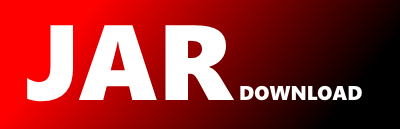
io.github.dbstarll.utils.json.jackson.EventStreamConvertResponseHandler Maven / Gradle / Ivy
The newest version!
package io.github.dbstarll.utils.json.jackson;
import io.github.dbstarll.utils.net.api.index.AbstractIndex;
import io.github.dbstarll.utils.net.api.index.EventStream;
import io.github.dbstarll.utils.net.api.index.Index;
import org.apache.commons.lang3.StringUtils;
import org.apache.hc.core5.http.ClassicHttpResponse;
import org.apache.hc.core5.http.ContentType;
import org.apache.hc.core5.http.HttpEntity;
import org.apache.hc.core5.http.HttpException;
import org.apache.hc.core5.http.io.HttpClientResponseHandler;
import org.apache.hc.core5.http.io.entity.StringEntity;
import org.apache.hc.core5.http.io.support.ClassicResponseBuilder;
import java.io.IOException;
import java.util.function.Predicate;
final class EventStreamConvertResponseHandler implements HttpClientResponseHandler> {
private final HttpClientResponseHandler extends Index> original;
private final HttpClientResponseHandler target;
private final Predicate ignore;
EventStreamConvertResponseHandler(final HttpClientResponseHandler extends Index> original,
final HttpClientResponseHandler target, final Predicate ignore) {
this.original = original;
this.target = target;
this.ignore = ignore;
}
@Override
public Index handleResponse(final ClassicHttpResponse response) throws HttpException, IOException {
final Index eventStreamIndex = original.handleResponse(response);
if (eventStreamIndex == null) {
return null;
}
final EventStream eventStream = eventStreamIndex.getData();
final T data = eventStream == null || StringUtils.isBlank(eventStream.getData()) || ignore.test(eventStream)
? null : handleResponse(response, eventStream.getData());
return new AbstractIndex(data, eventStreamIndex.getIndex()) {
};
}
private T handleResponse(final ClassicHttpResponse response, final String content)
throws IOException, HttpException {
final ClassicHttpResponse classicHttpResponse = ClassicResponseBuilder.create(response.getCode())
.setVersion(response.getVersion())
.setHeaders(response.getHeaders())
.setEntity(buildEntity(response, content))
.build();
return target.handleResponse(classicHttpResponse);
}
private HttpEntity buildEntity(final ClassicHttpResponse response, final String content) {
return new StringEntity(content, ContentType.parse(response.getEntity().getContentType()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy