = "jextract.includeStructs", required = false)
private String[] includeStructs;
/**
*
* - --include-typedef
* - name of type definition to include
*
*/
@Parameter(property = "jextract.includeTypedefs", required = false)
private String[] includeTypedefs;
/**
*
* - --include-union
* - name of union definition to include
*
*/
@Parameter(property = "jextract.includeUnions", required = false)
private String[] includeUnions;
/**
*
* - --include-var
* - name of global variable to include
*
*/
@Parameter(property = "jextract.includeVars", required = false)
private String[] includeVars;
/**
*
* - --library
* - path to shared libraries to load
*
*/
@Parameter(property = "jextract.libraries", required = false)
private String[] libraries;
/**
*
* - --output
* - specify the directory to place generated files
*
*/
@Parameter(property = "jextract.outputDirectory", defaultValue = "${project.build.directory}/generated-sources/jextract", required = true)
private File outputDirectory;
/**
* working directory, which might contain compile_flags.txt
to specify additional clang compiler options.
*/
@Parameter(property = "jextract.workingDirectory", defaultValue = "${project.basedir}", required = true)
private File workingDirectory;
public void execute() throws MojoFailureException {
try {
getLog().debug("Create dir " + outputDirectory);
Files.createDirectories(outputDirectory.toPath());
} catch (IOException e) {
throw new MojoFailureException("Failed to create dir " + outputDirectory.getAbsolutePath(), e);
}
List args = new ArrayList<>();
args.add(executable);
if (headerClassName != null) {
args.add("--header-class-name");
args.add(headerClassName);
}
args.add("--output");
args.add(outputDirectory.getAbsolutePath());
args.add("--target-package");
args.add(targetPackage);
Arrays.stream(libraries).forEach(str -> {
args.add("--library");
args.add(str);
});
Arrays.stream(headerSearchPaths).forEach(str -> {
args.add("-I");
args.add(str);
});
Arrays.stream(cPreprocessorMacros).forEach(str -> {
args.add("-D");
args.add(str);
});
Arrays.stream(includeFunctions).forEach(str -> {
args.add("--include-function");
args.add(str);
});
Arrays.stream(includeConstants).forEach(str -> {
args.add("--include-constant");
args.add(str);
});
Arrays.stream(includeStructs).forEach(str -> {
args.add("--include-struct");
args.add(str);
});
Arrays.stream(includeTypedefs).forEach(str -> {
args.add("--include-typedef");
args.add(str);
});
Arrays.stream(includeUnions).forEach(str -> {
args.add("--include-union");
args.add(str);
});
Arrays.stream(includeVars).forEach(str -> {
args.add("--include-var");
args.add(str);
});
args.add(headerFile);
getLog().debug("Running: " + String.join(" ", args));
ProcessBuilder command = new ProcessBuilder(args);
command.directory(workingDirectory);
try (var stdout = new LoggingOutputStream(getLog()::info, StandardCharsets.UTF_8);
var stderr = new LoggingOutputStream(getLog()::warn, StandardCharsets.UTF_8)) {
Process process = command.start();
process.getInputStream().transferTo(stdout);
process.getErrorStream().transferTo(stderr);
int result = process.waitFor();
if (result != 0) {
throw new MojoFailureException("jextract returned error code " + result);
}
} catch (IOException e) {
throw new MojoFailureException("Invoking jextract failed", e);
} catch (InterruptedException e) {
throw new MojoFailureException("Interrupted while waiting for jextract", e);
}
project.addCompileSourceRoot(outputDirectory.toString());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy