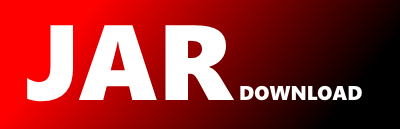
org.dinky.shaded.paimon.table.source.snapshot.IncrementalStartingScanner Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.dinky.shaded.paimon.table.source.snapshot;
import org.dinky.shaded.paimon.Snapshot;
import org.dinky.shaded.paimon.Snapshot.CommitKind;
import org.dinky.shaded.paimon.data.BinaryRow;
import org.dinky.shaded.paimon.io.DataFileMeta;
import org.dinky.shaded.paimon.table.source.DataSplit;
import org.dinky.shaded.paimon.table.source.ScanMode;
import org.dinky.shaded.paimon.table.source.Split;
import org.dinky.shaded.paimon.utils.Pair;
import org.dinky.shaded.paimon.utils.SnapshotManager;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/** {@link StartingScanner} for incremental changes by snapshot. */
public class IncrementalStartingScanner extends AbstractStartingScanner {
private long endingSnapshotId;
private ScanMode scanMode;
public IncrementalStartingScanner(
SnapshotManager snapshotManager, long start, long end, ScanMode scanMode) {
super(snapshotManager);
this.startingSnapshotId = start;
this.endingSnapshotId = end;
this.scanMode = scanMode;
}
@Override
public Result scan(SnapshotReader reader) {
Map, List> grouped = new HashMap<>();
for (long i = startingSnapshotId + 1; i < endingSnapshotId + 1; i++) {
List splits = readSplits(reader, snapshotManager.snapshot(i));
for (DataSplit split : splits) {
grouped.computeIfAbsent(
Pair.of(split.partition(), split.bucket()), k -> new ArrayList<>())
.addAll(split.dataFiles());
}
}
List result = new ArrayList<>();
for (Map.Entry, List> entry : grouped.entrySet()) {
BinaryRow partition = entry.getKey().getLeft();
int bucket = entry.getKey().getRight();
for (List files :
reader.splitGenerator().splitForBatch(entry.getValue())) {
result.add(
DataSplit.builder()
.withSnapshot(endingSnapshotId)
.withPartition(partition)
.withBucket(bucket)
.withDataFiles(files)
.build());
}
}
return StartingScanner.fromPlan(
new SnapshotReader.Plan() {
@Override
public Long watermark() {
return null;
}
@Override
public Long snapshotId() {
return endingSnapshotId;
}
@Override
public List splits() {
return (List) result;
}
});
}
private List readSplits(SnapshotReader reader, Snapshot s) {
switch (scanMode) {
case CHANGELOG:
return readChangeLogSplits(reader, s);
case DELTA:
return readDeltaSplits(reader, s);
default:
throw new UnsupportedOperationException("Unsupported scan kind: " + scanMode);
}
}
@SuppressWarnings({"unchecked", "rawtypes"})
private List readDeltaSplits(SnapshotReader reader, Snapshot s) {
if (s.commitKind() != CommitKind.APPEND) {
// ignore COMPACT and OVERWRITE
return Collections.emptyList();
}
return (List) reader.withSnapshot(s).withMode(ScanMode.DELTA).read().splits();
}
@SuppressWarnings({"unchecked", "rawtypes"})
private List readChangeLogSplits(SnapshotReader reader, Snapshot s) {
if (s.commitKind() == CommitKind.OVERWRITE) {
// ignore OVERWRITE
return Collections.emptyList();
}
return (List) reader.withSnapshot(s).withMode(ScanMode.CHANGELOG).read().splits();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy