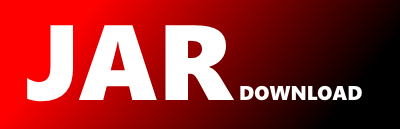
org.dinky.shaded.paimon.consumer.ConsumerManager Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.dinky.shaded.paimon.consumer;
import org.dinky.shaded.paimon.fs.FileIO;
import org.dinky.shaded.paimon.fs.Path;
import org.dinky.shaded.paimon.utils.DateTimeUtils;
import java.io.IOException;
import java.io.Serializable;
import java.io.UncheckedIOException;
import java.time.LocalDateTime;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.OptionalLong;
import java.util.stream.Collectors;
import static org.dinky.shaded.paimon.utils.FileUtils.listOriginalVersionedFiles;
import static org.dinky.shaded.paimon.utils.FileUtils.listVersionedFileStatus;
/** Manage consumer groups. */
public class ConsumerManager implements Serializable {
private static final long serialVersionUID = 1L;
private static final String CONSUMER_PREFIX = "consumer-";
private final FileIO fileIO;
private final Path tablePath;
public ConsumerManager(FileIO fileIO, Path tablePath) {
this.fileIO = fileIO;
this.tablePath = tablePath;
}
public Optional consumer(String consumerId) {
return Consumer.fromPath(fileIO, consumerPath(consumerId));
}
public void resetConsumer(String consumerId, Consumer consumer) {
try {
fileIO.overwriteFileUtf8(consumerPath(consumerId), consumer.toJson());
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
public void deleteConsumer(String consumerId) {
fileIO.deleteQuietly(consumerPath(consumerId));
}
public OptionalLong minNextSnapshot() {
try {
return listOriginalVersionedFiles(fileIO, consumerDirectory(), CONSUMER_PREFIX)
.map(this::consumer)
.filter(Optional::isPresent)
.map(Optional::get)
.mapToLong(Consumer::nextSnapshot)
.reduce(Math::min);
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
public void expire(LocalDateTime expireDateTime) {
try {
listVersionedFileStatus(fileIO, consumerDirectory(), CONSUMER_PREFIX)
.forEach(
status -> {
LocalDateTime modificationTime =
DateTimeUtils.toLocalDateTime(status.getModificationTime());
if (expireDateTime.isAfter(modificationTime)) {
fileIO.deleteQuietly(status.getPath());
}
});
} catch (IOException e) {
throw new RuntimeException(e);
}
}
/** Get all consumer. */
public Map consumers() throws IOException {
Map consumers = new HashMap<>();
listOriginalVersionedFiles(fileIO, consumerDirectory(), CONSUMER_PREFIX)
.forEach(
id -> {
Optional consumer = this.consumer(id);
consumer.ifPresent(value -> consumers.put(id, value.nextSnapshot()));
});
return consumers;
}
/** List all consumer IDs. */
public List listAllIds() {
try {
return listOriginalVersionedFiles(fileIO, consumerDirectory(), CONSUMER_PREFIX)
.collect(Collectors.toList());
} catch (IOException e) {
throw new UncheckedIOException(e);
}
}
private Path consumerDirectory() {
return new Path(tablePath + "/consumer");
}
private Path consumerPath(String consumerId) {
return new Path(tablePath + "/consumer/" + CONSUMER_PREFIX + consumerId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy