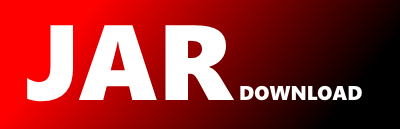
com.dao.support.util.StringUtil Maven / Gradle / Ivy
The newest version!
package com.dao.support.util;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.io.UnsupportedEncodingException;
import java.io.Writer;
import java.net.URLDecoder;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.text.DecimalFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.List;
import java.util.Random;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.servlet.http.HttpServletRequest;
/**
* 字串工具类
* @author jianghongyan
* @version v1.0
* @since v1.0
* 2017年3月6日 下午4:17:34
*/
public class StringUtil {
/**
* 将一个Double转为int的String,将省略小数点后面的值
*
* @param d double类型数据
* @return String
*/
public static String doubleToIntString(Double d) {
int value = ((Double) d).intValue();
return String.valueOf(value);
}
/**
* 检查浮点数
*
* @param num 待检查数字
* @param type
* "0+":非负浮点数 "+":正浮点数 "-0":非正浮点数 "-":负浮点数 "":浮点数
* @return boolean
*/
public static boolean checkFloat(String num, String type) {
String eL = "";
if (type.equals("0+"))
eL = "^\\d+(\\.\\d+)?$";// 非负浮点数
else if (type.equals("+"))
eL = "^((\\d+\\.\\d*[1-9]\\d*)|(\\d*[1-9]\\d*\\.\\d+)|(\\d*[1-9]\\d*))$";// 正浮点数
else if (type.equals("-0"))
eL = "^((-\\d+(\\.\\d+)?)|(0+(\\.0+)?))$";// 非正浮点数
else if (type.equals("-"))
eL = "^(-((\\d+\\.\\d*[1-9]\\d*)|(\\d*[1-9]\\d*\\.\\d+)|(\\d*[1-9]\\d*)))$";// 负浮点数
else
eL = "^(-?\\d+)(\\.\\d+)?$";// 浮点数
Pattern p = Pattern.compile(eL);
Matcher m = p.matcher(num);
boolean b = m.matches();
return b;
}
/**
* 检测某字串是否存在某数组中
*
* @param value 待检查字符串
* @param array 数组
* @return 存在返回真,不存在返回假
*/
public static boolean isInArray(String value, String[] array) {
if (array == null)
return false;
for (String v : array) {
if (v.equals(value))
return true;
}
return false;
}
public static boolean isInArray(int value, String[] array) {
if (array == null)
return false;
for (String v : array) {
if (Integer.valueOf(v).intValue() == value)
return true;
}
return false;
}
/**
* 将数组成str连接成字符串
*
* @param str 字符串
* @param array 数组
* @return string
*/
public static String implode(String str, Object[] array) {
if (str == null || array == null) {
return "";
}
String result = "";
for (int i = 0; i < array.length; i++) {
if (i == array.length - 1) {
result += array[i].toString();
} else {
result += array[i].toString() + str;
}
}
return result;
}
public static String implodeValue(String str, Object[] array) {
if (str == null || array == null) {
return "";
}
String result = "";
for (int i = 0; i < array.length; i++) {
if (i == array.length - 1) {
result += "?";
} else {
result += "?" + str;
}
}
return result;
}
/**
* MD5加密方法
*
* @param str
* String
* @return String
*/
public static String md5(String str) {
return md5(str,true);
}
public static String md5(String str, boolean zero) {
MessageDigest messageDigest = null;
try {
messageDigest = MessageDigest.getInstance("MD5");
} catch (NoSuchAlgorithmException ex) {
ex.printStackTrace();
return null;
}
byte[] resultByte = messageDigest.digest(str.getBytes());
StringBuffer result = new StringBuffer();
for (int i = 0; i < resultByte.length; ++i) {
int v = 0xFF & resultByte[i];
if(v<16 && zero)
result.append("0");
result.append(Integer.toHexString(v));
}
return result.toString();
}
public static void main(String[] args){
// //System.out.println(md5.getMD5("123456".getBytes()) );
//System.out.println( md5("123456"));
}
/**
* 验证Email地址是否有效
*
* @param sEmail 待检查的email地址
* @return boolean
*/
public static boolean validEmail(String sEmail) {
String pattern = "^([a-z0-9A-Z]+[-|\\.|_]?)+[a-z0-9A-Z]@([a-z0-9A-Z]+(-[a-z0-9A-Z]+)?\\.)+[a-zA-Z]{2,}$";
return sEmail.matches(pattern);
}
/**
* 验证字符最大长度
*
* @param str 待检查字符串
* @param length 长度
* @return boolean
*/
public static boolean validMaxLen(String str, int length) {
if (str == null || str.equals("")) {
return false;
}
if (str.length() > length) {
return false;
} else {
return true;
}
}
/**
* 验证字符最小长度
*
* @param str 待检查字符串
* @param length 长度
* @return boolean
*/
public static boolean validMinLen(String str, int length) {
if (str == null || str.equals("")) {
return false;
}
if (str.length() < length) {
return false;
} else {
return true;
}
}
/**
* 验证一个字符串是否为空
*
* @param str 待检查字符串
* @return 如果为空返回真,如果不为空返回假
*/
public static boolean isEmpty(String str) {
if (str == null || "".equals(str))
return true;
String pattern = "\\S";
Pattern p = Pattern.compile(pattern, 2 | Pattern.DOTALL);
Matcher m = p.matcher(str);
return !m.find();
}
/**
* 不为空的判断
* @param str 待检查字符串
* @return boolean
*/
public static boolean notEmpty(String str) {
return !isEmpty(str);
}
/**
* 验证两个字符串是否相等且不能为空
*
* @param str1 待检查字符串
* @param str2 待检查字符串
* @return boolean
*/
public static boolean equals(String str1, String str2) {
if (str1 == null || str1.equals("") || str2 == null || str2.equals("")) {
return false;
}
return str1.equals(str2);
}
/**
* 将字串转为数字
*
* @param str 待操作字符串
* @param checked 如果为treu格式不正确抛出异常
* @return int
*/
public static int toInt(String str, boolean checked) {
int value = 0;
if (str == null || str.equals("")) {
return 0;
}
try {
value = Integer.parseInt(str);
} catch (Exception ex) {
if (checked) {
throw new RuntimeException("整型数字格式不正确");
} else {
return 0;
}
}
return value;
}
/**
* 将object转为数字
*
* @param obj 需要转object的对象
* @param checked 如果为true格式不正确抛出异常
* @return int
*/
public static int toInt(Object obj, boolean checked) {
int value = 0;
if (obj == null ) {
return 0;
}
try {
value = Integer.parseInt(obj.toString());
} catch (Exception ex) {
if (checked) {
throw new RuntimeException("整型数字格式不正确");
} else {
return 0;
}
}
return value;
}
/**
* 将一个字串转为int,如果无空,则返回默认值
* @param str 要转换的数字字串
* @param defaultValue 默认值
* @return Integer
*/
public static Integer toInt(String str, Integer defaultValue) {
Integer value = defaultValue;
if (str == null || str.equals("")) {
return defaultValue;
}
try {
value = Integer.parseInt(str);
} catch (Exception ex) {
return defaultValue;
}
return value;
}
/**
* 将字符型转为Int型
*
* @param str 待操作字符串
* @return 返回的int值
*/
@Deprecated
public static int toInt(String str) {
int value = 0;
if (str == null || str.equals("")) {
return 0;
}
try {
value = Integer.parseInt(str);
} catch (Exception ex) {
value = 0;
ex.printStackTrace();
}
return value;
}
/**
* 已废弃
* @param str 待操作字符串
* @return 返回值
*/
@Deprecated
public static Double toDouble(String str) {
Double value = 0d;
if (str == null || str.equals("")) {
return 0d;
}
try {
value = Double.valueOf(str);
} catch (Exception ex) {
value = 0d;
// ex.printStackTrace();
}
return value;
}
/**
* 将一个字串转为double
*
* @param str 待操作字符串
* @param checked 如果为treu格式不正确抛出异常
* @return double
*/
public static Double toDouble(String str, boolean checked) {
Double value = 0d;
if (str == null || str.equals("")) {
return 0d;
}
try {
value = Double.valueOf(str);
} catch (Exception ex) {
if (checked)
throw new RuntimeException("数字格式不正确");
else
return 0D;
}
return value;
}
/**
* 将一个object转为double
* 如果object 为 null 则返回0;
*
* @param obj 需要转成Double的对象
* @param checked 如果为true格式不正确抛出异常
* @return double
*/
public static Double toDouble(Object obj, boolean checked) {
Double value = 0d;
if (obj == null ) {
if (checked)
throw new RuntimeException("数字格式不正确");
else
return 0D;
}
try {
value = Double.valueOf(obj.toString());
} catch (Exception ex) {
if (checked)
throw new RuntimeException("数字格式不正确");
else
return 0D;
}
return value;
}
/**
* 字符串转换为double
* @param str 待转换字符串
* @param defaultValue 默认值
* @return double
*/
public static Double toDouble(String str, Double defaultValue) {
Double value = defaultValue;
if (str == null || str.equals("")) {
return 0d;
}
try {
value = Double.valueOf(str);
} catch (Exception ex) {
ex.printStackTrace();
value =defaultValue;
}
return value;
}
/**
* 把数组转换成String
*
* @param array 数组
* @param split 分隔符
* @return string
*/
public static String arrayToString(Object[] array, String split) {
if (array == null) {
return "";
}
String str = "";
for (int i = 0; i < array.length; i++) {
if (i != array.length - 1) {
str += array[i].toString() + split;
} else {
str += array[i].toString();
}
}
return str;
}
/**
* 将一个list转为以split分隔的string
*
* @param list 目标list
* @param split 分割符
* @return string
*/
public static String listToString(List list, String split) {
if (list == null || list.isEmpty())
return "";
StringBuffer sb = new StringBuffer();
for (Object obj : list) {
if (sb.length() != 0) {
sb.append(split);
}
sb.append(obj.toString());
}
return sb.toString();
}
/**
* 得到WEB-INF的绝对路径
*
* @return string
*/
public static String getWebInfPath() {
String filePath = Thread.currentThread().getContextClassLoader()
.getResource("").toString();
if (filePath.toLowerCase().indexOf("file:") > -1) {
filePath = filePath.substring(6, filePath.length());
}
if (filePath.toLowerCase().indexOf("classes") > -1) {
filePath = filePath.replaceAll("/classes", "");
}
if (System.getProperty("os.name").toLowerCase().indexOf("window") < 0) {
filePath = "/" + filePath;
}
if (!filePath.endsWith("/"))
filePath += "/";
return filePath;
}
/**
* 格式化页码
*
* @param page 页码
* @return int 格式化后的页码
*/
public static int formatPage(String page) {
int iPage = 1;
if (page == null || page.equals("")) {
return iPage;
}
try {
iPage = Integer.parseInt(page);
} catch (Exception ex) {
iPage = 1;
}
return iPage;
}
/**
* 将计量单位字节转换为相应单位
*
* @param fileSize 计量单位
* @return string 相应单位
*/
public static String getFileSize(String fileSize) {
String temp = "";
DecimalFormat df = new DecimalFormat("0.00");
double dbFileSize = Double.parseDouble(fileSize);
if (dbFileSize >= 1024) {
if (dbFileSize >= 1048576) {
if (dbFileSize >= 1073741824) {
temp = df.format(dbFileSize / 1024 / 1024 / 1024) + " GB";
} else {
temp = df.format(dbFileSize / 1024 / 1024) + " MB";
}
} else {
temp = df.format(dbFileSize / 1024) + " KB";
}
} else {
temp = df.format(dbFileSize / 1024) + " KB";
}
return temp;
}
/**
* 得到一个32位随机字符
*
* @return string
*/
public static String getEntry() {
Random random = new Random(100);
Date now = new Date();
SimpleDateFormat formatter = new SimpleDateFormat(new String(
"yyyyMMddHHmmssS"));
return md5(formatter.format(now) + random.nextDouble());
}
/**
* 将中文汉字转成UTF8编码
*
* @param str 中文汉字
* @return string 转码后的结果
*/
public static String toUTF8(String str) {
if (str == null || str.equals("")) {
return "";
}
try {
return new String(str.getBytes("ISO8859-1"), "UTF-8");
} catch (Exception ex) {
ex.printStackTrace();
return "";
}
}
/**
* 按传入编码转码
* @param str 中文
* @param charset 编码格式
* @return 转码后的数据
*/
public static String to(String str, String charset) {
if (str == null || str.equals("")) {
return "";
}
try {
return new String(str.getBytes("UTF-8"), charset);
} catch (Exception ex) {
ex.printStackTrace();
return "";
}
}
/**
* 获得随机字符串
* @param n 字符串长度
* @return 随机字符串
*/
public static String getRandStr(int n) {
Random random = new Random();
String sRand = "";
for (int i = 0; i < n; i++) {
String rand = String.valueOf(random.nextInt(10));
sRand += rand;
}
return sRand;
}
/**
* 得到一个数字的大写(一到十之内)
*
* @param num 数字
* @return string
*/
public static String getChineseNum(int num) {
String[] chineseNum = new String[] { "一", "二", "三", "四", "五", "六", "七",
"八", "九", "十" };
return chineseNum[num];
}
/**
* 代替回车
* @param str 字符串
* @return 替代后的数据
*/
public static String replaceEnter(String str) {
if (str == null)
return null;
return str.replaceAll("\r", "").replaceAll("\n", "");
}
/**
* 替代所有
* @param source 原字符串
* @param target 目标内容
* @param content 替换内容
* @return 替换后的字符串
*/
public static String replaceAll(String source, String target, String content) {
StringBuffer buffer = new StringBuffer(source);
int start = buffer.indexOf(target);
if (start > 0) {
source = buffer.replace(start, start + target.length(), content)
.toString();
}
return source;
}
/**
* 去除HTML 元素
*
* @param element html内容
* @return string
*/
public static String getTxtWithoutHTMLElement(String element) {
if (null == element || "".equals(element.trim())) {
return element;
}
Pattern pattern = Pattern.compile("<[^<|^>]*>");
Matcher matcher = pattern.matcher(element);
StringBuffer txt = new StringBuffer();
while (matcher.find()) {
String group = matcher.group();
if (group.matches("<[\\s]*>")) {
matcher.appendReplacement(txt, group);
} else {
matcher.appendReplacement(txt, "");
}
}
matcher.appendTail(txt);
String temp = txt.toString().replaceAll("\n", "");
temp = temp.replaceAll(" ", "");
return temp;
}
/**
* clear trim to String
* @param strtrim 有trim的字符串
* @return string
*/
public static String toTrim(String strtrim) {
if (null != strtrim && !strtrim.equals("")) {
return strtrim.trim();
}
return "";
}
/**
* 转义字串的$
*
* @param str 待转换的字符串
* @return string
*/
public static String filterDollarStr(String str) {
String sReturn = "";
if (!toTrim(str).equals("")) {
if (str.indexOf('$', 0) > -1) {
while (str.length() > 0) {
if (str.indexOf('$', 0) > -1) {
sReturn += str.subSequence(0, str.indexOf('$', 0));
sReturn += "\\$";
str = str.substring(str.indexOf('$', 0) + 1,
str.length());
} else {
sReturn += str;
str = "";
}
}
} else {
sReturn = str;
}
}
return sReturn;
}
/**
* 压缩html
* @param html 待压缩的html
* @return 压缩后的html
*/
public static String compressHtml(String html) {
if (html == null)
return null;
html = html.replaceAll("[\\t\\n\\f\\r]", "");
return html;
}
/**
* 把double数据转换为金钱格式
* @param d 待转换的数据
* @return 金钱格式的数据
*/
public static String toCurrency(Double d) {
if (d != null) {
DecimalFormat df = new DecimalFormat("¥#,###.00");
return df.format(d);
}
return "";
}
/**
* 把int转换成string
* @param i 目标数字
* @return string
*/
public static String toString(Integer i) {
if (i != null) {
return String.valueOf(i);
}
return "";
}
/**
* 把double转换成string
* @param d 目标小数
* @return string
*/
public static String toString(Double d) {
if (null != d) {
return String.valueOf(d);
}
return "";
}
/**
* Object转String的方法
* @param o 需要转String的对象
* @param checked 是否检测异常
* @return String 转换之后的字符串
*/
public static String toString(Object o,boolean checked) {
String value="";
if (null != o) {
try {
value = o.toString();
} catch (Exception e) {
if (checked) {
throw new RuntimeException("String类型不正确");
}
}
}
return value;
}
/**
* Object转String的方法
* 若为空,或者转换出现异常
* @param o 需要转String的对象
* @return 转换之后的字符串
*/
public static String toString(Object o) {
String value="";
if (null != o) {
value =o.toString();
}
return value;
}
public static String getRandom() {
int[] array = { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 };
Random rand = new Random();
for (int i = 10; i > 1; i--) {
int index = rand.nextInt(i);
int tmp = array[index];
array[index] = array[i - 1];
array[i - 1] = tmp;
}
int result = 0;
for (int i = 0; i < 6; i++)
result = result * 10 + array[i];
return "" + result;
}
/**
* 处理树型码 获取本级别最大的code 如:301000 返回301999
*
* @param code 码
* @return int int
*/
public static int getMaxLevelCode(int code) {
String codeStr = "" + code;
StringBuffer str = new StringBuffer();
boolean flag = true;
for (int i = codeStr.length() - 1; i >= 0; i--) {
char c = codeStr.charAt(i);
if (c == '0' && flag) {
str.insert(0, '9');
} else {
str.insert(0, c);
flag = false;
}
}
return Integer.valueOf(str.toString());
}
/**
* 去掉sql的注释
* @param content sql内容
* @return string
*
*/
public static String delSqlComment(String content) {
String pattern = "/\\*(.|[\r\n])*?\\*/";
Pattern p = Pattern.compile(pattern, 2 | Pattern.DOTALL);
Matcher m = p.matcher(content);
if (m.find()) {
content = m.replaceAll("");
}
return content;
}
/**
* 把输入流转换成string
* @param is 输入流
* @return string
*/
public static String inputStream2String(InputStream is) {
BufferedReader in = new BufferedReader(new InputStreamReader(is));
StringBuffer buffer = new StringBuffer();
String line = "";
try {
while ((line = in.readLine()) != null) {
buffer.append(line);
}
} catch (IOException e) {
e.printStackTrace();
}
return buffer.toString();
}
/**
* 解码
* @param keyword 待解码数据
* @return string
*/
public static String decode(String keyword) {
try {
keyword = URLDecoder.decode(keyword, "UTF-8");
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return keyword;
}
/**
* 进行解析
*
* @param regex 正则
* @param rpstr 替换数据
* @param source 源数据
* @return string
*/
public static String doFilter(String regex, String rpstr, String source) {
Pattern p = Pattern.compile(regex, 2 | Pattern.DOTALL);
Matcher m = p.matcher(source);
return m.replaceAll(rpstr);
}
/**
* 脚本过滤
*
* @param source 源
* @return string
*/
public static String formatScript(String source) {
source = source.replaceAll("javascript", "javascript");
source = source.replaceAll("jscript:", "jscript:");
source = source.replaceAll("js:", "js:");
source = source.replaceAll("value", "value");
source = source.replaceAll("about:", "about:");
source = source.replaceAll("file:", "file:");
source = source.replaceAll("document.cookie", "documents.cookie");
source = source.replaceAll("vbscript:", "vbscript:");
source = source.replaceAll("vbs:", "vbs:");
source = doFilter("(on(mouse|exit|error|click|key))", "on$2",
source);
return source;
}
/**
* 格式化HTML代码
*
* @param htmlContent html数据
* @return string
*/
public static String htmlDecode(String htmlContent) {
htmlContent = formatScript(htmlContent);
htmlContent = htmlContent.replaceAll(" ", " ")
.replaceAll("<", "<").replaceAll(">", ">")
.replaceAll("\n\r", "
").replaceAll("\r\n", "
")
.replaceAll("\r", "
");
return htmlContent;
}
/**
* 动态添加表前缀,对没有前缀的表增加前缀
*
* @param table 表名
* @param prefix 前缀
* @return string
*/
public static String addPrefix(String table, String prefix) {
String result = "";
if (table.length() > prefix.length()) {
if (table.substring(0, prefix.length()).toLowerCase()
.equals(prefix.toLowerCase()))
result = table;
else
result = prefix + table;
} else
result = prefix + table;
return result;
}
/**
* 加后缀
* @param table 表名
* @param suffix 后缀
* @return string
*/
public static String addSuffix(String table, String suffix) {
String result = "";
if (table.length() > suffix.length()) {
int start = table.length() - suffix.length();
int end = start + suffix.length();
if (table.substring(start, end).toLowerCase()
.equals(suffix.toLowerCase()))
result = table;
else
result = table + suffix;
} else
result = table + suffix;
return result;
}
/**
* 得到异常的字串
* @param aThrowable 爆出的异常
* @return string
*/
public static String getStackTrace(Throwable aThrowable) {
final Writer result = new StringWriter();
final PrintWriter printWriter = new PrintWriter(result);
aThrowable.printStackTrace(printWriter); return result.toString();
}
/**
* 判断Integer是否为空
* @param i 需要校验的Integer对象
* @param includeZero 是否包含0
* @return boolean
*/
public static boolean isEmpty(Integer i,boolean includeZero){
if(includeZero){
if(i==null||i.equals(0)){
return true;
}
}
if(i==null){
return true;
}
return false;
}
/**
* 判断是否是数字,传入字符串,判断是否是数字,true 是 false 否
* @param str 字符串
* @return boolean
*/
public static boolean isNumber(String str) {
Pattern patternInteger = Pattern.compile("^[-\\+]?[\\d]*$"); //是否是整数
Pattern patternDouble = Pattern.compile("^[-\\+]?[.\\d]*$"); //是否是小数
return patternInteger.matcher(str).matches() || patternDouble.matcher(str).matches();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy