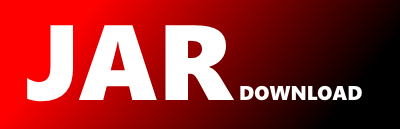
commonMain.com.copperleaf.ballast.test.internal.vm.TestInterceptor.kt Maven / Gradle / Ivy
package com.copperleaf.ballast.test.internal.vm
import com.copperleaf.ballast.BallastInterceptor
import com.copperleaf.ballast.BallastLogger
import com.copperleaf.ballast.BallastNotification
import com.copperleaf.ballast.SideJobScope
import com.copperleaf.ballast.test.TestResults
/**
* An internal class used to keep the test framework
*/
internal class TestInterceptor :
BallastInterceptor, Events, State> {
private val acceptedInputs = mutableListOf()
private val rejectedInputs = mutableListOf()
private val droppedInputs = mutableListOf()
private val successfulInputs = mutableListOf()
private val cancelledInputs = mutableListOf()
private val inputHandlerErrors = mutableListOf>()
private val events = mutableListOf()
private val successfulEvents = mutableListOf()
private val eventHandlerErrors = mutableListOf>()
private val states = mutableListOf()
private val sideJobs = mutableListOf>()
private val completedSideJobs = mutableListOf()
private val sideJobErrors = mutableListOf>()
private val unhandledErrors = mutableListOf()
private inline fun TestViewModel.Inputs.unwrap(block: (Inputs) -> Unit) {
when (this) {
is TestViewModel.Inputs.AwaitInput -> {
block(this.normalInput)
}
is TestViewModel.Inputs.ProcessInput -> {
block(this.normalInput)
}
is TestViewModel.Inputs.TestCompleted -> {
}
}
}
override suspend fun onNotify(
logger: BallastLogger,
notification: BallastNotification, Events, State>
) {
when (notification) {
is BallastNotification.InputAccepted -> {
notification.input.unwrap { acceptedInputs += it }
}
is BallastNotification.InputRejected -> {
notification.input.unwrap { rejectedInputs += it }
}
is BallastNotification.InputDropped -> {
notification.input.unwrap { droppedInputs += it }
}
is BallastNotification.InputHandledSuccessfully -> {
notification.input.unwrap { successfulInputs += it }
}
is BallastNotification.InputCancelled -> {
notification.input.unwrap { cancelledInputs += it }
}
is BallastNotification.InputHandlerError -> {
notification.input.unwrap { inputHandlerErrors += it to notification.throwable }
}
is BallastNotification.EventEmitted -> {
events += notification.event
}
is BallastNotification.EventHandledSuccessfully -> {
successfulEvents += notification.event
}
is BallastNotification.EventHandlerError -> {
eventHandlerErrors += notification.event to notification.throwable
}
is BallastNotification.StateChanged -> {
states += notification.state
}
is BallastNotification.SideJobStarted -> {
sideJobs += notification.key to notification.restartState
}
is BallastNotification.SideJobCompleted -> {
completedSideJobs += notification.key
}
is BallastNotification.SideJobError -> {
sideJobErrors += notification.key to notification.throwable
}
is BallastNotification.UnhandledError -> {
unhandledErrors += notification.throwable
}
else -> {}
}
}
internal suspend fun getResults(): TestResults {
// wait for the final notification to be received
return TestResults(
acceptedInputs = acceptedInputs.toList(),
rejectedInputs = rejectedInputs.toList(),
droppedInputs = droppedInputs.toList(),
successfulInputs = successfulInputs.toList(),
cancelledInputs = cancelledInputs.toList(),
inputHandlerErrors = inputHandlerErrors.toList(),
events = events.toList(),
eventHandlerErrors = eventHandlerErrors.toList(),
states = states.toList(),
sideJobs = sideJobs.toList(),
sideJobErrors = sideJobErrors.toList(),
unhandledErrors = unhandledErrors.toList(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy