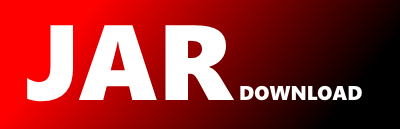
org.brewchain.sdk.model.Block Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cwvj Show documentation
Show all versions of cwvj Show documentation
Java sdk for Dapps interact with cwv blockchain node
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: block.proto
package org.brewchain.sdk.model;
public final class Block {
private Block() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface BlockInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.chain.sdk.model.BlockInfo)
com.google.protobuf.MessageOrBuilder {
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
boolean hasHeader();
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
Block.BlockHeader getHeader();
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
Block.BlockHeaderOrBuilder getHeaderOrBuilder();
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
boolean hasBody();
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
Block.BlockBody getBody();
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
Block.BlockBodyOrBuilder getBodyOrBuilder();
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
boolean hasMiner();
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
Block.BlockMiner getMiner();
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
Block.BlockMinerOrBuilder getMinerOrBuilder();
/**
* int32 version = 4;
*/
int getVersion();
}
/**
* Protobuf type {@code com.chain.sdk.model.BlockInfo}
*/
public static final class BlockInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.chain.sdk.model.BlockInfo)
BlockInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use BlockInfo.newBuilder() to construct.
private BlockInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BlockInfo() {
version_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BlockInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
Block.BlockHeader.Builder subBuilder = null;
if (header_ != null) {
subBuilder = header_.toBuilder();
}
header_ = input.readMessage(Block.BlockHeader.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(header_);
header_ = subBuilder.buildPartial();
}
break;
}
case 18: {
Block.BlockBody.Builder subBuilder = null;
if (body_ != null) {
subBuilder = body_.toBuilder();
}
body_ = input.readMessage(Block.BlockBody.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(body_);
body_ = subBuilder.buildPartial();
}
break;
}
case 26: {
Block.BlockMiner.Builder subBuilder = null;
if (miner_ != null) {
subBuilder = miner_.toBuilder();
}
miner_ = input.readMessage(Block.BlockMiner.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(miner_);
miner_ = subBuilder.buildPartial();
}
break;
}
case 32: {
version_ = input.readInt32();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Block.internal_static_com_chain_sdk_model_BlockInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return Block.internal_static_com_chain_sdk_model_BlockInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Block.BlockInfo.class, Block.BlockInfo.Builder.class);
}
public static final int HEADER_FIELD_NUMBER = 1;
private Block.BlockHeader header_;
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
public boolean hasHeader() {
return header_ != null;
}
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
public Block.BlockHeader getHeader() {
return header_ == null ? Block.BlockHeader.getDefaultInstance() : header_;
}
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
public Block.BlockHeaderOrBuilder getHeaderOrBuilder() {
return getHeader();
}
public static final int BODY_FIELD_NUMBER = 2;
private Block.BlockBody body_;
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
public boolean hasBody() {
return body_ != null;
}
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
public Block.BlockBody getBody() {
return body_ == null ? Block.BlockBody.getDefaultInstance() : body_;
}
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
public Block.BlockBodyOrBuilder getBodyOrBuilder() {
return getBody();
}
public static final int MINER_FIELD_NUMBER = 3;
private Block.BlockMiner miner_;
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
public boolean hasMiner() {
return miner_ != null;
}
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
public Block.BlockMiner getMiner() {
return miner_ == null ? Block.BlockMiner.getDefaultInstance() : miner_;
}
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
public Block.BlockMinerOrBuilder getMinerOrBuilder() {
return getMiner();
}
public static final int VERSION_FIELD_NUMBER = 4;
private int version_;
/**
* int32 version = 4;
*/
public int getVersion() {
return version_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (header_ != null) {
output.writeMessage(1, getHeader());
}
if (body_ != null) {
output.writeMessage(2, getBody());
}
if (miner_ != null) {
output.writeMessage(3, getMiner());
}
if (version_ != 0) {
output.writeInt32(4, version_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (header_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getHeader());
}
if (body_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getBody());
}
if (miner_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getMiner());
}
if (version_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, version_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof Block.BlockInfo)) {
return super.equals(obj);
}
Block.BlockInfo other = (Block.BlockInfo) obj;
boolean result = true;
result = result && (hasHeader() == other.hasHeader());
if (hasHeader()) {
result = result && getHeader()
.equals(other.getHeader());
}
result = result && (hasBody() == other.hasBody());
if (hasBody()) {
result = result && getBody()
.equals(other.getBody());
}
result = result && (hasMiner() == other.hasMiner());
if (hasMiner()) {
result = result && getMiner()
.equals(other.getMiner());
}
result = result && (getVersion()
== other.getVersion());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasHeader()) {
hash = (37 * hash) + HEADER_FIELD_NUMBER;
hash = (53 * hash) + getHeader().hashCode();
}
if (hasBody()) {
hash = (37 * hash) + BODY_FIELD_NUMBER;
hash = (53 * hash) + getBody().hashCode();
}
if (hasMiner()) {
hash = (37 * hash) + MINER_FIELD_NUMBER;
hash = (53 * hash) + getMiner().hashCode();
}
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static Block.BlockInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Block.BlockInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Block.BlockInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Block.BlockInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Block.BlockInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Block.BlockInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Block.BlockInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static Block.BlockInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static Block.BlockInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static Block.BlockInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static Block.BlockInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static Block.BlockInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(Block.BlockInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.chain.sdk.model.BlockInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.chain.sdk.model.BlockInfo)
Block.BlockInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Block.internal_static_com_chain_sdk_model_BlockInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return Block.internal_static_com_chain_sdk_model_BlockInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Block.BlockInfo.class, Block.BlockInfo.Builder.class);
}
// Construct using com.chain.sdk.model.Block.BlockInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (headerBuilder_ == null) {
header_ = null;
} else {
header_ = null;
headerBuilder_ = null;
}
if (bodyBuilder_ == null) {
body_ = null;
} else {
body_ = null;
bodyBuilder_ = null;
}
if (minerBuilder_ == null) {
miner_ = null;
} else {
miner_ = null;
minerBuilder_ = null;
}
version_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return Block.internal_static_com_chain_sdk_model_BlockInfo_descriptor;
}
@java.lang.Override
public Block.BlockInfo getDefaultInstanceForType() {
return Block.BlockInfo.getDefaultInstance();
}
@java.lang.Override
public Block.BlockInfo build() {
Block.BlockInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public Block.BlockInfo buildPartial() {
Block.BlockInfo result = new Block.BlockInfo(this);
if (headerBuilder_ == null) {
result.header_ = header_;
} else {
result.header_ = headerBuilder_.build();
}
if (bodyBuilder_ == null) {
result.body_ = body_;
} else {
result.body_ = bodyBuilder_.build();
}
if (minerBuilder_ == null) {
result.miner_ = miner_;
} else {
result.miner_ = minerBuilder_.build();
}
result.version_ = version_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Block.BlockInfo) {
return mergeFrom((Block.BlockInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Block.BlockInfo other) {
if (other == Block.BlockInfo.getDefaultInstance()) return this;
if (other.hasHeader()) {
mergeHeader(other.getHeader());
}
if (other.hasBody()) {
mergeBody(other.getBody());
}
if (other.hasMiner()) {
mergeMiner(other.getMiner());
}
if (other.getVersion() != 0) {
setVersion(other.getVersion());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Block.BlockInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Block.BlockInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private Block.BlockHeader header_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
Block.BlockHeader, Block.BlockHeader.Builder, Block.BlockHeaderOrBuilder> headerBuilder_;
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
public boolean hasHeader() {
return headerBuilder_ != null || header_ != null;
}
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
public Block.BlockHeader getHeader() {
if (headerBuilder_ == null) {
return header_ == null ? Block.BlockHeader.getDefaultInstance() : header_;
} else {
return headerBuilder_.getMessage();
}
}
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
public Builder setHeader(Block.BlockHeader value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
header_ = value;
onChanged();
} else {
headerBuilder_.setMessage(value);
}
return this;
}
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
public Builder setHeader(
Block.BlockHeader.Builder builderForValue) {
if (headerBuilder_ == null) {
header_ = builderForValue.build();
onChanged();
} else {
headerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
public Builder mergeHeader(Block.BlockHeader value) {
if (headerBuilder_ == null) {
if (header_ != null) {
header_ =
Block.BlockHeader.newBuilder(header_).mergeFrom(value).buildPartial();
} else {
header_ = value;
}
onChanged();
} else {
headerBuilder_.mergeFrom(value);
}
return this;
}
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
public Builder clearHeader() {
if (headerBuilder_ == null) {
header_ = null;
onChanged();
} else {
header_ = null;
headerBuilder_ = null;
}
return this;
}
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
public Block.BlockHeader.Builder getHeaderBuilder() {
onChanged();
return getHeaderFieldBuilder().getBuilder();
}
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
public Block.BlockHeaderOrBuilder getHeaderOrBuilder() {
if (headerBuilder_ != null) {
return headerBuilder_.getMessageOrBuilder();
} else {
return header_ == null ?
Block.BlockHeader.getDefaultInstance() : header_;
}
}
/**
* .com.chain.sdk.model.BlockHeader header = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
Block.BlockHeader, Block.BlockHeader.Builder, Block.BlockHeaderOrBuilder>
getHeaderFieldBuilder() {
if (headerBuilder_ == null) {
headerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
Block.BlockHeader, Block.BlockHeader.Builder, Block.BlockHeaderOrBuilder>(
getHeader(),
getParentForChildren(),
isClean());
header_ = null;
}
return headerBuilder_;
}
private Block.BlockBody body_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
Block.BlockBody, Block.BlockBody.Builder, Block.BlockBodyOrBuilder> bodyBuilder_;
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
public boolean hasBody() {
return bodyBuilder_ != null || body_ != null;
}
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
public Block.BlockBody getBody() {
if (bodyBuilder_ == null) {
return body_ == null ? Block.BlockBody.getDefaultInstance() : body_;
} else {
return bodyBuilder_.getMessage();
}
}
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
public Builder setBody(Block.BlockBody value) {
if (bodyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
body_ = value;
onChanged();
} else {
bodyBuilder_.setMessage(value);
}
return this;
}
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
public Builder setBody(
Block.BlockBody.Builder builderForValue) {
if (bodyBuilder_ == null) {
body_ = builderForValue.build();
onChanged();
} else {
bodyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
public Builder mergeBody(Block.BlockBody value) {
if (bodyBuilder_ == null) {
if (body_ != null) {
body_ =
Block.BlockBody.newBuilder(body_).mergeFrom(value).buildPartial();
} else {
body_ = value;
}
onChanged();
} else {
bodyBuilder_.mergeFrom(value);
}
return this;
}
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
public Builder clearBody() {
if (bodyBuilder_ == null) {
body_ = null;
onChanged();
} else {
body_ = null;
bodyBuilder_ = null;
}
return this;
}
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
public Block.BlockBody.Builder getBodyBuilder() {
onChanged();
return getBodyFieldBuilder().getBuilder();
}
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
public Block.BlockBodyOrBuilder getBodyOrBuilder() {
if (bodyBuilder_ != null) {
return bodyBuilder_.getMessageOrBuilder();
} else {
return body_ == null ?
Block.BlockBody.getDefaultInstance() : body_;
}
}
/**
* .com.chain.sdk.model.BlockBody body = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
Block.BlockBody, Block.BlockBody.Builder, Block.BlockBodyOrBuilder>
getBodyFieldBuilder() {
if (bodyBuilder_ == null) {
bodyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
Block.BlockBody, Block.BlockBody.Builder, Block.BlockBodyOrBuilder>(
getBody(),
getParentForChildren(),
isClean());
body_ = null;
}
return bodyBuilder_;
}
private Block.BlockMiner miner_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
Block.BlockMiner, Block.BlockMiner.Builder, Block.BlockMinerOrBuilder> minerBuilder_;
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
public boolean hasMiner() {
return minerBuilder_ != null || miner_ != null;
}
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
public Block.BlockMiner getMiner() {
if (minerBuilder_ == null) {
return miner_ == null ? Block.BlockMiner.getDefaultInstance() : miner_;
} else {
return minerBuilder_.getMessage();
}
}
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
public Builder setMiner(Block.BlockMiner value) {
if (minerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
miner_ = value;
onChanged();
} else {
minerBuilder_.setMessage(value);
}
return this;
}
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
public Builder setMiner(
Block.BlockMiner.Builder builderForValue) {
if (minerBuilder_ == null) {
miner_ = builderForValue.build();
onChanged();
} else {
minerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
public Builder mergeMiner(Block.BlockMiner value) {
if (minerBuilder_ == null) {
if (miner_ != null) {
miner_ =
Block.BlockMiner.newBuilder(miner_).mergeFrom(value).buildPartial();
} else {
miner_ = value;
}
onChanged();
} else {
minerBuilder_.mergeFrom(value);
}
return this;
}
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
public Builder clearMiner() {
if (minerBuilder_ == null) {
miner_ = null;
onChanged();
} else {
miner_ = null;
minerBuilder_ = null;
}
return this;
}
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
public Block.BlockMiner.Builder getMinerBuilder() {
onChanged();
return getMinerFieldBuilder().getBuilder();
}
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
public Block.BlockMinerOrBuilder getMinerOrBuilder() {
if (minerBuilder_ != null) {
return minerBuilder_.getMessageOrBuilder();
} else {
return miner_ == null ?
Block.BlockMiner.getDefaultInstance() : miner_;
}
}
/**
* .com.chain.sdk.model.BlockMiner miner = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
Block.BlockMiner, Block.BlockMiner.Builder, Block.BlockMinerOrBuilder>
getMinerFieldBuilder() {
if (minerBuilder_ == null) {
minerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
Block.BlockMiner, Block.BlockMiner.Builder, Block.BlockMinerOrBuilder>(
getMiner(),
getParentForChildren(),
isClean());
miner_ = null;
}
return minerBuilder_;
}
private int version_ ;
/**
* int32 version = 4;
*/
public int getVersion() {
return version_;
}
/**
* int32 version = 4;
*/
public Builder setVersion(int value) {
version_ = value;
onChanged();
return this;
}
/**
* int32 version = 4;
*/
public Builder clearVersion() {
version_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.chain.sdk.model.BlockInfo)
}
// @@protoc_insertion_point(class_scope:com.chain.sdk.model.BlockInfo)
private static final Block.BlockInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new Block.BlockInfo();
}
public static Block.BlockInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BlockInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BlockInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public Block.BlockInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BlockHeaderOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.chain.sdk.model.BlockHeader)
com.google.protobuf.MessageOrBuilder {
/**
* bytes hash = 1;
*/
com.google.protobuf.ByteString getHash();
/**
* bytes parentHash = 2;
*/
com.google.protobuf.ByteString getParentHash();
/**
* int64 height = 3;
*/
long getHeight();
/**
* bytes stateRoot = 4;
*/
com.google.protobuf.ByteString getStateRoot();
/**
* bytes receiptRoot = 5;
*/
com.google.protobuf.ByteString getReceiptRoot();
/**
* int64 timestamp = 6;
*/
long getTimestamp();
/**
* bytes extraData = 7;
*/
com.google.protobuf.ByteString getExtraData();
/**
* repeated bytes txHashs = 8;
*/
java.util.List getTxHashsList();
/**
* repeated bytes txHashs = 8;
*/
int getTxHashsCount();
/**
* repeated bytes txHashs = 8;
*/
com.google.protobuf.ByteString getTxHashs(int index);
/**
* repeated int32 txexecbulkindex = 9;
*/
java.util.List getTxexecbulkindexList();
/**
* repeated int32 txexecbulkindex = 9;
*/
int getTxexecbulkindexCount();
/**
* repeated int32 txexecbulkindex = 9;
*/
int getTxexecbulkindex(int index);
/**
* int32 txexecbulksize = 10;
*/
int getTxexecbulksize();
/**
* int64 totaltriecount = 11;
*/
long getTotaltriecount();
/**
* int64 totaltriesize = 12;
*/
long getTotaltriesize();
/**
* bytes evfsRoot = 13;
*/
com.google.protobuf.ByteString getEvfsRoot();
}
/**
* Protobuf type {@code com.chain.sdk.model.BlockHeader}
*/
public static final class BlockHeader extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.chain.sdk.model.BlockHeader)
BlockHeaderOrBuilder {
private static final long serialVersionUID = 0L;
// Use BlockHeader.newBuilder() to construct.
private BlockHeader(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BlockHeader() {
hash_ = com.google.protobuf.ByteString.EMPTY;
parentHash_ = com.google.protobuf.ByteString.EMPTY;
height_ = 0L;
stateRoot_ = com.google.protobuf.ByteString.EMPTY;
receiptRoot_ = com.google.protobuf.ByteString.EMPTY;
timestamp_ = 0L;
extraData_ = com.google.protobuf.ByteString.EMPTY;
txHashs_ = java.util.Collections.emptyList();
txexecbulkindex_ = java.util.Collections.emptyList();
txexecbulksize_ = 0;
totaltriecount_ = 0L;
totaltriesize_ = 0L;
evfsRoot_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BlockHeader(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
hash_ = input.readBytes();
break;
}
case 18: {
parentHash_ = input.readBytes();
break;
}
case 24: {
height_ = input.readInt64();
break;
}
case 34: {
stateRoot_ = input.readBytes();
break;
}
case 42: {
receiptRoot_ = input.readBytes();
break;
}
case 48: {
timestamp_ = input.readInt64();
break;
}
case 58: {
extraData_ = input.readBytes();
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
txHashs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000080;
}
txHashs_.add(input.readBytes());
break;
}
case 72: {
if (!((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
txexecbulkindex_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000100;
}
txexecbulkindex_.add(input.readInt32());
break;
}
case 74: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000100) == 0x00000100) && input.getBytesUntilLimit() > 0) {
txexecbulkindex_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000100;
}
while (input.getBytesUntilLimit() > 0) {
txexecbulkindex_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
case 80: {
txexecbulksize_ = input.readInt32();
break;
}
case 88: {
totaltriecount_ = input.readInt64();
break;
}
case 96: {
totaltriesize_ = input.readInt64();
break;
}
case 106: {
evfsRoot_ = input.readBytes();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
txHashs_ = java.util.Collections.unmodifiableList(txHashs_);
}
if (((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
txexecbulkindex_ = java.util.Collections.unmodifiableList(txexecbulkindex_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Block.internal_static_com_chain_sdk_model_BlockHeader_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return Block.internal_static_com_chain_sdk_model_BlockHeader_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Block.BlockHeader.class, Block.BlockHeader.Builder.class);
}
private int bitField0_;
public static final int HASH_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString hash_;
/**
* bytes hash = 1;
*/
public com.google.protobuf.ByteString getHash() {
return hash_;
}
public static final int PARENTHASH_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString parentHash_;
/**
* bytes parentHash = 2;
*/
public com.google.protobuf.ByteString getParentHash() {
return parentHash_;
}
public static final int HEIGHT_FIELD_NUMBER = 3;
private long height_;
/**
* int64 height = 3;
*/
public long getHeight() {
return height_;
}
public static final int STATEROOT_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString stateRoot_;
/**
* bytes stateRoot = 4;
*/
public com.google.protobuf.ByteString getStateRoot() {
return stateRoot_;
}
public static final int RECEIPTROOT_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString receiptRoot_;
/**
* bytes receiptRoot = 5;
*/
public com.google.protobuf.ByteString getReceiptRoot() {
return receiptRoot_;
}
public static final int TIMESTAMP_FIELD_NUMBER = 6;
private long timestamp_;
/**
* int64 timestamp = 6;
*/
public long getTimestamp() {
return timestamp_;
}
public static final int EXTRADATA_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString extraData_;
/**
* bytes extraData = 7;
*/
public com.google.protobuf.ByteString getExtraData() {
return extraData_;
}
public static final int TXHASHS_FIELD_NUMBER = 8;
private java.util.List txHashs_;
/**
* repeated bytes txHashs = 8;
*/
public java.util.List
getTxHashsList() {
return txHashs_;
}
/**
* repeated bytes txHashs = 8;
*/
public int getTxHashsCount() {
return txHashs_.size();
}
/**
* repeated bytes txHashs = 8;
*/
public com.google.protobuf.ByteString getTxHashs(int index) {
return txHashs_.get(index);
}
public static final int TXEXECBULKINDEX_FIELD_NUMBER = 9;
private java.util.List txexecbulkindex_;
/**
* repeated int32 txexecbulkindex = 9;
*/
public java.util.List
getTxexecbulkindexList() {
return txexecbulkindex_;
}
/**
* repeated int32 txexecbulkindex = 9;
*/
public int getTxexecbulkindexCount() {
return txexecbulkindex_.size();
}
/**
* repeated int32 txexecbulkindex = 9;
*/
public int getTxexecbulkindex(int index) {
return txexecbulkindex_.get(index);
}
private int txexecbulkindexMemoizedSerializedSize = -1;
public static final int TXEXECBULKSIZE_FIELD_NUMBER = 10;
private int txexecbulksize_;
/**
* int32 txexecbulksize = 10;
*/
public int getTxexecbulksize() {
return txexecbulksize_;
}
public static final int TOTALTRIECOUNT_FIELD_NUMBER = 11;
private long totaltriecount_;
/**
* int64 totaltriecount = 11;
*/
public long getTotaltriecount() {
return totaltriecount_;
}
public static final int TOTALTRIESIZE_FIELD_NUMBER = 12;
private long totaltriesize_;
/**
* int64 totaltriesize = 12;
*/
public long getTotaltriesize() {
return totaltriesize_;
}
public static final int EVFSROOT_FIELD_NUMBER = 13;
private com.google.protobuf.ByteString evfsRoot_;
/**
* bytes evfsRoot = 13;
*/
public com.google.protobuf.ByteString getEvfsRoot() {
return evfsRoot_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (!hash_.isEmpty()) {
output.writeBytes(1, hash_);
}
if (!parentHash_.isEmpty()) {
output.writeBytes(2, parentHash_);
}
if (height_ != 0L) {
output.writeInt64(3, height_);
}
if (!stateRoot_.isEmpty()) {
output.writeBytes(4, stateRoot_);
}
if (!receiptRoot_.isEmpty()) {
output.writeBytes(5, receiptRoot_);
}
if (timestamp_ != 0L) {
output.writeInt64(6, timestamp_);
}
if (!extraData_.isEmpty()) {
output.writeBytes(7, extraData_);
}
for (int i = 0; i < txHashs_.size(); i++) {
output.writeBytes(8, txHashs_.get(i));
}
if (getTxexecbulkindexList().size() > 0) {
output.writeUInt32NoTag(74);
output.writeUInt32NoTag(txexecbulkindexMemoizedSerializedSize);
}
for (int i = 0; i < txexecbulkindex_.size(); i++) {
output.writeInt32NoTag(txexecbulkindex_.get(i));
}
if (txexecbulksize_ != 0) {
output.writeInt32(10, txexecbulksize_);
}
if (totaltriecount_ != 0L) {
output.writeInt64(11, totaltriecount_);
}
if (totaltriesize_ != 0L) {
output.writeInt64(12, totaltriesize_);
}
if (!evfsRoot_.isEmpty()) {
output.writeBytes(13, evfsRoot_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!hash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, hash_);
}
if (!parentHash_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, parentHash_);
}
if (height_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, height_);
}
if (!stateRoot_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, stateRoot_);
}
if (!receiptRoot_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, receiptRoot_);
}
if (timestamp_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(6, timestamp_);
}
if (!extraData_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, extraData_);
}
{
int dataSize = 0;
for (int i = 0; i < txHashs_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(txHashs_.get(i));
}
size += dataSize;
size += 1 * getTxHashsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < txexecbulkindex_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(txexecbulkindex_.get(i));
}
size += dataSize;
if (!getTxexecbulkindexList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
txexecbulkindexMemoizedSerializedSize = dataSize;
}
if (txexecbulksize_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(10, txexecbulksize_);
}
if (totaltriecount_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(11, totaltriecount_);
}
if (totaltriesize_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(12, totaltriesize_);
}
if (!evfsRoot_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(13, evfsRoot_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof Block.BlockHeader)) {
return super.equals(obj);
}
Block.BlockHeader other = (Block.BlockHeader) obj;
boolean result = true;
result = result && getHash()
.equals(other.getHash());
result = result && getParentHash()
.equals(other.getParentHash());
result = result && (getHeight()
== other.getHeight());
result = result && getStateRoot()
.equals(other.getStateRoot());
result = result && getReceiptRoot()
.equals(other.getReceiptRoot());
result = result && (getTimestamp()
== other.getTimestamp());
result = result && getExtraData()
.equals(other.getExtraData());
result = result && getTxHashsList()
.equals(other.getTxHashsList());
result = result && getTxexecbulkindexList()
.equals(other.getTxexecbulkindexList());
result = result && (getTxexecbulksize()
== other.getTxexecbulksize());
result = result && (getTotaltriecount()
== other.getTotaltriecount());
result = result && (getTotaltriesize()
== other.getTotaltriesize());
result = result && getEvfsRoot()
.equals(other.getEvfsRoot());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + HASH_FIELD_NUMBER;
hash = (53 * hash) + getHash().hashCode();
hash = (37 * hash) + PARENTHASH_FIELD_NUMBER;
hash = (53 * hash) + getParentHash().hashCode();
hash = (37 * hash) + HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getHeight());
hash = (37 * hash) + STATEROOT_FIELD_NUMBER;
hash = (53 * hash) + getStateRoot().hashCode();
hash = (37 * hash) + RECEIPTROOT_FIELD_NUMBER;
hash = (53 * hash) + getReceiptRoot().hashCode();
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
hash = (37 * hash) + EXTRADATA_FIELD_NUMBER;
hash = (53 * hash) + getExtraData().hashCode();
if (getTxHashsCount() > 0) {
hash = (37 * hash) + TXHASHS_FIELD_NUMBER;
hash = (53 * hash) + getTxHashsList().hashCode();
}
if (getTxexecbulkindexCount() > 0) {
hash = (37 * hash) + TXEXECBULKINDEX_FIELD_NUMBER;
hash = (53 * hash) + getTxexecbulkindexList().hashCode();
}
hash = (37 * hash) + TXEXECBULKSIZE_FIELD_NUMBER;
hash = (53 * hash) + getTxexecbulksize();
hash = (37 * hash) + TOTALTRIECOUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTotaltriecount());
hash = (37 * hash) + TOTALTRIESIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTotaltriesize());
hash = (37 * hash) + EVFSROOT_FIELD_NUMBER;
hash = (53 * hash) + getEvfsRoot().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static Block.BlockHeader parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Block.BlockHeader parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Block.BlockHeader parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Block.BlockHeader parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Block.BlockHeader parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Block.BlockHeader parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Block.BlockHeader parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static Block.BlockHeader parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static Block.BlockHeader parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static Block.BlockHeader parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static Block.BlockHeader parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static Block.BlockHeader parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(Block.BlockHeader prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.chain.sdk.model.BlockHeader}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.chain.sdk.model.BlockHeader)
Block.BlockHeaderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Block.internal_static_com_chain_sdk_model_BlockHeader_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return Block.internal_static_com_chain_sdk_model_BlockHeader_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Block.BlockHeader.class, Block.BlockHeader.Builder.class);
}
// Construct using com.chain.sdk.model.Block.BlockHeader.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
hash_ = com.google.protobuf.ByteString.EMPTY;
parentHash_ = com.google.protobuf.ByteString.EMPTY;
height_ = 0L;
stateRoot_ = com.google.protobuf.ByteString.EMPTY;
receiptRoot_ = com.google.protobuf.ByteString.EMPTY;
timestamp_ = 0L;
extraData_ = com.google.protobuf.ByteString.EMPTY;
txHashs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
txexecbulkindex_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
txexecbulksize_ = 0;
totaltriecount_ = 0L;
totaltriesize_ = 0L;
evfsRoot_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return Block.internal_static_com_chain_sdk_model_BlockHeader_descriptor;
}
@java.lang.Override
public Block.BlockHeader getDefaultInstanceForType() {
return Block.BlockHeader.getDefaultInstance();
}
@java.lang.Override
public Block.BlockHeader build() {
Block.BlockHeader result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public Block.BlockHeader buildPartial() {
Block.BlockHeader result = new Block.BlockHeader(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.hash_ = hash_;
result.parentHash_ = parentHash_;
result.height_ = height_;
result.stateRoot_ = stateRoot_;
result.receiptRoot_ = receiptRoot_;
result.timestamp_ = timestamp_;
result.extraData_ = extraData_;
if (((bitField0_ & 0x00000080) == 0x00000080)) {
txHashs_ = java.util.Collections.unmodifiableList(txHashs_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.txHashs_ = txHashs_;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
txexecbulkindex_ = java.util.Collections.unmodifiableList(txexecbulkindex_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.txexecbulkindex_ = txexecbulkindex_;
result.txexecbulksize_ = txexecbulksize_;
result.totaltriecount_ = totaltriecount_;
result.totaltriesize_ = totaltriesize_;
result.evfsRoot_ = evfsRoot_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Block.BlockHeader) {
return mergeFrom((Block.BlockHeader)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Block.BlockHeader other) {
if (other == Block.BlockHeader.getDefaultInstance()) return this;
if (other.getHash() != com.google.protobuf.ByteString.EMPTY) {
setHash(other.getHash());
}
if (other.getParentHash() != com.google.protobuf.ByteString.EMPTY) {
setParentHash(other.getParentHash());
}
if (other.getHeight() != 0L) {
setHeight(other.getHeight());
}
if (other.getStateRoot() != com.google.protobuf.ByteString.EMPTY) {
setStateRoot(other.getStateRoot());
}
if (other.getReceiptRoot() != com.google.protobuf.ByteString.EMPTY) {
setReceiptRoot(other.getReceiptRoot());
}
if (other.getTimestamp() != 0L) {
setTimestamp(other.getTimestamp());
}
if (other.getExtraData() != com.google.protobuf.ByteString.EMPTY) {
setExtraData(other.getExtraData());
}
if (!other.txHashs_.isEmpty()) {
if (txHashs_.isEmpty()) {
txHashs_ = other.txHashs_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureTxHashsIsMutable();
txHashs_.addAll(other.txHashs_);
}
onChanged();
}
if (!other.txexecbulkindex_.isEmpty()) {
if (txexecbulkindex_.isEmpty()) {
txexecbulkindex_ = other.txexecbulkindex_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureTxexecbulkindexIsMutable();
txexecbulkindex_.addAll(other.txexecbulkindex_);
}
onChanged();
}
if (other.getTxexecbulksize() != 0) {
setTxexecbulksize(other.getTxexecbulksize());
}
if (other.getTotaltriecount() != 0L) {
setTotaltriecount(other.getTotaltriecount());
}
if (other.getTotaltriesize() != 0L) {
setTotaltriesize(other.getTotaltriesize());
}
if (other.getEvfsRoot() != com.google.protobuf.ByteString.EMPTY) {
setEvfsRoot(other.getEvfsRoot());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Block.BlockHeader parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Block.BlockHeader) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString hash_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes hash = 1;
*/
public com.google.protobuf.ByteString getHash() {
return hash_;
}
/**
* bytes hash = 1;
*/
public Builder setHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
hash_ = value;
onChanged();
return this;
}
/**
* bytes hash = 1;
*/
public Builder clearHash() {
hash_ = getDefaultInstance().getHash();
onChanged();
return this;
}
private com.google.protobuf.ByteString parentHash_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes parentHash = 2;
*/
public com.google.protobuf.ByteString getParentHash() {
return parentHash_;
}
/**
* bytes parentHash = 2;
*/
public Builder setParentHash(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
parentHash_ = value;
onChanged();
return this;
}
/**
* bytes parentHash = 2;
*/
public Builder clearParentHash() {
parentHash_ = getDefaultInstance().getParentHash();
onChanged();
return this;
}
private long height_ ;
/**
* int64 height = 3;
*/
public long getHeight() {
return height_;
}
/**
* int64 height = 3;
*/
public Builder setHeight(long value) {
height_ = value;
onChanged();
return this;
}
/**
* int64 height = 3;
*/
public Builder clearHeight() {
height_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString stateRoot_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes stateRoot = 4;
*/
public com.google.protobuf.ByteString getStateRoot() {
return stateRoot_;
}
/**
* bytes stateRoot = 4;
*/
public Builder setStateRoot(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
stateRoot_ = value;
onChanged();
return this;
}
/**
* bytes stateRoot = 4;
*/
public Builder clearStateRoot() {
stateRoot_ = getDefaultInstance().getStateRoot();
onChanged();
return this;
}
private com.google.protobuf.ByteString receiptRoot_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes receiptRoot = 5;
*/
public com.google.protobuf.ByteString getReceiptRoot() {
return receiptRoot_;
}
/**
* bytes receiptRoot = 5;
*/
public Builder setReceiptRoot(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
receiptRoot_ = value;
onChanged();
return this;
}
/**
* bytes receiptRoot = 5;
*/
public Builder clearReceiptRoot() {
receiptRoot_ = getDefaultInstance().getReceiptRoot();
onChanged();
return this;
}
private long timestamp_ ;
/**
* int64 timestamp = 6;
*/
public long getTimestamp() {
return timestamp_;
}
/**
* int64 timestamp = 6;
*/
public Builder setTimestamp(long value) {
timestamp_ = value;
onChanged();
return this;
}
/**
* int64 timestamp = 6;
*/
public Builder clearTimestamp() {
timestamp_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString extraData_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes extraData = 7;
*/
public com.google.protobuf.ByteString getExtraData() {
return extraData_;
}
/**
* bytes extraData = 7;
*/
public Builder setExtraData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
extraData_ = value;
onChanged();
return this;
}
/**
* bytes extraData = 7;
*/
public Builder clearExtraData() {
extraData_ = getDefaultInstance().getExtraData();
onChanged();
return this;
}
private java.util.List txHashs_ = java.util.Collections.emptyList();
private void ensureTxHashsIsMutable() {
if (!((bitField0_ & 0x00000080) == 0x00000080)) {
txHashs_ = new java.util.ArrayList(txHashs_);
bitField0_ |= 0x00000080;
}
}
/**
* repeated bytes txHashs = 8;
*/
public java.util.List
getTxHashsList() {
return java.util.Collections.unmodifiableList(txHashs_);
}
/**
* repeated bytes txHashs = 8;
*/
public int getTxHashsCount() {
return txHashs_.size();
}
/**
* repeated bytes txHashs = 8;
*/
public com.google.protobuf.ByteString getTxHashs(int index) {
return txHashs_.get(index);
}
/**
* repeated bytes txHashs = 8;
*/
public Builder setTxHashs(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureTxHashsIsMutable();
txHashs_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes txHashs = 8;
*/
public Builder addTxHashs(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureTxHashsIsMutable();
txHashs_.add(value);
onChanged();
return this;
}
/**
* repeated bytes txHashs = 8;
*/
public Builder addAllTxHashs(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureTxHashsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, txHashs_);
onChanged();
return this;
}
/**
* repeated bytes txHashs = 8;
*/
public Builder clearTxHashs() {
txHashs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
private java.util.List txexecbulkindex_ = java.util.Collections.emptyList();
private void ensureTxexecbulkindexIsMutable() {
if (!((bitField0_ & 0x00000100) == 0x00000100)) {
txexecbulkindex_ = new java.util.ArrayList(txexecbulkindex_);
bitField0_ |= 0x00000100;
}
}
/**
* repeated int32 txexecbulkindex = 9;
*/
public java.util.List
getTxexecbulkindexList() {
return java.util.Collections.unmodifiableList(txexecbulkindex_);
}
/**
* repeated int32 txexecbulkindex = 9;
*/
public int getTxexecbulkindexCount() {
return txexecbulkindex_.size();
}
/**
* repeated int32 txexecbulkindex = 9;
*/
public int getTxexecbulkindex(int index) {
return txexecbulkindex_.get(index);
}
/**
* repeated int32 txexecbulkindex = 9;
*/
public Builder setTxexecbulkindex(
int index, int value) {
ensureTxexecbulkindexIsMutable();
txexecbulkindex_.set(index, value);
onChanged();
return this;
}
/**
* repeated int32 txexecbulkindex = 9;
*/
public Builder addTxexecbulkindex(int value) {
ensureTxexecbulkindexIsMutable();
txexecbulkindex_.add(value);
onChanged();
return this;
}
/**
* repeated int32 txexecbulkindex = 9;
*/
public Builder addAllTxexecbulkindex(
java.lang.Iterable extends java.lang.Integer> values) {
ensureTxexecbulkindexIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, txexecbulkindex_);
onChanged();
return this;
}
/**
* repeated int32 txexecbulkindex = 9;
*/
public Builder clearTxexecbulkindex() {
txexecbulkindex_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
private int txexecbulksize_ ;
/**
* int32 txexecbulksize = 10;
*/
public int getTxexecbulksize() {
return txexecbulksize_;
}
/**
* int32 txexecbulksize = 10;
*/
public Builder setTxexecbulksize(int value) {
txexecbulksize_ = value;
onChanged();
return this;
}
/**
* int32 txexecbulksize = 10;
*/
public Builder clearTxexecbulksize() {
txexecbulksize_ = 0;
onChanged();
return this;
}
private long totaltriecount_ ;
/**
* int64 totaltriecount = 11;
*/
public long getTotaltriecount() {
return totaltriecount_;
}
/**
* int64 totaltriecount = 11;
*/
public Builder setTotaltriecount(long value) {
totaltriecount_ = value;
onChanged();
return this;
}
/**
* int64 totaltriecount = 11;
*/
public Builder clearTotaltriecount() {
totaltriecount_ = 0L;
onChanged();
return this;
}
private long totaltriesize_ ;
/**
* int64 totaltriesize = 12;
*/
public long getTotaltriesize() {
return totaltriesize_;
}
/**
* int64 totaltriesize = 12;
*/
public Builder setTotaltriesize(long value) {
totaltriesize_ = value;
onChanged();
return this;
}
/**
* int64 totaltriesize = 12;
*/
public Builder clearTotaltriesize() {
totaltriesize_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString evfsRoot_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes evfsRoot = 13;
*/
public com.google.protobuf.ByteString getEvfsRoot() {
return evfsRoot_;
}
/**
* bytes evfsRoot = 13;
*/
public Builder setEvfsRoot(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
evfsRoot_ = value;
onChanged();
return this;
}
/**
* bytes evfsRoot = 13;
*/
public Builder clearEvfsRoot() {
evfsRoot_ = getDefaultInstance().getEvfsRoot();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.chain.sdk.model.BlockHeader)
}
// @@protoc_insertion_point(class_scope:com.chain.sdk.model.BlockHeader)
private static final Block.BlockHeader DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new Block.BlockHeader();
}
public static Block.BlockHeader getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BlockHeader parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BlockHeader(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public Block.BlockHeader getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BlockBodyOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.chain.sdk.model.BlockBody)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
java.util.List
getTxsList();
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
Transaction.TransactionInfo getTxs(int index);
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
int getTxsCount();
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
java.util.List extends Transaction.TransactionInfoOrBuilder>
getTxsOrBuilderList();
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
Transaction.TransactionInfoOrBuilder getTxsOrBuilder(
int index);
}
/**
* Protobuf type {@code com.chain.sdk.model.BlockBody}
*/
public static final class BlockBody extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.chain.sdk.model.BlockBody)
BlockBodyOrBuilder {
private static final long serialVersionUID = 0L;
// Use BlockBody.newBuilder() to construct.
private BlockBody(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BlockBody() {
txs_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BlockBody(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
txs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
txs_.add(
input.readMessage(Transaction.TransactionInfo.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
txs_ = java.util.Collections.unmodifiableList(txs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Block.internal_static_com_chain_sdk_model_BlockBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return Block.internal_static_com_chain_sdk_model_BlockBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Block.BlockBody.class, Block.BlockBody.Builder.class);
}
public static final int TXS_FIELD_NUMBER = 1;
private java.util.List txs_;
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public java.util.List getTxsList() {
return txs_;
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public java.util.List extends Transaction.TransactionInfoOrBuilder>
getTxsOrBuilderList() {
return txs_;
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public int getTxsCount() {
return txs_.size();
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Transaction.TransactionInfo getTxs(int index) {
return txs_.get(index);
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Transaction.TransactionInfoOrBuilder getTxsOrBuilder(
int index) {
return txs_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < txs_.size(); i++) {
output.writeMessage(1, txs_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < txs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, txs_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof Block.BlockBody)) {
return super.equals(obj);
}
Block.BlockBody other = (Block.BlockBody) obj;
boolean result = true;
result = result && getTxsList()
.equals(other.getTxsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getTxsCount() > 0) {
hash = (37 * hash) + TXS_FIELD_NUMBER;
hash = (53 * hash) + getTxsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static Block.BlockBody parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Block.BlockBody parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Block.BlockBody parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Block.BlockBody parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Block.BlockBody parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Block.BlockBody parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Block.BlockBody parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static Block.BlockBody parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static Block.BlockBody parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static Block.BlockBody parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static Block.BlockBody parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static Block.BlockBody parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(Block.BlockBody prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.chain.sdk.model.BlockBody}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.chain.sdk.model.BlockBody)
Block.BlockBodyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Block.internal_static_com_chain_sdk_model_BlockBody_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return Block.internal_static_com_chain_sdk_model_BlockBody_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Block.BlockBody.class, Block.BlockBody.Builder.class);
}
// Construct using com.chain.sdk.model.Block.BlockBody.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTxsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (txsBuilder_ == null) {
txs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
txsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return Block.internal_static_com_chain_sdk_model_BlockBody_descriptor;
}
@java.lang.Override
public Block.BlockBody getDefaultInstanceForType() {
return Block.BlockBody.getDefaultInstance();
}
@java.lang.Override
public Block.BlockBody build() {
Block.BlockBody result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public Block.BlockBody buildPartial() {
Block.BlockBody result = new Block.BlockBody(this);
int from_bitField0_ = bitField0_;
if (txsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
txs_ = java.util.Collections.unmodifiableList(txs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.txs_ = txs_;
} else {
result.txs_ = txsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Block.BlockBody) {
return mergeFrom((Block.BlockBody)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Block.BlockBody other) {
if (other == Block.BlockBody.getDefaultInstance()) return this;
if (txsBuilder_ == null) {
if (!other.txs_.isEmpty()) {
if (txs_.isEmpty()) {
txs_ = other.txs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTxsIsMutable();
txs_.addAll(other.txs_);
}
onChanged();
}
} else {
if (!other.txs_.isEmpty()) {
if (txsBuilder_.isEmpty()) {
txsBuilder_.dispose();
txsBuilder_ = null;
txs_ = other.txs_;
bitField0_ = (bitField0_ & ~0x00000001);
txsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTxsFieldBuilder() : null;
} else {
txsBuilder_.addAllMessages(other.txs_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Block.BlockBody parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Block.BlockBody) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List txs_ =
java.util.Collections.emptyList();
private void ensureTxsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
txs_ = new java.util.ArrayList(txs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
Transaction.TransactionInfo, Transaction.TransactionInfo.Builder, Transaction.TransactionInfoOrBuilder> txsBuilder_;
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public java.util.List getTxsList() {
if (txsBuilder_ == null) {
return java.util.Collections.unmodifiableList(txs_);
} else {
return txsBuilder_.getMessageList();
}
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public int getTxsCount() {
if (txsBuilder_ == null) {
return txs_.size();
} else {
return txsBuilder_.getCount();
}
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Transaction.TransactionInfo getTxs(int index) {
if (txsBuilder_ == null) {
return txs_.get(index);
} else {
return txsBuilder_.getMessage(index);
}
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Builder setTxs(
int index, Transaction.TransactionInfo value) {
if (txsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTxsIsMutable();
txs_.set(index, value);
onChanged();
} else {
txsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Builder setTxs(
int index, Transaction.TransactionInfo.Builder builderForValue) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
txs_.set(index, builderForValue.build());
onChanged();
} else {
txsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Builder addTxs(Transaction.TransactionInfo value) {
if (txsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTxsIsMutable();
txs_.add(value);
onChanged();
} else {
txsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Builder addTxs(
int index, Transaction.TransactionInfo value) {
if (txsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTxsIsMutable();
txs_.add(index, value);
onChanged();
} else {
txsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Builder addTxs(
Transaction.TransactionInfo.Builder builderForValue) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
txs_.add(builderForValue.build());
onChanged();
} else {
txsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Builder addTxs(
int index, Transaction.TransactionInfo.Builder builderForValue) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
txs_.add(index, builderForValue.build());
onChanged();
} else {
txsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Builder addAllTxs(
java.lang.Iterable extends Transaction.TransactionInfo> values) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, txs_);
onChanged();
} else {
txsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Builder clearTxs() {
if (txsBuilder_ == null) {
txs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
txsBuilder_.clear();
}
return this;
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Builder removeTxs(int index) {
if (txsBuilder_ == null) {
ensureTxsIsMutable();
txs_.remove(index);
onChanged();
} else {
txsBuilder_.remove(index);
}
return this;
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Transaction.TransactionInfo.Builder getTxsBuilder(
int index) {
return getTxsFieldBuilder().getBuilder(index);
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Transaction.TransactionInfoOrBuilder getTxsOrBuilder(
int index) {
if (txsBuilder_ == null) {
return txs_.get(index); } else {
return txsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public java.util.List extends Transaction.TransactionInfoOrBuilder>
getTxsOrBuilderList() {
if (txsBuilder_ != null) {
return txsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(txs_);
}
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Transaction.TransactionInfo.Builder addTxsBuilder() {
return getTxsFieldBuilder().addBuilder(
Transaction.TransactionInfo.getDefaultInstance());
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public Transaction.TransactionInfo.Builder addTxsBuilder(
int index) {
return getTxsFieldBuilder().addBuilder(
index, Transaction.TransactionInfo.getDefaultInstance());
}
/**
* repeated .com.chain.sdk.model.TransactionInfo txs = 1;
*/
public java.util.List
getTxsBuilderList() {
return getTxsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
Transaction.TransactionInfo, Transaction.TransactionInfo.Builder, Transaction.TransactionInfoOrBuilder>
getTxsFieldBuilder() {
if (txsBuilder_ == null) {
txsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
Transaction.TransactionInfo, Transaction.TransactionInfo.Builder, Transaction.TransactionInfoOrBuilder>(
txs_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
txs_ = null;
}
return txsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.chain.sdk.model.BlockBody)
}
// @@protoc_insertion_point(class_scope:com.chain.sdk.model.BlockBody)
private static final Block.BlockBody DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new Block.BlockBody();
}
public static Block.BlockBody getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BlockBody parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BlockBody(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public Block.BlockBody getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BlockMinerOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.chain.sdk.model.BlockMiner)
com.google.protobuf.MessageOrBuilder {
/**
* bytes address = 1;
*/
com.google.protobuf.ByteString getAddress();
/**
* bytes reward = 2;
*/
com.google.protobuf.ByteString getReward();
/**
* string nid = 3;
*/
java.lang.String getNid();
/**
* string nid = 3;
*/
com.google.protobuf.ByteString
getNidBytes();
/**
* string term = 4;
*/
java.lang.String getTerm();
/**
* string term = 4;
*/
com.google.protobuf.ByteString
getTermBytes();
/**
* string bits = 5;
*/
java.lang.String getBits();
/**
* string bits = 5;
*/
com.google.protobuf.ByteString
getBitsBytes();
}
/**
* Protobuf type {@code com.chain.sdk.model.BlockMiner}
*/
public static final class BlockMiner extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.chain.sdk.model.BlockMiner)
BlockMinerOrBuilder {
private static final long serialVersionUID = 0L;
// Use BlockMiner.newBuilder() to construct.
private BlockMiner(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BlockMiner() {
address_ = com.google.protobuf.ByteString.EMPTY;
reward_ = com.google.protobuf.ByteString.EMPTY;
nid_ = "";
term_ = "";
bits_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BlockMiner(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
address_ = input.readBytes();
break;
}
case 18: {
reward_ = input.readBytes();
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
nid_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
term_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
bits_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Block.internal_static_com_chain_sdk_model_BlockMiner_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return Block.internal_static_com_chain_sdk_model_BlockMiner_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Block.BlockMiner.class, Block.BlockMiner.Builder.class);
}
public static final int ADDRESS_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString address_;
/**
* bytes address = 1;
*/
public com.google.protobuf.ByteString getAddress() {
return address_;
}
public static final int REWARD_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString reward_;
/**
* bytes reward = 2;
*/
public com.google.protobuf.ByteString getReward() {
return reward_;
}
public static final int NID_FIELD_NUMBER = 3;
private volatile java.lang.Object nid_;
/**
* string nid = 3;
*/
public java.lang.String getNid() {
java.lang.Object ref = nid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nid_ = s;
return s;
}
}
/**
* string nid = 3;
*/
public com.google.protobuf.ByteString
getNidBytes() {
java.lang.Object ref = nid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TERM_FIELD_NUMBER = 4;
private volatile java.lang.Object term_;
/**
* string term = 4;
*/
public java.lang.String getTerm() {
java.lang.Object ref = term_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
term_ = s;
return s;
}
}
/**
* string term = 4;
*/
public com.google.protobuf.ByteString
getTermBytes() {
java.lang.Object ref = term_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
term_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BITS_FIELD_NUMBER = 5;
private volatile java.lang.Object bits_;
/**
* string bits = 5;
*/
public java.lang.String getBits() {
java.lang.Object ref = bits_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
bits_ = s;
return s;
}
}
/**
* string bits = 5;
*/
public com.google.protobuf.ByteString
getBitsBytes() {
java.lang.Object ref = bits_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
bits_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!address_.isEmpty()) {
output.writeBytes(1, address_);
}
if (!reward_.isEmpty()) {
output.writeBytes(2, reward_);
}
if (!getNidBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, nid_);
}
if (!getTermBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, term_);
}
if (!getBitsBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, bits_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!address_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, address_);
}
if (!reward_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, reward_);
}
if (!getNidBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, nid_);
}
if (!getTermBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, term_);
}
if (!getBitsBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, bits_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof Block.BlockMiner)) {
return super.equals(obj);
}
Block.BlockMiner other = (Block.BlockMiner) obj;
boolean result = true;
result = result && getAddress()
.equals(other.getAddress());
result = result && getReward()
.equals(other.getReward());
result = result && getNid()
.equals(other.getNid());
result = result && getTerm()
.equals(other.getTerm());
result = result && getBits()
.equals(other.getBits());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress().hashCode();
hash = (37 * hash) + REWARD_FIELD_NUMBER;
hash = (53 * hash) + getReward().hashCode();
hash = (37 * hash) + NID_FIELD_NUMBER;
hash = (53 * hash) + getNid().hashCode();
hash = (37 * hash) + TERM_FIELD_NUMBER;
hash = (53 * hash) + getTerm().hashCode();
hash = (37 * hash) + BITS_FIELD_NUMBER;
hash = (53 * hash) + getBits().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static Block.BlockMiner parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Block.BlockMiner parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Block.BlockMiner parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Block.BlockMiner parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Block.BlockMiner parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static Block.BlockMiner parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static Block.BlockMiner parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static Block.BlockMiner parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static Block.BlockMiner parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static Block.BlockMiner parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static Block.BlockMiner parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static Block.BlockMiner parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(Block.BlockMiner prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.chain.sdk.model.BlockMiner}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.chain.sdk.model.BlockMiner)
Block.BlockMinerOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return Block.internal_static_com_chain_sdk_model_BlockMiner_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return Block.internal_static_com_chain_sdk_model_BlockMiner_fieldAccessorTable
.ensureFieldAccessorsInitialized(
Block.BlockMiner.class, Block.BlockMiner.Builder.class);
}
// Construct using com.chain.sdk.model.Block.BlockMiner.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
address_ = com.google.protobuf.ByteString.EMPTY;
reward_ = com.google.protobuf.ByteString.EMPTY;
nid_ = "";
term_ = "";
bits_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return Block.internal_static_com_chain_sdk_model_BlockMiner_descriptor;
}
@java.lang.Override
public Block.BlockMiner getDefaultInstanceForType() {
return Block.BlockMiner.getDefaultInstance();
}
@java.lang.Override
public Block.BlockMiner build() {
Block.BlockMiner result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public Block.BlockMiner buildPartial() {
Block.BlockMiner result = new Block.BlockMiner(this);
result.address_ = address_;
result.reward_ = reward_;
result.nid_ = nid_;
result.term_ = term_;
result.bits_ = bits_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof Block.BlockMiner) {
return mergeFrom((Block.BlockMiner)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(Block.BlockMiner other) {
if (other == Block.BlockMiner.getDefaultInstance()) return this;
if (other.getAddress() != com.google.protobuf.ByteString.EMPTY) {
setAddress(other.getAddress());
}
if (other.getReward() != com.google.protobuf.ByteString.EMPTY) {
setReward(other.getReward());
}
if (!other.getNid().isEmpty()) {
nid_ = other.nid_;
onChanged();
}
if (!other.getTerm().isEmpty()) {
term_ = other.term_;
onChanged();
}
if (!other.getBits().isEmpty()) {
bits_ = other.bits_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
Block.BlockMiner parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (Block.BlockMiner) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString address_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes address = 1;
*/
public com.google.protobuf.ByteString getAddress() {
return address_;
}
/**
* bytes address = 1;
*/
public Builder setAddress(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
address_ = value;
onChanged();
return this;
}
/**
* bytes address = 1;
*/
public Builder clearAddress() {
address_ = getDefaultInstance().getAddress();
onChanged();
return this;
}
private com.google.protobuf.ByteString reward_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes reward = 2;
*/
public com.google.protobuf.ByteString getReward() {
return reward_;
}
/**
* bytes reward = 2;
*/
public Builder setReward(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
reward_ = value;
onChanged();
return this;
}
/**
* bytes reward = 2;
*/
public Builder clearReward() {
reward_ = getDefaultInstance().getReward();
onChanged();
return this;
}
private java.lang.Object nid_ = "";
/**
* string nid = 3;
*/
public java.lang.String getNid() {
java.lang.Object ref = nid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string nid = 3;
*/
public com.google.protobuf.ByteString
getNidBytes() {
java.lang.Object ref = nid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string nid = 3;
*/
public Builder setNid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
nid_ = value;
onChanged();
return this;
}
/**
* string nid = 3;
*/
public Builder clearNid() {
nid_ = getDefaultInstance().getNid();
onChanged();
return this;
}
/**
* string nid = 3;
*/
public Builder setNidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
nid_ = value;
onChanged();
return this;
}
private java.lang.Object term_ = "";
/**
* string term = 4;
*/
public java.lang.String getTerm() {
java.lang.Object ref = term_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
term_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string term = 4;
*/
public com.google.protobuf.ByteString
getTermBytes() {
java.lang.Object ref = term_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
term_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string term = 4;
*/
public Builder setTerm(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
term_ = value;
onChanged();
return this;
}
/**
* string term = 4;
*/
public Builder clearTerm() {
term_ = getDefaultInstance().getTerm();
onChanged();
return this;
}
/**
* string term = 4;
*/
public Builder setTermBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
term_ = value;
onChanged();
return this;
}
private java.lang.Object bits_ = "";
/**
* string bits = 5;
*/
public java.lang.String getBits() {
java.lang.Object ref = bits_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
bits_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string bits = 5;
*/
public com.google.protobuf.ByteString
getBitsBytes() {
java.lang.Object ref = bits_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
bits_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string bits = 5;
*/
public Builder setBits(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bits_ = value;
onChanged();
return this;
}
/**
* string bits = 5;
*/
public Builder clearBits() {
bits_ = getDefaultInstance().getBits();
onChanged();
return this;
}
/**
* string bits = 5;
*/
public Builder setBitsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bits_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.chain.sdk.model.BlockMiner)
}
// @@protoc_insertion_point(class_scope:com.chain.sdk.model.BlockMiner)
private static final Block.BlockMiner DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new Block.BlockMiner();
}
public static Block.BlockMiner getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BlockMiner parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BlockMiner(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public Block.BlockMiner getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_chain_sdk_model_BlockInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_chain_sdk_model_BlockInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_chain_sdk_model_BlockHeader_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_chain_sdk_model_BlockHeader_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_chain_sdk_model_BlockBody_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_chain_sdk_model_BlockBody_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_chain_sdk_model_BlockMiner_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_chain_sdk_model_BlockMiner_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\013block.proto\022\023com.chain.sdk.model\032\021tran" +
"saction.proto\"\254\001\n\tBlockInfo\0220\n\006header\030\001 " +
"\001(\0132 .com.chain.sdk.model.BlockHeader\022,\n" +
"\004body\030\002 \001(\0132\036.com.chain.sdk.model.BlockB" +
"ody\022.\n\005miner\030\003 \001(\0132\037.com.chain.sdk.model" +
".BlockMiner\022\017\n\007version\030\004 \001(\005\"\220\002\n\013BlockHe" +
"ader\022\014\n\004hash\030\001 \001(\014\022\022\n\nparentHash\030\002 \001(\014\022\016" +
"\n\006height\030\003 \001(\003\022\021\n\tstateRoot\030\004 \001(\014\022\023\n\013rec" +
"eiptRoot\030\005 \001(\014\022\021\n\ttimestamp\030\006 \001(\003\022\021\n\text" +
"raData\030\007 \001(\014\022\017\n\007txHashs\030\010 \003(\014\022\027\n\017txexecb" +
"ulkindex\030\t \003(\005\022\026\n\016txexecbulksize\030\n \001(\005\022\026" +
"\n\016totaltriecount\030\013 \001(\003\022\025\n\rtotaltriesize\030" +
"\014 \001(\003\022\020\n\010evfsRoot\030\r \001(\014\">\n\tBlockBody\0221\n\003" +
"txs\030\001 \003(\0132$.com.chain.sdk.model.Transact" +
"ionInfo\"V\n\nBlockMiner\022\017\n\007address\030\001 \001(\014\022\016" +
"\n\006reward\030\002 \001(\014\022\013\n\003nid\030\003 \001(\t\022\014\n\004term\030\004 \001(" +
"\t\022\014\n\004bits\030\005 \001(\tb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
Transaction.getDescriptor(),
}, assigner);
internal_static_com_chain_sdk_model_BlockInfo_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_com_chain_sdk_model_BlockInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_chain_sdk_model_BlockInfo_descriptor,
new java.lang.String[] { "Header", "Body", "Miner", "Version", });
internal_static_com_chain_sdk_model_BlockHeader_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_com_chain_sdk_model_BlockHeader_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_chain_sdk_model_BlockHeader_descriptor,
new java.lang.String[] { "Hash", "ParentHash", "Height", "StateRoot", "ReceiptRoot", "Timestamp", "ExtraData", "TxHashs", "Txexecbulkindex", "Txexecbulksize", "Totaltriecount", "Totaltriesize", "EvfsRoot", });
internal_static_com_chain_sdk_model_BlockBody_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_com_chain_sdk_model_BlockBody_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_chain_sdk_model_BlockBody_descriptor,
new java.lang.String[] { "Txs", });
internal_static_com_chain_sdk_model_BlockMiner_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_com_chain_sdk_model_BlockMiner_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_chain_sdk_model_BlockMiner_descriptor,
new java.lang.String[] { "Address", "Reward", "Nid", "Term", "Bits", });
Transaction.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy