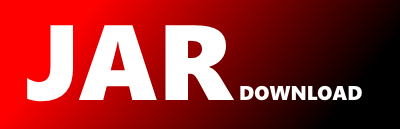
org.dinky.shaded.paimon.operation.MemoryFileStoreWrite Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.dinky.shaded.paimon.operation;
import org.dinky.shaded.paimon.CoreOptions;
import org.dinky.shaded.paimon.index.IndexMaintainer;
import org.dinky.shaded.paimon.io.cache.CacheManager;
import org.dinky.shaded.paimon.memory.HeapMemorySegmentPool;
import org.dinky.shaded.paimon.memory.MemoryOwner;
import org.dinky.shaded.paimon.memory.MemoryPoolFactory;
import org.dinky.shaded.paimon.metrics.MetricRegistry;
import org.dinky.shaded.paimon.operation.metrics.WriterBufferMetric;
import org.dinky.shaded.paimon.utils.FileStorePathFactory;
import org.dinky.shaded.paimon.utils.RecordWriter;
import org.dinky.shaded.paimon.utils.SnapshotManager;
import org.dinky.shaded.paimon.shade.guava30.com.google.common.collect.Iterators;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.annotation.Nullable;
import java.util.Iterator;
import java.util.Map;
import static org.dinky.shaded.paimon.CoreOptions.LOOKUP_CACHE_MAX_MEMORY_SIZE;
/**
* Base {@link FileStoreWrite} implementation which supports using shared memory and preempting
* memory from other writers.
*
* @param type of record to write.
*/
public abstract class MemoryFileStoreWrite extends AbstractFileStoreWrite {
private static final Logger LOG = LoggerFactory.getLogger(MemoryFileStoreWrite.class);
private final CoreOptions options;
protected final CacheManager cacheManager;
private MemoryPoolFactory writeBufferPool;
private WriterBufferMetric writerBufferMetric;
public MemoryFileStoreWrite(
String commitUser,
SnapshotManager snapshotManager,
FileStoreScan scan,
CoreOptions options,
@Nullable IndexMaintainer.Factory indexFactory,
String tableName,
FileStorePathFactory pathFactory) {
super(commitUser, snapshotManager, scan, indexFactory, tableName, pathFactory);
this.options = options;
this.cacheManager =
new CacheManager(
options.pageSize(),
options.toConfiguration().get(LOOKUP_CACHE_MAX_MEMORY_SIZE));
}
@Override
public FileStoreWrite withMemoryPoolFactory(MemoryPoolFactory memoryPoolFactory) {
this.writeBufferPool = memoryPoolFactory.addOwners(this::memoryOwners);
return this;
}
private Iterator memoryOwners() {
Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy