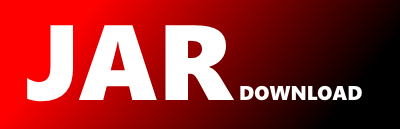
repicea.io.javadbf.DBFField Maven / Gradle / Ivy
/*
DBFField
Class represents a "field" (or column) definition of a DBF data structure.
This file is part of JavaDBF package.
author: [email protected]
license: LGPL (http://www.gnu.org/copyleft/lesser.html)
$Id: DBFField.java,v 1.7 2004/03/31 10:50:11 anil Exp $
*/
package repicea.io.javadbf;
import java.io.DataInput;
import java.io.DataOutput;
import java.io.IOException;
import repicea.io.FormatField;
/**
DBFField represents a field specification in an dbf file.
DBFField objects are either created and added to a DBFWriter object or obtained
from DBFReader object through getField( int) query.
*/
public class DBFField extends FormatField {
public static final byte FIELD_TYPE_C = (byte)'C';
public static final byte FIELD_TYPE_L = (byte)'L';
public static final byte FIELD_TYPE_N = (byte)'N';
public static final byte FIELD_TYPE_F = (byte)'F';
public static final byte FIELD_TYPE_D = (byte)'D';
public static final byte FIELD_TYPE_M = (byte)'M';
/* Field struct variables start here */
byte[] fieldName = new byte[ 11]; /* 0-10*/
byte dataType; /* 11 */
int reserv1; /* 12-15 */
int fieldLength; /* 16 */
byte decimalCount; /* 17 */
short reserv2; /* 18-19 */
byte workAreaId; /* 20 */
short reserv3; /* 21-22 */
byte setFieldsFlag; /* 23 */
byte[] reserv4 = new byte[ 7]; /* 24-30 */
byte indexFieldFlag; /* 31 */
/* Field struct variables end here */
/* other class variables */
int nameNullIndex = 0;
/**
Creates a DBFField object from the data read from the given DataInputStream.
The data in the DataInputStream object is supposed to be organised correctly
and the stream "pointer" is supposed to be positioned properly.
@param in DataInputStream
@return Returns the created DBFField object.
@throws IOException If any stream reading problems occures.
*/
protected static DBFField createField(DataInput in) throws IOException {
DBFField field = new DBFField();
byte t_byte = in.readByte(); /* 0 */
if( t_byte == (byte)0x0d) {
//System.out.println( "End of header found");
return null;
}
in.readFully( field.fieldName, 1, 10); /* 1-10 */
field.fieldName[0] = t_byte;
for( int i=0; i 10) {
value = value.substring(0, 10);
}
this.fieldName = value.getBytes();
this.nameNullIndex = this.fieldName.length;
}
/**
* Sets the data type of the field.
* @param value type of the field. One of the following:
* C, L, N, F, D, M
*/
public void setDataType( byte value) {
switch( value) {
case 'D':
this.fieldLength = 8; /* fall through */
case 'C':
case 'L':
case 'N':
case 'F':
case 'M':
this.dataType = value;
break;
default:
throw new IllegalArgumentException( "Unknown data type");
}
}
/**
* Length of the field.
* This method should be called before calling setDecimalCount().
* @param value length of the field as int.
*/
public void setFieldLength(int value) {
if( value <= 0) {
throw new IllegalArgumentException( "Field length should be a positive number");
}
if( this.dataType == FIELD_TYPE_D) {
throw new UnsupportedOperationException( "Cannot do this on a Date field");
}
fieldLength = value;
}
/**
* Sets the decimal place size of the field.
* Before calling this method the size of the field
* should be set by calling setFieldLength().
* @param value the size of the decimal field.
*/
public void setDecimalCount(int value) {
if( value < 0) {
throw new IllegalArgumentException( "Decimal length should be a positive number");
}
if( value > fieldLength) {
throw new IllegalArgumentException( "Decimal length should be less than field length");
}
decimalCount = (byte)value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy