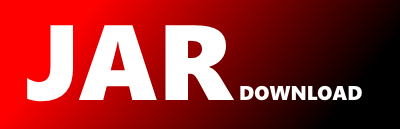
io.github.contractautomata.catlib.automaton.label.action.AddressedRequestAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of catlib Show documentation
Show all versions of catlib Show documentation
Library for specifying and verifying contract automata.
The newest version!
package io.github.contractautomata.catlib.automaton.label.action;
import java.util.Objects;
/**
* Class implementing an addressed request action.
* It extends request action and implements addressed action.
*
* @author Davide Basile
*/
public class AddressedRequestAction extends RequestAction implements AddressedAction{
/**
* the address of this action
*/
private final Address address;
/**
* Constructor for an addressed request action
* @param label the label of this action
* @param address the address of this action
*/
public AddressedRequestAction(String label, Address address) {
super(label);
Objects.requireNonNull(address);
this.address=address;
}
/**
* Getter of the address of this action
* @return the address of this action
*/
@Override
public Address getAddress() {
return address;
}
/**
* Redefinition of the match of an action.
* Returns true if arg is an addressed action, the corresponding
* addresses are matching as well as their super classes.
* For example, an addressed offer action matches an addressed request action
* if both addresses are matching and the offer is matching the request.
*
* @param arg the other action to match
* @return true if the two actions are matching.
*/
@Override
public boolean match(Action arg) {
return (arg instanceof AddressedAction) && address.match(((AddressedAction)arg).getAddress()) && super.match(arg);
}
/**
* Print a String representing this object
* @return a String representing this object
*/
@Override
public String toString() {
return address.toString()+super.toString();
}
/**
* Overrides the method of the object class
* @param o the other object to compare to
* @return true if the two objects are equal
*/
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
AddressedRequestAction that = (AddressedRequestAction) o;
return Objects.equals(address, that.address);
}
/**
* Overrides the method of the object class
* @return the hashcode of this object
*/
@Override
public int hashCode() {
return Objects.hash(super.hashCode(), address);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy