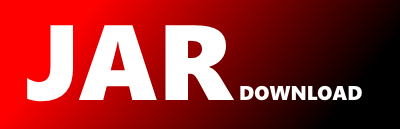
com.brewchain.sdk.model.Model Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cwvj Show documentation
Show all versions of cwvj Show documentation
Java sdk for Dapps interact with cwv blockchain node
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: Model.proto
package com.brewchain.sdk.model;
public final class Model {
private Model() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code com.brewchain.sdk.model.SendOwnerTokenOpCode}
*/
public enum SendOwnerTokenOpCode
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*发行
*
*
* PUBLIC = 0;
*/
PUBLIC(0),
/**
*
*燃烧
*
*
* BURN = 1;
*/
BURN(1),
/**
*
*增发
*
*
* MINT = 2;
*/
MINT(2),
UNRECOGNIZED(-1),
;
/**
*
*发行
*
*
* PUBLIC = 0;
*/
public static final int PUBLIC_VALUE = 0;
/**
*
*燃烧
*
*
* BURN = 1;
*/
public static final int BURN_VALUE = 1;
/**
*
*增发
*
*
* MINT = 2;
*/
public static final int MINT_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SendOwnerTokenOpCode valueOf(int value) {
return forNumber(value);
}
public static SendOwnerTokenOpCode forNumber(int value) {
switch (value) {
case 0: return PUBLIC;
case 1: return BURN;
case 2: return MINT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
SendOwnerTokenOpCode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public SendOwnerTokenOpCode findValueByNumber(int number) {
return SendOwnerTokenOpCode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.brewchain.sdk.model.Model.getDescriptor().getEnumTypes().get(0);
}
private static final SendOwnerTokenOpCode[] VALUES = values();
public static SendOwnerTokenOpCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private SendOwnerTokenOpCode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:com.brewchain.sdk.model.SendOwnerTokenOpCode)
}
public interface SendTransactionOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.brewchain.sdk.model.SendTransaction)
com.google.protobuf.MessageOrBuilder {
/**
* string address = 1;
*/
java.lang.String getAddress();
/**
* string address = 1;
*/
com.google.protobuf.ByteString
getAddressBytes();
/**
* int32 nonce = 2;
*/
int getNonce();
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
java.util.List
getOutputsList();
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
com.brewchain.sdk.model.Model.SendTransactionOutput getOutputs(int index);
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
int getOutputsCount();
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
java.util.List extends com.brewchain.sdk.model.Model.SendTransactionOutputOrBuilder>
getOutputsOrBuilderList();
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
com.brewchain.sdk.model.Model.SendTransactionOutputOrBuilder getOutputsOrBuilder(
int index);
/**
*
*手续费高位
*
*
* int64 feeHi = 4;
*/
long getFeeHi();
/**
*
*手续费低位;
*
*
* int64 feeLow = 5;
*/
long getFeeLow();
/**
*
*内置指令交易[0=普通交易,1=多重签名交易,2=RC20交易,3=RC721交易,4=CVM合约调用,5=JSVM合约调用,6=evfs交易,7=链委员会,8=链管理员组
*
*
* .com.brewchain.sdk.model.SendTransaction.CodeType innerCodeType = 6;
*/
int getInnerCodeTypeValue();
/**
*
*内置指令交易[0=普通交易,1=多重签名交易,2=RC20交易,3=RC721交易,4=CVM合约调用,5=JSVM合约调用,6=evfs交易,7=链委员会,8=链管理员组
*
*
* .com.brewchain.sdk.model.SendTransaction.CodeType innerCodeType = 6;
*/
com.brewchain.sdk.model.Model.SendTransaction.CodeType getInnerCodeType();
/**
*
*指令数据
*
*
* bytes codeData = 7;
*/
com.google.protobuf.ByteString getCodeData();
/**
* string exData = 8;
*/
java.lang.String getExData();
/**
* string exData = 8;
*/
com.google.protobuf.ByteString
getExDataBytes();
/**
* int64 timestamp = 9;
*/
long getTimestamp();
/**
* string privateKey = 10;
*/
java.lang.String getPrivateKey();
/**
* string privateKey = 10;
*/
com.google.protobuf.ByteString
getPrivateKeyBytes();
}
/**
* Protobuf type {@code com.brewchain.sdk.model.SendTransaction}
*/
public static final class SendTransaction extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.brewchain.sdk.model.SendTransaction)
SendTransactionOrBuilder {
private static final long serialVersionUID = 0L;
// Use SendTransaction.newBuilder() to construct.
private SendTransaction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SendTransaction() {
address_ = "";
nonce_ = 0;
outputs_ = java.util.Collections.emptyList();
feeHi_ = 0L;
feeLow_ = 0L;
innerCodeType_ = 0;
codeData_ = com.google.protobuf.ByteString.EMPTY;
exData_ = "";
timestamp_ = 0L;
privateKey_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SendTransaction(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
address_ = s;
break;
}
case 16: {
nonce_ = input.readInt32();
break;
}
case 32: {
feeHi_ = input.readInt64();
break;
}
case 40: {
feeLow_ = input.readInt64();
break;
}
case 48: {
int rawValue = input.readEnum();
innerCodeType_ = rawValue;
break;
}
case 58: {
codeData_ = input.readBytes();
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
exData_ = s;
break;
}
case 72: {
timestamp_ = input.readInt64();
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
privateKey_ = s;
break;
}
case 290: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
outputs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
outputs_.add(
input.readMessage(com.brewchain.sdk.model.Model.SendTransactionOutput.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
outputs_ = java.util.Collections.unmodifiableList(outputs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.brewchain.sdk.model.Model.internal_static_com_brewchain_sdk_model_SendTransaction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.brewchain.sdk.model.Model.internal_static_com_brewchain_sdk_model_SendTransaction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.brewchain.sdk.model.Model.SendTransaction.class, com.brewchain.sdk.model.Model.SendTransaction.Builder.class);
}
/**
* Protobuf enum {@code com.brewchain.sdk.model.SendTransaction.CodeType}
*/
public enum CodeType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NORMAL = 0;
*/
NORMAL(0),
/**
* MULI_SIGN = 1;
*/
MULI_SIGN(1),
/**
* RC20_CONTRACT = 2;
*/
RC20_CONTRACT(2),
/**
* RC721_CONTRACT = 3;
*/
RC721_CONTRACT(3),
/**
* CVM_CONTRACT = 4;
*/
CVM_CONTRACT(4),
/**
* JSVM_CONTRACT = 5;
*/
JSVM_CONTRACT(5),
UNRECOGNIZED(-1),
;
/**
* NORMAL = 0;
*/
public static final int NORMAL_VALUE = 0;
/**
* MULI_SIGN = 1;
*/
public static final int MULI_SIGN_VALUE = 1;
/**
* RC20_CONTRACT = 2;
*/
public static final int RC20_CONTRACT_VALUE = 2;
/**
* RC721_CONTRACT = 3;
*/
public static final int RC721_CONTRACT_VALUE = 3;
/**
* CVM_CONTRACT = 4;
*/
public static final int CVM_CONTRACT_VALUE = 4;
/**
* JSVM_CONTRACT = 5;
*/
public static final int JSVM_CONTRACT_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CodeType valueOf(int value) {
return forNumber(value);
}
public static CodeType forNumber(int value) {
switch (value) {
case 0: return NORMAL;
case 1: return MULI_SIGN;
case 2: return RC20_CONTRACT;
case 3: return RC721_CONTRACT;
case 4: return CVM_CONTRACT;
case 5: return JSVM_CONTRACT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
CodeType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public CodeType findValueByNumber(int number) {
return CodeType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.brewchain.sdk.model.Model.SendTransaction.getDescriptor().getEnumTypes().get(0);
}
private static final CodeType[] VALUES = values();
public static CodeType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private CodeType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:com.brewchain.sdk.model.SendTransaction.CodeType)
}
private int bitField0_;
public static final int ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object address_;
/**
* string address = 1;
*/
public java.lang.String getAddress() {
java.lang.Object ref = address_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
address_ = s;
return s;
}
}
/**
* string address = 1;
*/
public com.google.protobuf.ByteString
getAddressBytes() {
java.lang.Object ref = address_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
address_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NONCE_FIELD_NUMBER = 2;
private int nonce_;
/**
* int32 nonce = 2;
*/
public int getNonce() {
return nonce_;
}
public static final int OUTPUTS_FIELD_NUMBER = 36;
private java.util.List outputs_;
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public java.util.List getOutputsList() {
return outputs_;
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public java.util.List extends com.brewchain.sdk.model.Model.SendTransactionOutputOrBuilder>
getOutputsOrBuilderList() {
return outputs_;
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public int getOutputsCount() {
return outputs_.size();
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public com.brewchain.sdk.model.Model.SendTransactionOutput getOutputs(int index) {
return outputs_.get(index);
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public com.brewchain.sdk.model.Model.SendTransactionOutputOrBuilder getOutputsOrBuilder(
int index) {
return outputs_.get(index);
}
public static final int FEEHI_FIELD_NUMBER = 4;
private long feeHi_;
/**
*
*手续费高位
*
*
* int64 feeHi = 4;
*/
public long getFeeHi() {
return feeHi_;
}
public static final int FEELOW_FIELD_NUMBER = 5;
private long feeLow_;
/**
*
*手续费低位;
*
*
* int64 feeLow = 5;
*/
public long getFeeLow() {
return feeLow_;
}
public static final int INNERCODETYPE_FIELD_NUMBER = 6;
private int innerCodeType_;
/**
*
*内置指令交易[0=普通交易,1=多重签名交易,2=RC20交易,3=RC721交易,4=CVM合约调用,5=JSVM合约调用,6=evfs交易,7=链委员会,8=链管理员组
*
*
* .com.brewchain.sdk.model.SendTransaction.CodeType innerCodeType = 6;
*/
public int getInnerCodeTypeValue() {
return innerCodeType_;
}
/**
*
*内置指令交易[0=普通交易,1=多重签名交易,2=RC20交易,3=RC721交易,4=CVM合约调用,5=JSVM合约调用,6=evfs交易,7=链委员会,8=链管理员组
*
*
* .com.brewchain.sdk.model.SendTransaction.CodeType innerCodeType = 6;
*/
public com.brewchain.sdk.model.Model.SendTransaction.CodeType getInnerCodeType() {
@SuppressWarnings("deprecation")
com.brewchain.sdk.model.Model.SendTransaction.CodeType result = com.brewchain.sdk.model.Model.SendTransaction.CodeType.valueOf(innerCodeType_);
return result == null ? com.brewchain.sdk.model.Model.SendTransaction.CodeType.UNRECOGNIZED : result;
}
public static final int CODEDATA_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString codeData_;
/**
*
*指令数据
*
*
* bytes codeData = 7;
*/
public com.google.protobuf.ByteString getCodeData() {
return codeData_;
}
public static final int EXDATA_FIELD_NUMBER = 8;
private volatile java.lang.Object exData_;
/**
* string exData = 8;
*/
public java.lang.String getExData() {
java.lang.Object ref = exData_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
exData_ = s;
return s;
}
}
/**
* string exData = 8;
*/
public com.google.protobuf.ByteString
getExDataBytes() {
java.lang.Object ref = exData_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
exData_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMESTAMP_FIELD_NUMBER = 9;
private long timestamp_;
/**
* int64 timestamp = 9;
*/
public long getTimestamp() {
return timestamp_;
}
public static final int PRIVATEKEY_FIELD_NUMBER = 10;
private volatile java.lang.Object privateKey_;
/**
* string privateKey = 10;
*/
public java.lang.String getPrivateKey() {
java.lang.Object ref = privateKey_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
privateKey_ = s;
return s;
}
}
/**
* string privateKey = 10;
*/
public com.google.protobuf.ByteString
getPrivateKeyBytes() {
java.lang.Object ref = privateKey_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
privateKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, address_);
}
if (nonce_ != 0) {
output.writeInt32(2, nonce_);
}
if (feeHi_ != 0L) {
output.writeInt64(4, feeHi_);
}
if (feeLow_ != 0L) {
output.writeInt64(5, feeLow_);
}
if (innerCodeType_ != com.brewchain.sdk.model.Model.SendTransaction.CodeType.NORMAL.getNumber()) {
output.writeEnum(6, innerCodeType_);
}
if (!codeData_.isEmpty()) {
output.writeBytes(7, codeData_);
}
if (!getExDataBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, exData_);
}
if (timestamp_ != 0L) {
output.writeInt64(9, timestamp_);
}
if (!getPrivateKeyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, privateKey_);
}
for (int i = 0; i < outputs_.size(); i++) {
output.writeMessage(36, outputs_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, address_);
}
if (nonce_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, nonce_);
}
if (feeHi_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, feeHi_);
}
if (feeLow_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, feeLow_);
}
if (innerCodeType_ != com.brewchain.sdk.model.Model.SendTransaction.CodeType.NORMAL.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, innerCodeType_);
}
if (!codeData_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, codeData_);
}
if (!getExDataBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, exData_);
}
if (timestamp_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(9, timestamp_);
}
if (!getPrivateKeyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, privateKey_);
}
for (int i = 0; i < outputs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(36, outputs_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.brewchain.sdk.model.Model.SendTransaction)) {
return super.equals(obj);
}
com.brewchain.sdk.model.Model.SendTransaction other = (com.brewchain.sdk.model.Model.SendTransaction) obj;
boolean result = true;
result = result && getAddress()
.equals(other.getAddress());
result = result && (getNonce()
== other.getNonce());
result = result && getOutputsList()
.equals(other.getOutputsList());
result = result && (getFeeHi()
== other.getFeeHi());
result = result && (getFeeLow()
== other.getFeeLow());
result = result && innerCodeType_ == other.innerCodeType_;
result = result && getCodeData()
.equals(other.getCodeData());
result = result && getExData()
.equals(other.getExData());
result = result && (getTimestamp()
== other.getTimestamp());
result = result && getPrivateKey()
.equals(other.getPrivateKey());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress().hashCode();
hash = (37 * hash) + NONCE_FIELD_NUMBER;
hash = (53 * hash) + getNonce();
if (getOutputsCount() > 0) {
hash = (37 * hash) + OUTPUTS_FIELD_NUMBER;
hash = (53 * hash) + getOutputsList().hashCode();
}
hash = (37 * hash) + FEEHI_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFeeHi());
hash = (37 * hash) + FEELOW_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFeeLow());
hash = (37 * hash) + INNERCODETYPE_FIELD_NUMBER;
hash = (53 * hash) + innerCodeType_;
hash = (37 * hash) + CODEDATA_FIELD_NUMBER;
hash = (53 * hash) + getCodeData().hashCode();
hash = (37 * hash) + EXDATA_FIELD_NUMBER;
hash = (53 * hash) + getExData().hashCode();
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
hash = (37 * hash) + PRIVATEKEY_FIELD_NUMBER;
hash = (53 * hash) + getPrivateKey().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.brewchain.sdk.model.Model.SendTransaction parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.brewchain.sdk.model.Model.SendTransaction parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.brewchain.sdk.model.Model.SendTransaction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.brewchain.sdk.model.Model.SendTransaction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.brewchain.sdk.model.Model.SendTransaction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.brewchain.sdk.model.Model.SendTransaction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.brewchain.sdk.model.Model.SendTransaction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.brewchain.sdk.model.Model.SendTransaction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.brewchain.sdk.model.Model.SendTransaction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.brewchain.sdk.model.Model.SendTransaction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.brewchain.sdk.model.Model.SendTransaction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.brewchain.sdk.model.Model.SendTransaction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.brewchain.sdk.model.Model.SendTransaction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.brewchain.sdk.model.SendTransaction}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.brewchain.sdk.model.SendTransaction)
com.brewchain.sdk.model.Model.SendTransactionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.brewchain.sdk.model.Model.internal_static_com_brewchain_sdk_model_SendTransaction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.brewchain.sdk.model.Model.internal_static_com_brewchain_sdk_model_SendTransaction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.brewchain.sdk.model.Model.SendTransaction.class, com.brewchain.sdk.model.Model.SendTransaction.Builder.class);
}
// Construct using com.brewchain.sdk.model.Model.SendTransaction.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getOutputsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
address_ = "";
nonce_ = 0;
if (outputsBuilder_ == null) {
outputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
outputsBuilder_.clear();
}
feeHi_ = 0L;
feeLow_ = 0L;
innerCodeType_ = 0;
codeData_ = com.google.protobuf.ByteString.EMPTY;
exData_ = "";
timestamp_ = 0L;
privateKey_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.brewchain.sdk.model.Model.internal_static_com_brewchain_sdk_model_SendTransaction_descriptor;
}
@java.lang.Override
public com.brewchain.sdk.model.Model.SendTransaction getDefaultInstanceForType() {
return com.brewchain.sdk.model.Model.SendTransaction.getDefaultInstance();
}
@java.lang.Override
public com.brewchain.sdk.model.Model.SendTransaction build() {
com.brewchain.sdk.model.Model.SendTransaction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.brewchain.sdk.model.Model.SendTransaction buildPartial() {
com.brewchain.sdk.model.Model.SendTransaction result = new com.brewchain.sdk.model.Model.SendTransaction(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.address_ = address_;
result.nonce_ = nonce_;
if (outputsBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
outputs_ = java.util.Collections.unmodifiableList(outputs_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.outputs_ = outputs_;
} else {
result.outputs_ = outputsBuilder_.build();
}
result.feeHi_ = feeHi_;
result.feeLow_ = feeLow_;
result.innerCodeType_ = innerCodeType_;
result.codeData_ = codeData_;
result.exData_ = exData_;
result.timestamp_ = timestamp_;
result.privateKey_ = privateKey_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.brewchain.sdk.model.Model.SendTransaction) {
return mergeFrom((com.brewchain.sdk.model.Model.SendTransaction)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.brewchain.sdk.model.Model.SendTransaction other) {
if (other == com.brewchain.sdk.model.Model.SendTransaction.getDefaultInstance()) return this;
if (!other.getAddress().isEmpty()) {
address_ = other.address_;
onChanged();
}
if (other.getNonce() != 0) {
setNonce(other.getNonce());
}
if (outputsBuilder_ == null) {
if (!other.outputs_.isEmpty()) {
if (outputs_.isEmpty()) {
outputs_ = other.outputs_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureOutputsIsMutable();
outputs_.addAll(other.outputs_);
}
onChanged();
}
} else {
if (!other.outputs_.isEmpty()) {
if (outputsBuilder_.isEmpty()) {
outputsBuilder_.dispose();
outputsBuilder_ = null;
outputs_ = other.outputs_;
bitField0_ = (bitField0_ & ~0x00000004);
outputsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOutputsFieldBuilder() : null;
} else {
outputsBuilder_.addAllMessages(other.outputs_);
}
}
}
if (other.getFeeHi() != 0L) {
setFeeHi(other.getFeeHi());
}
if (other.getFeeLow() != 0L) {
setFeeLow(other.getFeeLow());
}
if (other.innerCodeType_ != 0) {
setInnerCodeTypeValue(other.getInnerCodeTypeValue());
}
if (other.getCodeData() != com.google.protobuf.ByteString.EMPTY) {
setCodeData(other.getCodeData());
}
if (!other.getExData().isEmpty()) {
exData_ = other.exData_;
onChanged();
}
if (other.getTimestamp() != 0L) {
setTimestamp(other.getTimestamp());
}
if (!other.getPrivateKey().isEmpty()) {
privateKey_ = other.privateKey_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.brewchain.sdk.model.Model.SendTransaction parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.brewchain.sdk.model.Model.SendTransaction) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object address_ = "";
/**
* string address = 1;
*/
public java.lang.String getAddress() {
java.lang.Object ref = address_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
address_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string address = 1;
*/
public com.google.protobuf.ByteString
getAddressBytes() {
java.lang.Object ref = address_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
address_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string address = 1;
*/
public Builder setAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
address_ = value;
onChanged();
return this;
}
/**
* string address = 1;
*/
public Builder clearAddress() {
address_ = getDefaultInstance().getAddress();
onChanged();
return this;
}
/**
* string address = 1;
*/
public Builder setAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
address_ = value;
onChanged();
return this;
}
private int nonce_ ;
/**
* int32 nonce = 2;
*/
public int getNonce() {
return nonce_;
}
/**
* int32 nonce = 2;
*/
public Builder setNonce(int value) {
nonce_ = value;
onChanged();
return this;
}
/**
* int32 nonce = 2;
*/
public Builder clearNonce() {
nonce_ = 0;
onChanged();
return this;
}
private java.util.List outputs_ =
java.util.Collections.emptyList();
private void ensureOutputsIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
outputs_ = new java.util.ArrayList(outputs_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.brewchain.sdk.model.Model.SendTransactionOutput, com.brewchain.sdk.model.Model.SendTransactionOutput.Builder, com.brewchain.sdk.model.Model.SendTransactionOutputOrBuilder> outputsBuilder_;
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public java.util.List getOutputsList() {
if (outputsBuilder_ == null) {
return java.util.Collections.unmodifiableList(outputs_);
} else {
return outputsBuilder_.getMessageList();
}
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public int getOutputsCount() {
if (outputsBuilder_ == null) {
return outputs_.size();
} else {
return outputsBuilder_.getCount();
}
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public com.brewchain.sdk.model.Model.SendTransactionOutput getOutputs(int index) {
if (outputsBuilder_ == null) {
return outputs_.get(index);
} else {
return outputsBuilder_.getMessage(index);
}
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public Builder setOutputs(
int index, com.brewchain.sdk.model.Model.SendTransactionOutput value) {
if (outputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputsIsMutable();
outputs_.set(index, value);
onChanged();
} else {
outputsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public Builder setOutputs(
int index, com.brewchain.sdk.model.Model.SendTransactionOutput.Builder builderForValue) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.set(index, builderForValue.build());
onChanged();
} else {
outputsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public Builder addOutputs(com.brewchain.sdk.model.Model.SendTransactionOutput value) {
if (outputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputsIsMutable();
outputs_.add(value);
onChanged();
} else {
outputsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public Builder addOutputs(
int index, com.brewchain.sdk.model.Model.SendTransactionOutput value) {
if (outputsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputsIsMutable();
outputs_.add(index, value);
onChanged();
} else {
outputsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public Builder addOutputs(
com.brewchain.sdk.model.Model.SendTransactionOutput.Builder builderForValue) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.add(builderForValue.build());
onChanged();
} else {
outputsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public Builder addOutputs(
int index, com.brewchain.sdk.model.Model.SendTransactionOutput.Builder builderForValue) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.add(index, builderForValue.build());
onChanged();
} else {
outputsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public Builder addAllOutputs(
java.lang.Iterable extends com.brewchain.sdk.model.Model.SendTransactionOutput> values) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, outputs_);
onChanged();
} else {
outputsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public Builder clearOutputs() {
if (outputsBuilder_ == null) {
outputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
outputsBuilder_.clear();
}
return this;
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public Builder removeOutputs(int index) {
if (outputsBuilder_ == null) {
ensureOutputsIsMutable();
outputs_.remove(index);
onChanged();
} else {
outputsBuilder_.remove(index);
}
return this;
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public com.brewchain.sdk.model.Model.SendTransactionOutput.Builder getOutputsBuilder(
int index) {
return getOutputsFieldBuilder().getBuilder(index);
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public com.brewchain.sdk.model.Model.SendTransactionOutputOrBuilder getOutputsOrBuilder(
int index) {
if (outputsBuilder_ == null) {
return outputs_.get(index); } else {
return outputsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public java.util.List extends com.brewchain.sdk.model.Model.SendTransactionOutputOrBuilder>
getOutputsOrBuilderList() {
if (outputsBuilder_ != null) {
return outputsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(outputs_);
}
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public com.brewchain.sdk.model.Model.SendTransactionOutput.Builder addOutputsBuilder() {
return getOutputsFieldBuilder().addBuilder(
com.brewchain.sdk.model.Model.SendTransactionOutput.getDefaultInstance());
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public com.brewchain.sdk.model.Model.SendTransactionOutput.Builder addOutputsBuilder(
int index) {
return getOutputsFieldBuilder().addBuilder(
index, com.brewchain.sdk.model.Model.SendTransactionOutput.getDefaultInstance());
}
/**
* repeated .com.brewchain.sdk.model.SendTransactionOutput outputs = 36;
*/
public java.util.List
getOutputsBuilderList() {
return getOutputsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.brewchain.sdk.model.Model.SendTransactionOutput, com.brewchain.sdk.model.Model.SendTransactionOutput.Builder, com.brewchain.sdk.model.Model.SendTransactionOutputOrBuilder>
getOutputsFieldBuilder() {
if (outputsBuilder_ == null) {
outputsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.brewchain.sdk.model.Model.SendTransactionOutput, com.brewchain.sdk.model.Model.SendTransactionOutput.Builder, com.brewchain.sdk.model.Model.SendTransactionOutputOrBuilder>(
outputs_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
outputs_ = null;
}
return outputsBuilder_;
}
private long feeHi_ ;
/**
*
*手续费高位
*
*
* int64 feeHi = 4;
*/
public long getFeeHi() {
return feeHi_;
}
/**
*
*手续费高位
*
*
* int64 feeHi = 4;
*/
public Builder setFeeHi(long value) {
feeHi_ = value;
onChanged();
return this;
}
/**
*
*手续费高位
*
*
* int64 feeHi = 4;
*/
public Builder clearFeeHi() {
feeHi_ = 0L;
onChanged();
return this;
}
private long feeLow_ ;
/**
*
*手续费低位;
*
*
* int64 feeLow = 5;
*/
public long getFeeLow() {
return feeLow_;
}
/**
*
*手续费低位;
*
*
* int64 feeLow = 5;
*/
public Builder setFeeLow(long value) {
feeLow_ = value;
onChanged();
return this;
}
/**
*
*手续费低位;
*
*
* int64 feeLow = 5;
*/
public Builder clearFeeLow() {
feeLow_ = 0L;
onChanged();
return this;
}
private int innerCodeType_ = 0;
/**
*
*内置指令交易[0=普通交易,1=多重签名交易,2=RC20交易,3=RC721交易,4=CVM合约调用,5=JSVM合约调用,6=evfs交易,7=链委员会,8=链管理员组
*
*
* .com.brewchain.sdk.model.SendTransaction.CodeType innerCodeType = 6;
*/
public int getInnerCodeTypeValue() {
return innerCodeType_;
}
/**
*
*内置指令交易[0=普通交易,1=多重签名交易,2=RC20交易,3=RC721交易,4=CVM合约调用,5=JSVM合约调用,6=evfs交易,7=链委员会,8=链管理员组
*
*
* .com.brewchain.sdk.model.SendTransaction.CodeType innerCodeType = 6;
*/
public Builder setInnerCodeTypeValue(int value) {
innerCodeType_ = value;
onChanged();
return this;
}
/**
*
*内置指令交易[0=普通交易,1=多重签名交易,2=RC20交易,3=RC721交易,4=CVM合约调用,5=JSVM合约调用,6=evfs交易,7=链委员会,8=链管理员组
*
*
* .com.brewchain.sdk.model.SendTransaction.CodeType innerCodeType = 6;
*/
public com.brewchain.sdk.model.Model.SendTransaction.CodeType getInnerCodeType() {
@SuppressWarnings("deprecation")
com.brewchain.sdk.model.Model.SendTransaction.CodeType result = com.brewchain.sdk.model.Model.SendTransaction.CodeType.valueOf(innerCodeType_);
return result == null ? com.brewchain.sdk.model.Model.SendTransaction.CodeType.UNRECOGNIZED : result;
}
/**
*
*内置指令交易[0=普通交易,1=多重签名交易,2=RC20交易,3=RC721交易,4=CVM合约调用,5=JSVM合约调用,6=evfs交易,7=链委员会,8=链管理员组
*
*
* .com.brewchain.sdk.model.SendTransaction.CodeType innerCodeType = 6;
*/
public Builder setInnerCodeType(com.brewchain.sdk.model.Model.SendTransaction.CodeType value) {
if (value == null) {
throw new NullPointerException();
}
innerCodeType_ = value.getNumber();
onChanged();
return this;
}
/**
*
*内置指令交易[0=普通交易,1=多重签名交易,2=RC20交易,3=RC721交易,4=CVM合约调用,5=JSVM合约调用,6=evfs交易,7=链委员会,8=链管理员组
*
*
* .com.brewchain.sdk.model.SendTransaction.CodeType innerCodeType = 6;
*/
public Builder clearInnerCodeType() {
innerCodeType_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString codeData_ = com.google.protobuf.ByteString.EMPTY;
/**
*
*指令数据
*
*
* bytes codeData = 7;
*/
public com.google.protobuf.ByteString getCodeData() {
return codeData_;
}
/**
*
*指令数据
*
*
* bytes codeData = 7;
*/
public Builder setCodeData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
codeData_ = value;
onChanged();
return this;
}
/**
*
*指令数据
*
*
* bytes codeData = 7;
*/
public Builder clearCodeData() {
codeData_ = getDefaultInstance().getCodeData();
onChanged();
return this;
}
private java.lang.Object exData_ = "";
/**
* string exData = 8;
*/
public java.lang.String getExData() {
java.lang.Object ref = exData_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
exData_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string exData = 8;
*/
public com.google.protobuf.ByteString
getExDataBytes() {
java.lang.Object ref = exData_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
exData_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string exData = 8;
*/
public Builder setExData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
exData_ = value;
onChanged();
return this;
}
/**
* string exData = 8;
*/
public Builder clearExData() {
exData_ = getDefaultInstance().getExData();
onChanged();
return this;
}
/**
* string exData = 8;
*/
public Builder setExDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
exData_ = value;
onChanged();
return this;
}
private long timestamp_ ;
/**
* int64 timestamp = 9;
*/
public long getTimestamp() {
return timestamp_;
}
/**
* int64 timestamp = 9;
*/
public Builder setTimestamp(long value) {
timestamp_ = value;
onChanged();
return this;
}
/**
* int64 timestamp = 9;
*/
public Builder clearTimestamp() {
timestamp_ = 0L;
onChanged();
return this;
}
private java.lang.Object privateKey_ = "";
/**
* string privateKey = 10;
*/
public java.lang.String getPrivateKey() {
java.lang.Object ref = privateKey_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
privateKey_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string privateKey = 10;
*/
public com.google.protobuf.ByteString
getPrivateKeyBytes() {
java.lang.Object ref = privateKey_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
privateKey_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string privateKey = 10;
*/
public Builder setPrivateKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
privateKey_ = value;
onChanged();
return this;
}
/**
* string privateKey = 10;
*/
public Builder clearPrivateKey() {
privateKey_ = getDefaultInstance().getPrivateKey();
onChanged();
return this;
}
/**
* string privateKey = 10;
*/
public Builder setPrivateKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
privateKey_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.brewchain.sdk.model.SendTransaction)
}
// @@protoc_insertion_point(class_scope:com.brewchain.sdk.model.SendTransaction)
private static final com.brewchain.sdk.model.Model.SendTransaction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.brewchain.sdk.model.Model.SendTransaction();
}
public static com.brewchain.sdk.model.Model.SendTransaction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SendTransaction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SendTransaction(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.brewchain.sdk.model.Model.SendTransaction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SendTransactionOutputOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.brewchain.sdk.model.SendTransactionOutput)
com.google.protobuf.MessageOrBuilder {
/**
* string address = 1;
*/
java.lang.String getAddress();
/**
* string address = 1;
*/
com.google.protobuf.ByteString
getAddressBytes();
/**
* string amount = 2;
*/
java.lang.String getAmount();
/**
* string amount = 2;
*/
com.google.protobuf.ByteString
getAmountBytes();
/**
* string token = 3;
*/
java.lang.String getToken();
/**
* string token = 3;
*/
com.google.protobuf.ByteString
getTokenBytes();
/**
* string tokenAmount = 4;
*/
java.lang.String getTokenAmount();
/**
* string tokenAmount = 4;
*/
com.google.protobuf.ByteString
getTokenAmountBytes();
/**
* string symbol = 5;
*/
java.lang.String getSymbol();
/**
* string symbol = 5;
*/
com.google.protobuf.ByteString
getSymbolBytes();
/**
* repeated string cryptoToken = 6;
*/
java.util.List
getCryptoTokenList();
/**
* repeated string cryptoToken = 6;
*/
int getCryptoTokenCount();
/**
* repeated string cryptoToken = 6;
*/
java.lang.String getCryptoToken(int index);
/**
* repeated string cryptoToken = 6;
*/
com.google.protobuf.ByteString
getCryptoTokenBytes(int index);
}
/**
* Protobuf type {@code com.brewchain.sdk.model.SendTransactionOutput}
*/
public static final class SendTransactionOutput extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.brewchain.sdk.model.SendTransactionOutput)
SendTransactionOutputOrBuilder {
private static final long serialVersionUID = 0L;
// Use SendTransactionOutput.newBuilder() to construct.
private SendTransactionOutput(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SendTransactionOutput() {
address_ = "";
amount_ = "";
token_ = "";
tokenAmount_ = "";
symbol_ = "";
cryptoToken_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SendTransactionOutput(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
address_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
amount_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
token_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
tokenAmount_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
symbol_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
cryptoToken_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000020;
}
cryptoToken_.add(s);
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
cryptoToken_ = cryptoToken_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.brewchain.sdk.model.Model.internal_static_com_brewchain_sdk_model_SendTransactionOutput_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.brewchain.sdk.model.Model.internal_static_com_brewchain_sdk_model_SendTransactionOutput_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.brewchain.sdk.model.Model.SendTransactionOutput.class, com.brewchain.sdk.model.Model.SendTransactionOutput.Builder.class);
}
private int bitField0_;
public static final int ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object address_;
/**
* string address = 1;
*/
public java.lang.String getAddress() {
java.lang.Object ref = address_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
address_ = s;
return s;
}
}
/**
* string address = 1;
*/
public com.google.protobuf.ByteString
getAddressBytes() {
java.lang.Object ref = address_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
address_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AMOUNT_FIELD_NUMBER = 2;
private volatile java.lang.Object amount_;
/**
* string amount = 2;
*/
public java.lang.String getAmount() {
java.lang.Object ref = amount_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
amount_ = s;
return s;
}
}
/**
* string amount = 2;
*/
public com.google.protobuf.ByteString
getAmountBytes() {
java.lang.Object ref = amount_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
amount_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TOKEN_FIELD_NUMBER = 3;
private volatile java.lang.Object token_;
/**
* string token = 3;
*/
public java.lang.String getToken() {
java.lang.Object ref = token_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
token_ = s;
return s;
}
}
/**
* string token = 3;
*/
public com.google.protobuf.ByteString
getTokenBytes() {
java.lang.Object ref = token_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
token_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TOKENAMOUNT_FIELD_NUMBER = 4;
private volatile java.lang.Object tokenAmount_;
/**
* string tokenAmount = 4;
*/
public java.lang.String getTokenAmount() {
java.lang.Object ref = tokenAmount_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tokenAmount_ = s;
return s;
}
}
/**
* string tokenAmount = 4;
*/
public com.google.protobuf.ByteString
getTokenAmountBytes() {
java.lang.Object ref = tokenAmount_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tokenAmount_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SYMBOL_FIELD_NUMBER = 5;
private volatile java.lang.Object symbol_;
/**
* string symbol = 5;
*/
public java.lang.String getSymbol() {
java.lang.Object ref = symbol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
symbol_ = s;
return s;
}
}
/**
* string symbol = 5;
*/
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = symbol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
symbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CRYPTOTOKEN_FIELD_NUMBER = 6;
private com.google.protobuf.LazyStringList cryptoToken_;
/**
* repeated string cryptoToken = 6;
*/
public com.google.protobuf.ProtocolStringList
getCryptoTokenList() {
return cryptoToken_;
}
/**
* repeated string cryptoToken = 6;
*/
public int getCryptoTokenCount() {
return cryptoToken_.size();
}
/**
* repeated string cryptoToken = 6;
*/
public java.lang.String getCryptoToken(int index) {
return cryptoToken_.get(index);
}
/**
* repeated string cryptoToken = 6;
*/
public com.google.protobuf.ByteString
getCryptoTokenBytes(int index) {
return cryptoToken_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, address_);
}
if (!getAmountBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, amount_);
}
if (!getTokenBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, token_);
}
if (!getTokenAmountBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, tokenAmount_);
}
if (!getSymbolBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, symbol_);
}
for (int i = 0; i < cryptoToken_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, cryptoToken_.getRaw(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, address_);
}
if (!getAmountBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, amount_);
}
if (!getTokenBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, token_);
}
if (!getTokenAmountBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, tokenAmount_);
}
if (!getSymbolBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, symbol_);
}
{
int dataSize = 0;
for (int i = 0; i < cryptoToken_.size(); i++) {
dataSize += computeStringSizeNoTag(cryptoToken_.getRaw(i));
}
size += dataSize;
size += 1 * getCryptoTokenList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.brewchain.sdk.model.Model.SendTransactionOutput)) {
return super.equals(obj);
}
com.brewchain.sdk.model.Model.SendTransactionOutput other = (com.brewchain.sdk.model.Model.SendTransactionOutput) obj;
boolean result = true;
result = result && getAddress()
.equals(other.getAddress());
result = result && getAmount()
.equals(other.getAmount());
result = result && getToken()
.equals(other.getToken());
result = result && getTokenAmount()
.equals(other.getTokenAmount());
result = result && getSymbol()
.equals(other.getSymbol());
result = result && getCryptoTokenList()
.equals(other.getCryptoTokenList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress().hashCode();
hash = (37 * hash) + AMOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAmount().hashCode();
hash = (37 * hash) + TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getToken().hashCode();
hash = (37 * hash) + TOKENAMOUNT_FIELD_NUMBER;
hash = (53 * hash) + getTokenAmount().hashCode();
hash = (37 * hash) + SYMBOL_FIELD_NUMBER;
hash = (53 * hash) + getSymbol().hashCode();
if (getCryptoTokenCount() > 0) {
hash = (37 * hash) + CRYPTOTOKEN_FIELD_NUMBER;
hash = (53 * hash) + getCryptoTokenList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.brewchain.sdk.model.Model.SendTransactionOutput parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.brewchain.sdk.model.Model.SendTransactionOutput parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.brewchain.sdk.model.Model.SendTransactionOutput parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.brewchain.sdk.model.Model.SendTransactionOutput parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.brewchain.sdk.model.Model.SendTransactionOutput parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.brewchain.sdk.model.Model.SendTransactionOutput parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.brewchain.sdk.model.Model.SendTransactionOutput parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.brewchain.sdk.model.Model.SendTransactionOutput parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.brewchain.sdk.model.Model.SendTransactionOutput parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.brewchain.sdk.model.Model.SendTransactionOutput parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.brewchain.sdk.model.Model.SendTransactionOutput parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.brewchain.sdk.model.Model.SendTransactionOutput parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.brewchain.sdk.model.Model.SendTransactionOutput prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.brewchain.sdk.model.SendTransactionOutput}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.brewchain.sdk.model.SendTransactionOutput)
com.brewchain.sdk.model.Model.SendTransactionOutputOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.brewchain.sdk.model.Model.internal_static_com_brewchain_sdk_model_SendTransactionOutput_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.brewchain.sdk.model.Model.internal_static_com_brewchain_sdk_model_SendTransactionOutput_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.brewchain.sdk.model.Model.SendTransactionOutput.class, com.brewchain.sdk.model.Model.SendTransactionOutput.Builder.class);
}
// Construct using com.brewchain.sdk.model.Model.SendTransactionOutput.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
address_ = "";
amount_ = "";
token_ = "";
tokenAmount_ = "";
symbol_ = "";
cryptoToken_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.brewchain.sdk.model.Model.internal_static_com_brewchain_sdk_model_SendTransactionOutput_descriptor;
}
@java.lang.Override
public com.brewchain.sdk.model.Model.SendTransactionOutput getDefaultInstanceForType() {
return com.brewchain.sdk.model.Model.SendTransactionOutput.getDefaultInstance();
}
@java.lang.Override
public com.brewchain.sdk.model.Model.SendTransactionOutput build() {
com.brewchain.sdk.model.Model.SendTransactionOutput result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.brewchain.sdk.model.Model.SendTransactionOutput buildPartial() {
com.brewchain.sdk.model.Model.SendTransactionOutput result = new com.brewchain.sdk.model.Model.SendTransactionOutput(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.address_ = address_;
result.amount_ = amount_;
result.token_ = token_;
result.tokenAmount_ = tokenAmount_;
result.symbol_ = symbol_;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
cryptoToken_ = cryptoToken_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000020);
}
result.cryptoToken_ = cryptoToken_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.brewchain.sdk.model.Model.SendTransactionOutput) {
return mergeFrom((com.brewchain.sdk.model.Model.SendTransactionOutput)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.brewchain.sdk.model.Model.SendTransactionOutput other) {
if (other == com.brewchain.sdk.model.Model.SendTransactionOutput.getDefaultInstance()) return this;
if (!other.getAddress().isEmpty()) {
address_ = other.address_;
onChanged();
}
if (!other.getAmount().isEmpty()) {
amount_ = other.amount_;
onChanged();
}
if (!other.getToken().isEmpty()) {
token_ = other.token_;
onChanged();
}
if (!other.getTokenAmount().isEmpty()) {
tokenAmount_ = other.tokenAmount_;
onChanged();
}
if (!other.getSymbol().isEmpty()) {
symbol_ = other.symbol_;
onChanged();
}
if (!other.cryptoToken_.isEmpty()) {
if (cryptoToken_.isEmpty()) {
cryptoToken_ = other.cryptoToken_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureCryptoTokenIsMutable();
cryptoToken_.addAll(other.cryptoToken_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.brewchain.sdk.model.Model.SendTransactionOutput parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.brewchain.sdk.model.Model.SendTransactionOutput) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object address_ = "";
/**
* string address = 1;
*/
public java.lang.String getAddress() {
java.lang.Object ref = address_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
address_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string address = 1;
*/
public com.google.protobuf.ByteString
getAddressBytes() {
java.lang.Object ref = address_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
address_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string address = 1;
*/
public Builder setAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
address_ = value;
onChanged();
return this;
}
/**
* string address = 1;
*/
public Builder clearAddress() {
address_ = getDefaultInstance().getAddress();
onChanged();
return this;
}
/**
* string address = 1;
*/
public Builder setAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
address_ = value;
onChanged();
return this;
}
private java.lang.Object amount_ = "";
/**
* string amount = 2;
*/
public java.lang.String getAmount() {
java.lang.Object ref = amount_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
amount_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string amount = 2;
*/
public com.google.protobuf.ByteString
getAmountBytes() {
java.lang.Object ref = amount_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
amount_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string amount = 2;
*/
public Builder setAmount(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
amount_ = value;
onChanged();
return this;
}
/**
* string amount = 2;
*/
public Builder clearAmount() {
amount_ = getDefaultInstance().getAmount();
onChanged();
return this;
}
/**
* string amount = 2;
*/
public Builder setAmountBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
amount_ = value;
onChanged();
return this;
}
private java.lang.Object token_ = "";
/**
* string token = 3;
*/
public java.lang.String getToken() {
java.lang.Object ref = token_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
token_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string token = 3;
*/
public com.google.protobuf.ByteString
getTokenBytes() {
java.lang.Object ref = token_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
token_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string token = 3;
*/
public Builder setToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
token_ = value;
onChanged();
return this;
}
/**
* string token = 3;
*/
public Builder clearToken() {
token_ = getDefaultInstance().getToken();
onChanged();
return this;
}
/**
* string token = 3;
*/
public Builder setTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
token_ = value;
onChanged();
return this;
}
private java.lang.Object tokenAmount_ = "";
/**
* string tokenAmount = 4;
*/
public java.lang.String getTokenAmount() {
java.lang.Object ref = tokenAmount_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tokenAmount_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string tokenAmount = 4;
*/
public com.google.protobuf.ByteString
getTokenAmountBytes() {
java.lang.Object ref = tokenAmount_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tokenAmount_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string tokenAmount = 4;
*/
public Builder setTokenAmount(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tokenAmount_ = value;
onChanged();
return this;
}
/**
* string tokenAmount = 4;
*/
public Builder clearTokenAmount() {
tokenAmount_ = getDefaultInstance().getTokenAmount();
onChanged();
return this;
}
/**
* string tokenAmount = 4;
*/
public Builder setTokenAmountBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tokenAmount_ = value;
onChanged();
return this;
}
private java.lang.Object symbol_ = "";
/**
* string symbol = 5;
*/
public java.lang.String getSymbol() {
java.lang.Object ref = symbol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
symbol_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string symbol = 5;
*/
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = symbol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
symbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string symbol = 5;
*/
public Builder setSymbol(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
symbol_ = value;
onChanged();
return this;
}
/**
* string symbol = 5;
*/
public Builder clearSymbol() {
symbol_ = getDefaultInstance().getSymbol();
onChanged();
return this;
}
/**
* string symbol = 5;
*/
public Builder setSymbolBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
symbol_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList cryptoToken_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureCryptoTokenIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
cryptoToken_ = new com.google.protobuf.LazyStringArrayList(cryptoToken_);
bitField0_ |= 0x00000020;
}
}
/**
* repeated string cryptoToken = 6;
*/
public com.google.protobuf.ProtocolStringList
getCryptoTokenList() {
return cryptoToken_.getUnmodifiableView();
}
/**
* repeated string cryptoToken = 6;
*/
public int getCryptoTokenCount() {
return cryptoToken_.size();
}
/**
* repeated string cryptoToken = 6;
*/
public java.lang.String getCryptoToken(int index) {
return cryptoToken_.get(index);
}
/**
* repeated string cryptoToken = 6;
*/
public com.google.protobuf.ByteString
getCryptoTokenBytes(int index) {
return cryptoToken_.getByteString(index);
}
/**
* repeated string cryptoToken = 6;
*/
public Builder setCryptoToken(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCryptoTokenIsMutable();
cryptoToken_.set(index, value);
onChanged();
return this;
}
/**
* repeated string cryptoToken = 6;
*/
public Builder addCryptoToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCryptoTokenIsMutable();
cryptoToken_.add(value);
onChanged();
return this;
}
/**
* repeated string cryptoToken = 6;
*/
public Builder addAllCryptoToken(
java.lang.Iterable values) {
ensureCryptoTokenIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, cryptoToken_);
onChanged();
return this;
}
/**
* repeated string cryptoToken = 6;
*/
public Builder clearCryptoToken() {
cryptoToken_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
* repeated string cryptoToken = 6;
*/
public Builder addCryptoTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureCryptoTokenIsMutable();
cryptoToken_.add(value);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.brewchain.sdk.model.SendTransactionOutput)
}
// @@protoc_insertion_point(class_scope:com.brewchain.sdk.model.SendTransactionOutput)
private static final com.brewchain.sdk.model.Model.SendTransactionOutput DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.brewchain.sdk.model.Model.SendTransactionOutput();
}
public static com.brewchain.sdk.model.Model.SendTransactionOutput getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SendTransactionOutput parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SendTransactionOutput(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.brewchain.sdk.model.Model.SendTransactionOutput getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_brewchain_sdk_model_SendTransaction_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_brewchain_sdk_model_SendTransaction_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_brewchain_sdk_model_SendTransactionOutput_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_brewchain_sdk_model_SendTransactionOutput_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\013Model.proto\022\027com.brewchain.sdk.model\"\227" +
"\003\n\017SendTransaction\022\017\n\007address\030\001 \001(\t\022\r\n\005n" +
"once\030\002 \001(\005\022?\n\007outputs\030$ \003(\0132..com.brewch" +
"ain.sdk.model.SendTransactionOutput\022\r\n\005f" +
"eeHi\030\004 \001(\003\022\016\n\006feeLow\030\005 \001(\003\022H\n\rinnerCodeT" +
"ype\030\006 \001(\01621.com.brewchain.sdk.model.Send" +
"Transaction.CodeType\022\020\n\010codeData\030\007 \001(\014\022\016" +
"\n\006exData\030\010 \001(\t\022\021\n\ttimestamp\030\t \001(\003\022\022\n\npri" +
"vateKey\030\n \001(\t\"q\n\010CodeType\022\n\n\006NORMAL\020\000\022\r\n" +
"\tMULI_SIGN\020\001\022\021\n\rRC20_CONTRACT\020\002\022\022\n\016RC721" +
"_CONTRACT\020\003\022\020\n\014CVM_CONTRACT\020\004\022\021\n\rJSVM_CO" +
"NTRACT\020\005\"\201\001\n\025SendTransactionOutput\022\017\n\007ad" +
"dress\030\001 \001(\t\022\016\n\006amount\030\002 \001(\t\022\r\n\005token\030\003 \001" +
"(\t\022\023\n\013tokenAmount\030\004 \001(\t\022\016\n\006symbol\030\005 \001(\t\022" +
"\023\n\013cryptoToken\030\006 \003(\t*6\n\024SendOwnerTokenOp" +
"Code\022\n\n\006PUBLIC\020\000\022\010\n\004BURN\020\001\022\010\n\004MINT\020\002b\006pr" +
"oto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_com_brewchain_sdk_model_SendTransaction_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_com_brewchain_sdk_model_SendTransaction_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_brewchain_sdk_model_SendTransaction_descriptor,
new java.lang.String[] { "Address", "Nonce", "Outputs", "FeeHi", "FeeLow", "InnerCodeType", "CodeData", "ExData", "Timestamp", "PrivateKey", });
internal_static_com_brewchain_sdk_model_SendTransactionOutput_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_com_brewchain_sdk_model_SendTransactionOutput_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_brewchain_sdk_model_SendTransactionOutput_descriptor,
new java.lang.String[] { "Address", "Amount", "Token", "TokenAmount", "Symbol", "CryptoToken", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy