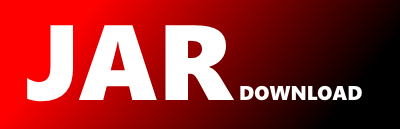
io.github.dan2097.jnainchi.InchiOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jna-inchi-api Show documentation
Show all versions of jna-inchi-api Show documentation
Functionality for calling the InChI library from Java. Requires an appropriate InChI binary on the classpath to use
/**
* JNA-InChI - Library for calling InChI from Java
* Copyright © 2018 Daniel Lowe
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package io.github.dan2097.jnainchi;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Locale;
public class InchiOptions {
static final InchiOptions DEFAULT_OPTIONS = new InchiOptionsBuilder().build();
private static final boolean IS_WINDOWS = System.getProperty("os.name", "").toLowerCase(Locale.ROOT).startsWith("windows");
private final List flags;
private final long timeoutMilliSecs;
private InchiOptions(InchiOptionsBuilder builder) {
this.flags = builder.flags;
this.timeoutMilliSecs = builder.timeoutMilliSecs;
}
public static class InchiOptionsBuilder {
private final List flags = new ArrayList<>();
private long timeoutMilliSecs = 0;
public InchiOptionsBuilder withFlag(InchiFlag... flags) {
for (InchiFlag flag : flags) {
this.flags.add(flag);
}
return this;
}
/**
* Timeout in seconds (0 = infinite timeout)
* @param timeoutSecs
* @return
*/
public InchiOptionsBuilder withTimeout(int timeoutSecs) {
if (timeoutSecs < 0) {
throw new IllegalArgumentException("Timeout should be a time in seconds or 0 for infinite: " + timeoutSecs);
}
this.timeoutMilliSecs = (long) timeoutSecs * 1000;
return this;
}
/**
* Timeout in milliseconds (0 = infinite timeout)
* @param timeoutMilliSecs
* @return
*/
public InchiOptionsBuilder withTimeoutMilliSeconds(long timeoutMilliSecs) {
if (timeoutMilliSecs < 0) {
throw new IllegalArgumentException("Timeout should be a time in milliseconds or 0 for infinite: " + timeoutMilliSecs);
}
this.timeoutMilliSecs = timeoutMilliSecs;
return this;
}
public InchiOptions build() {
return new InchiOptions(this);
}
}
public List getFlags() {
return Collections.unmodifiableList(flags);
}
public int getTimeout() {
return (int) (timeoutMilliSecs/1000);
}
public long getTimeoutMilliSeconds() {
return timeoutMilliSecs;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
for (InchiFlag inchiFlag : flags) {
if (sb.length() > 0) {
sb.append(' ');
}
sb.append(IS_WINDOWS ? "/" : "-");
sb.append(inchiFlag.toString());
}
if (timeoutMilliSecs != 0) {
if (sb.length() > 0) {
sb.append(' ');
}
sb.append(IS_WINDOWS ? "/" : "-");
sb.append("WM");
sb.append(String.valueOf(timeoutMilliSecs));
}
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy