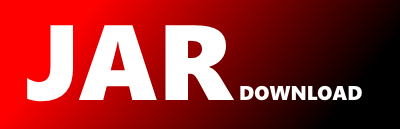
io.swagger.models.parameters.AbstractSerializableParameter Maven / Gradle / Ivy
package io.swagger.models.parameters;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import io.swagger.models.properties.ArrayProperty;
import io.swagger.models.properties.BaseIntegerProperty;
import io.swagger.models.properties.BooleanProperty;
import io.swagger.models.properties.DecimalProperty;
import io.swagger.models.properties.DoubleProperty;
import io.swagger.models.properties.FloatProperty;
import io.swagger.models.properties.IntegerProperty;
import io.swagger.models.properties.LongProperty;
import io.swagger.models.properties.Property;
import io.swagger.models.properties.StringProperty;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
@JsonPropertyOrder({ "name", "in", "description", "required", "type", "items", "collectionFormat", "default", "maximum",
"exclusiveMaximum", "minimum", "exclusiveMinimum", "maxLength", "minLength", "pattern", "maxItems", "minItems",
"uniqueItems", "multipleOf" })
public abstract class AbstractSerializableParameter> extends AbstractParameter implements SerializableParameter {
private static final Logger LOGGER = LoggerFactory.getLogger(AbstractSerializableParameter.class);
protected String type;
protected String format;
protected String collectionFormat;
protected Property items;
protected Boolean exclusiveMaximum;
protected BigDecimal maximum;
protected Boolean exclusiveMinimum;
protected BigDecimal minimum;
protected String example;
private Integer maxItems;
private Integer minItems;
protected Boolean allowEmptyValue;
@JsonIgnore
protected List _enum;
/**
* See http://json-schema.org/latest/json-schema-validation.html#anchor26
*/
public Integer maxLength;
/**
* See http://json-schema.org/latest/json-schema-validation.html#anchor29
*/
public Integer minLength;
/**
* See http://json-schema.org/latest/json-schema-validation.html#anchor33
*/
public String pattern;
/**
* See http://json-schema.org/latest/json-schema-validation.html#anchor49
*/
public Boolean uniqueItems;
/**
* See http://json-schema.org/latest/json-schema-validation.html#anchor14
*/
public Number multipleOf;
@JsonIgnore
protected String defaultValue;
public T property(Property property) {
this.setProperty(property);
return castThis();
}
public T type(String type) {
this.setType(type);
return castThis();
}
public T format(String format) {
this.setFormat(format);
return castThis();
}
public T description(String description) {
this.setDescription(description);
return castThis();
}
public T name(String name) {
this.setName(name);
return castThis();
}
public T required(boolean required) {
this.setRequired(required);
return castThis();
}
public T collectionFormat(String collectionFormat) {
this.setCollectionFormat(collectionFormat);
return castThis();
}
public T example(String example) {
this.setExample(example);
return castThis();
}
public T allowEmptyValue(Boolean allowEmpty) {
this.setAllowEmptyValue(allowEmpty);
return castThis();
}
public T readOnly(Boolean readOnly) {
this.setReadOnly(readOnly);
return castThis();
}
@JsonIgnore
protected String getDefaultCollectionFormat() {
return "csv";
}
public T items(Property items) {
this.items = items;
return castThis();
}
public T _enum(List value) {
this._enum = value;
return castThis();
}
@JsonIgnore
@Override
public List getEnum() {
return _enum;
}
@Override
public void setEnum(List _enum) {
this._enum = _enum;
}
@JsonProperty("enum")
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy