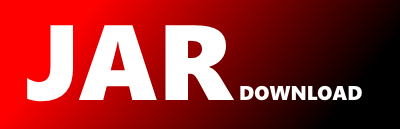
commonMain.com.copperleaf.ballast.navigation.internal.internalUtils.kt Maven / Gradle / Ivy
package com.copperleaf.ballast.navigation.internal
import com.copperleaf.ballast.navigation.routing.Destination
import com.copperleaf.ballast.navigation.routing.PathSegment
import com.copperleaf.ballast.navigation.routing.QueryParameter
import com.copperleaf.ballast.navigation.routing.Route
// Directions
// ---------------------------------------------------------------------------------------------------------------------
internal fun Route.directionsInternal(
parameters: Destination.Parameters,
): String {
val formattedPath = directionsForPath(parameters.pathParameters)
val formattedQueryString = directionsForQuery(parameters.queryParameters)
if (formattedPath.isFailure || formattedQueryString.isFailure) {
error(directionsErrorMessage(parameters, formattedPath, formattedQueryString))
}
return "${formattedPath.getOrThrow()}${formattedQueryString.getOrThrow()}"
}
internal fun Route.directionsForPath(
pathParameters: Map>,
): Result {
val pathDirections: Pair
© 2015 - 2025 Weber Informatics LLC | Privacy Policy