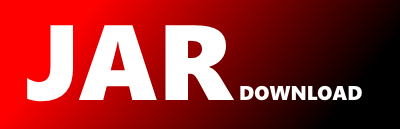
com.github.davaded.echarts.DataZoom Maven / Gradle / Ivy
/*
* The MIT License (MIT)
*
* Copyright (c) 2014-2015 [email protected]
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.github.davaded.echarts;
import java.util.Date;
import com.github.davaded.echarts.code.DataZoomType;
import com.github.davaded.echarts.code.FilterMode;
import com.github.davaded.echarts.code.Orient;
import com.github.davaded.echarts.style.TextStyle;
import lombok.Getter;
import lombok.Setter;
/**
* 数据区域缩放。与toolbox.feature.dataZoom同步,仅对直角坐标系图表有效
*
* @author liuzh
*/
@Getter
@Setter
public class DataZoom extends Basic implements Component {
/**
* 类型
*/
private DataZoomType type;
/**
* 显示label的小数精度。默认根据数据自动决定
*/
private String labelPrecision;
/**
* 显示的label的格式化器
*/
private Object labelFormatter;
/**
* 是否在 dataZoom-silder 组件中显示数据阴影。数据阴影可以简单得反应数据走势
*/
private String showDataShadow;
/**
* 文字样式
*/
private TextStyle textStyle;
/**
* 数据窗口范围的起始数值。如果设置了 dataZoom-slider.start 则 startValue 失效
*/
private Object startValue;
/**
* 数据窗口范围的结束数值。如果设置了 dataZoom-slider.end 则 endValue 失效
*/
private Object endValue;
/**
* 设置 dataZoom-inside 组件控制的 角度轴
*/
private Object angleAxisIndex;
/**
* 设置 dataZoom-inside 组件控制的 半径轴
*/
private Object radiusAxisIndex;
/**
* dataZoom 的运行原理是通过 数据过滤 来达到 数据窗口缩放 的效果
*/
private FilterMode filterMode;
/**
* 设置触发视图刷新的频率。单位为毫秒(ms)。一般不需要更改这个值
*/
private Integer throttle;
/**
* 布局方式,默认为水平布局,可选为:'horizontal' | 'vertical'
*/
private Orient orient;
/**
* 默认#ccc,数据缩略背景颜色
*/
private String dataBackgroundColor;
/**
* 默认值rgba(144,197,237,0.2),选择区域填充颜色
*/
private String fillerColor;
/**
* 默认值rgba(70,130,180,0.8),控制手柄颜色
*/
private String handleColor;
/**
* 控制手柄大小
*/
private Integer handleSize;
/**
* 当不指定时默认控制所有横向类目,可通过数组指定多个需要控制的横向类目坐标轴Index,仅一个时可直接为数字
*/
private Object xAxisIndex;
/**
* 当不指定时默认控制所有纵向类目,可通过数组指定多个需要控制的纵向类目坐标轴Index,仅一个时可直接为数字
*/
private Object yAxisIndex;
/**
* 数据缩放,选择起始比例,默认为0(%),从首个数据起选择
*/
private Integer start;
/**
* 数据缩放,选择结束比例,默认为100(%),到最后一个数据选择结束
*/
private Integer end;
/**
* 缩放变化是否实时显示,建议性能较低的浏览器或数据量巨大时不启动实时效果
*/
private Boolean realtime;
/**
* 数据缩放锁,默认为false,当设置为true时选择区域不能伸缩,即(end - start)值保持不变,仅能做数据漫游
*/
private Boolean zoomLock;
/**
* 缩放变化是否显示定位详情
*/
private Boolean showDetail;
private Object minValueSpan;
/**
* label precision
*
* @return {@link String}
*/
public String labelPrecision() {
return this.labelPrecision;
}
/**
* label precision
*
* @param labelPrecision label precision
* @return {@link DataZoom}
*/
public DataZoom labelPrecision(String labelPrecision) {
this.labelPrecision = labelPrecision;
return this;
}
/**
* label formatter
*
* @return {@link Object}
*/
public Object labelFormatter() {
return this.labelFormatter;
}
/**
* label formatter
*
* @param labelFormatter label formatter
* @return {@link DataZoom}
*/
public DataZoom labelFormatter(Object labelFormatter) {
this.labelFormatter = labelFormatter;
return this;
}
/**
* show data shadow
*
* @return {@link String}
*/
public String showDataShadow() {
return this.showDataShadow;
}
/**
* show data shadow
*
* @param showDataShadow show data shadow
* @return {@link DataZoom}
*/
public DataZoom showDataShadow(String showDataShadow) {
this.showDataShadow = showDataShadow;
return this;
}
/**
* text style
*
* @return {@link TextStyle}
*/
public TextStyle textStyle() {
if (this.textStyle == null) {
this.textStyle = new TextStyle();
}
return this.textStyle;
}
/**
* text style
*
* @param textStyle text style
* @return {@link DataZoom}
*/
public DataZoom textStyle(TextStyle textStyle) {
this.textStyle = textStyle;
return this;
}
/**
* start value
*
* @return {@link Object}
*/
public Object startValue() {
return this.startValue;
}
/**
* start value
*
* @param startValue start value
* @return {@link DataZoom}
*/
public DataZoom startValue(Object startValue) {
this.startValue = startValue;
return this;
}
/**
* start value
*
* @param startValue start value
* @return {@link DataZoom}
*/
public DataZoom startValue(String startValue) {
this.startValue = startValue;
return this;
}
/**
* start value
*
* @param startValue start value
* @return {@link DataZoom}
*/
public DataZoom startValue(Integer startValue) {
this.startValue = startValue;
return this;
}
/**
* start value
*
* @param startValue start value
* @return {@link DataZoom}
*/
public DataZoom startValue(Date startValue) {
this.startValue = startValue;
return this;
}
/**
* end value
*
* @return {@link Object}
*/
public Object endValue() {
return this.endValue;
}
/**
* end value
*
* @param endValue end value
* @return {@link DataZoom}
*/
public DataZoom endValue(Object endValue) {
this.endValue = endValue;
return this;
}
/**
* end value
*
* @param endValue end value
* @return {@link DataZoom}
*/
public DataZoom endValue(Integer endValue) {
this.endValue = endValue;
return this;
}
/**
* end value
*
* @param endValue end value
* @return {@link DataZoom}
*/
public DataZoom endValue(String endValue) {
this.endValue = endValue;
return this;
}
/**
* end value
*
* @param endValue end value
* @return {@link DataZoom}
*/
public DataZoom endValue(Date endValue) {
this.endValue = endValue;
return this;
}
/**
* type
*
* @return {@link DataZoomType}
*/
public DataZoomType type() {
return this.type;
}
/**
* type
*
* @param type type
* @return {@link DataZoom}
*/
public DataZoom type(DataZoomType type) {
this.type = type;
return this;
}
/**
* angle axis index
*
* @return {@link Object}
*/
public Object angleAxisIndex() {
return this.angleAxisIndex;
}
/**
* angle axis index
*
* @param angleAxisIndex angle axis index
* @return {@link DataZoom}
*/
public DataZoom angleAxisIndex(Object angleAxisIndex) {
this.angleAxisIndex = angleAxisIndex;
return this;
}
/**
* angle axis index
*
* @param angleAxisIndex angle axis index
* @return {@link DataZoom}
*/
public DataZoom angleAxisIndex(Integer angleAxisIndex) {
this.angleAxisIndex = angleAxisIndex;
return this;
}
/**
* angle axis index
*
* @param angleAxisIndex angle axis index
* @return {@link DataZoom}
*/
public DataZoom angleAxisIndex(Integer... angleAxisIndex) {
this.angleAxisIndex = angleAxisIndex;
return this;
}
/**
* radius axis index
*
* @return {@link Object}
*/
public Object radiusAxisIndex() {
return this.radiusAxisIndex;
}
/**
* radius axis index
*
* @param radiusAxisIndex radius axis index
* @return {@link DataZoom}
*/
public DataZoom radiusAxisIndex(Object radiusAxisIndex) {
this.radiusAxisIndex = radiusAxisIndex;
return this;
}
/**
* radius axis index
*
* @param radiusAxisIndex radius axis index
* @return {@link DataZoom}
*/
public DataZoom radiusAxisIndex(Integer radiusAxisIndex) {
this.radiusAxisIndex = radiusAxisIndex;
return this;
}
/**
* radius axis index
*
* @param radiusAxisIndex radius axis index
* @return {@link DataZoom}
*/
public DataZoom radiusAxisIndex(Integer... radiusAxisIndex) {
this.radiusAxisIndex = radiusAxisIndex;
return this;
}
/**
* filter mode
*
* @return {@link FilterMode}
*/
public FilterMode filterMode() {
return this.filterMode;
}
/**
* filter mode
*
* @param filterMode filter mode
* @return {@link DataZoom}
*/
public DataZoom filterMode(FilterMode filterMode) {
this.filterMode = filterMode;
return this;
}
/**
* throttle
*
* @return {@link Integer}
*/
public Integer throttle() {
return this.throttle;
}
/**
* throttle
*
* @param throttle throttle
* @return {@link DataZoom}
*/
public DataZoom throttle(Integer throttle) {
this.throttle = throttle;
return this;
}
/**
* handle size
* 获取handleSize值
*
* @return {@link Integer}
*/
public Integer handleSize() {
return this.handleSize;
}
/**
* handle size
* 设置handleSize值
*
* @param handleSize handleSize
* @return {@link DataZoom}
*/
public DataZoom handleSize(Integer handleSize) {
this.handleSize = handleSize;
return this;
}
/**
* orient
* 获取orient值
*
* @return {@link Orient}
*/
public Orient orient() {
return this.orient;
}
/**
* orient
* 设置orient值
*
* @param orient orient
* @return {@link DataZoom}
*/
public DataZoom orient(Orient orient) {
this.orient = orient;
return this;
}
/**
* data background color
* 获取dataBackgroundColor值
*
* @return {@link String}
*/
public String dataBackgroundColor() {
return this.dataBackgroundColor;
}
/**
* data background color
* 设置dataBackgroundColor值
*
* @param dataBackgroundColor data background color
* @return {@link DataZoom}
*/
public DataZoom dataBackgroundColor(String dataBackgroundColor) {
this.dataBackgroundColor = dataBackgroundColor;
return this;
}
/**
* filler color
* 获取fillerColor值
*
* @return {@link String}
*/
public String fillerColor() {
return this.fillerColor;
}
/**
* filler color
* 设置fillerColor值
*
* @param fillerColor filler color
* @return {@link DataZoom}
*/
public DataZoom fillerColor(String fillerColor) {
this.fillerColor = fillerColor;
return this;
}
/**
* handle color
* 获取handleColor值
*
* @return {@link String}
*/
public String handleColor() {
return this.handleColor;
}
/**
* handle color
* 设置handleColor值
*
* @param handleColor handle color
* @return {@link DataZoom}
*/
public DataZoom handleColor(String handleColor) {
this.handleColor = handleColor;
return this;
}
/**
* x axis index
* 获取xAxisIndex值
*
* @return {@link Object}
*/
public Object xAxisIndex() {
return this.xAxisIndex;
}
/**
* x axis index
* 设置xAxisIndex值
*
* @param xAxisIndex x axis index
* @return {@link DataZoom}
*/
public DataZoom xAxisIndex(Object xAxisIndex) {
this.xAxisIndex = xAxisIndex;
return this;
}
/**
* y axis index
* 获取yAxisIndex值
*
* @return {@link Object}
*/
public Object yAxisIndex() {
return this.yAxisIndex;
}
/**
* y axis index
* 设置yAxisIndex值
*
* @param yAxisIndex y axis index
* @return {@link DataZoom}
*/
public DataZoom yAxisIndex(Object yAxisIndex) {
this.yAxisIndex = yAxisIndex;
return this;
}
/**
* start
* 获取start值
*
* @return {@link Integer}
*/
public Integer start() {
return this.start;
}
/**
* start
* 设置start值
*
* @param start start
* @return {@link DataZoom}
*/
public DataZoom start(Integer start) {
this.start = start;
return this;
}
/**
* end
* 获取end值
*
* @return {@link Integer}
*/
public Integer end() {
return this.end;
}
/**
* end
* 设置end值
*
* @param end end
* @return {@link DataZoom}
*/
public DataZoom end(Integer end) {
this.end = end;
return this;
}
/**
* realtime
* 获取realtime值
*
* @return {@link Boolean}
*/
public Boolean realtime() {
return this.realtime;
}
/**
* realtime
* 设置realtime值
*
* @param realtime realtime
* @return {@link DataZoom}
*/
public DataZoom realtime(Boolean realtime) {
this.realtime = realtime;
return this;
}
/**
* zoom lock
* 获取zoomLock值
*
* @return {@link Boolean}
*/
public Boolean zoomLock() {
return this.zoomLock;
}
/**
* zoom lock
* 设置zoomLock值
*
* @param zoomLock zoom lock
* @return {@link DataZoom}
*/
public DataZoom zoomLock(Boolean zoomLock) {
this.zoomLock = zoomLock;
return this;
}
/**
* show detail
* 获取showDetail值
*
* @return {@link Boolean}
*/
public Boolean showDetail() {
return this.showDetail;
}
/**
* show detail
* 设置showDetail值
*
* @param showDetail show detail
* @return {@link DataZoom}
*/
public DataZoom showDetail(Boolean showDetail) {
this.showDetail = showDetail;
return this;
}
/**
* min value span
* minValueSpan
*
* @param minValueSpan min value span
* @return {@link DataZoom}
*/
public DataZoom minValueSpan(Object minValueSpan) {
this.minValueSpan = minValueSpan;
return this;
}
/**
* min value span
* minValueSpan
*
* @return {@link Object}
*/
public Object minValueSpan() {
return this.minValueSpan;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy