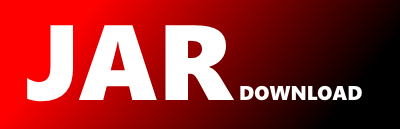
org.dinky.shaded.paimon.table.PrimaryKeyTableUtils Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.dinky.shaded.paimon.table;
import org.dinky.shaded.paimon.CoreOptions;
import org.dinky.shaded.paimon.KeyValue;
import org.dinky.shaded.paimon.mergetree.compact.DeduplicateMergeFunction;
import org.dinky.shaded.paimon.mergetree.compact.FirstRowMergeFunction;
import org.dinky.shaded.paimon.mergetree.compact.MergeFunctionFactory;
import org.dinky.shaded.paimon.mergetree.compact.PartialUpdateMergeFunction;
import org.dinky.shaded.paimon.mergetree.compact.aggregate.AggregateMergeFunction;
import org.dinky.shaded.paimon.options.Options;
import org.dinky.shaded.paimon.schema.KeyValueFieldsExtractor;
import org.dinky.shaded.paimon.schema.TableSchema;
import org.dinky.shaded.paimon.types.DataField;
import org.dinky.shaded.paimon.types.RowType;
import java.util.List;
import java.util.stream.Collectors;
import static org.dinky.shaded.paimon.schema.SystemColumns.KEY_FIELD_PREFIX;
/** Utils for creating changelog table with primary keys. */
public class PrimaryKeyTableUtils {
public static RowType addKeyNamePrefix(RowType type) {
return new RowType(addKeyNamePrefix(type.getFields()));
}
public static List addKeyNamePrefix(List keyFields) {
return keyFields.stream()
.map(
f ->
new DataField(
f.id(),
KEY_FIELD_PREFIX + f.name(),
f.type(),
f.description()))
.collect(Collectors.toList());
}
public static MergeFunctionFactory createMergeFunctionFactory(
TableSchema tableSchema, KeyValueFieldsExtractor extractor) {
RowType rowType = tableSchema.logicalRowType();
Options conf = Options.fromMap(tableSchema.options());
CoreOptions options = new CoreOptions(conf);
CoreOptions.MergeEngine mergeEngine = options.mergeEngine();
switch (mergeEngine) {
case DEDUPLICATE:
return DeduplicateMergeFunction.factory();
case PARTIAL_UPDATE:
return PartialUpdateMergeFunction.factory(conf, rowType, tableSchema.primaryKeys());
case AGGREGATE:
return AggregateMergeFunction.factory(
conf,
tableSchema.fieldNames(),
rowType.getFieldTypes(),
tableSchema.primaryKeys());
case FIRST_ROW:
return FirstRowMergeFunction.factory(
new RowType(extractor.keyFields(tableSchema)), rowType, conf);
default:
throw new UnsupportedOperationException("Unsupported merge engine: " + mergeEngine);
}
}
static class PrimaryKeyFieldsExtractor implements KeyValueFieldsExtractor {
private static final long serialVersionUID = 1L;
static final PrimaryKeyFieldsExtractor EXTRACTOR = new PrimaryKeyFieldsExtractor();
private PrimaryKeyFieldsExtractor() {}
@Override
public List keyFields(TableSchema schema) {
return addKeyNamePrefix(schema.trimmedPrimaryKeysFields());
}
@Override
public List valueFields(TableSchema schema) {
return schema.fields();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy