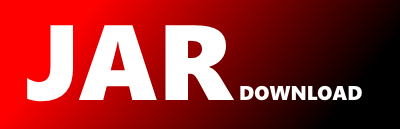
io.dddspring.common.port.adapter.persistence.ResultSetObjectMapper Maven / Gradle / Ivy
// Copyright 2012,2013 Vaughn Vernon
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package io.dddspring.common.port.adapter.persistence;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.ParameterizedType;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.util.*;
public class ResultSetObjectMapper {
private String columnPrefix;
private JoinOn joinOn;
private ResultSet resultSet;
private Class extends T> resultType;
public ResultSetObjectMapper(
ResultSet aResultSet,
Class aResultType,
JoinOn aJoinOn) {
super();
this.joinOn = aJoinOn;
this.resultSet = aResultSet;
this.resultType = aResultType;
}
public ResultSetObjectMapper(
ResultSet aResultSet,
Class extends T> aResultType,
String aColumnPrefix,
JoinOn aJoinOn) {
super();
this.columnPrefix = aColumnPrefix;
this.joinOn = aJoinOn;
this.resultSet = aResultSet;
this.resultType = aResultType;
}
public T mapResultToType() {
T object = this.createFrom(this.resultType());
Set associationsToMap = new HashSet();
Field[] fields = this.resultType().getDeclaredFields();
for (Field field : fields) {
String columnName = this.fieldNameToColumnName(field.getName());
if (this.hasColumn(this.resultSet(), columnName)) {
Object columnValue = this.columnValueFrom(columnName, field.getType());
this.joinOn().saveCurrentLeftQualifier(columnName, columnValue);
try {
field.setAccessible(true);
field.set(object, columnValue);
} catch (Exception e) {
throw new IllegalStateException(
"Cannot map to: "
+ this.resultType.getSimpleName()
+ "#"
+ columnName);
}
} else {
String objectPrefix = this.toObjectPrefix(columnName);
if (!associationsToMap.contains(objectPrefix) &&
this.hasAssociation(this.resultSet(), objectPrefix)) {
associationsToMap.add(field.getName());
}
}
}
if (!associationsToMap.isEmpty()) {
this.mapAssociations(object, this.resultSet(), associationsToMap);
}
return object;
}
private String columnPrefix() {
return this.columnPrefix;
}
private boolean hasColumnPrefix() {
return this.columnPrefix != null;
}
private Object columnValueFrom(String aColumnName, Class> aType) {
Object value = null;
String tempStr = null;
try {
String typeName = aType.getName();
if (aType.isPrimitive()) {
// boolean, byte, char, short, int, long, float, double
if (typeName.equals("int")) {
value = new Integer(this.resultSet().getInt(aColumnName));
} else if (typeName.equals("long")) {
value = new Long(this.resultSet().getLong(aColumnName));
} else if (typeName.equals("boolean")) {
int oneOrZero = this.resultSet().getInt(aColumnName);
value = oneOrZero == 1 ? Boolean.TRUE : Boolean.FALSE;
} else if (typeName.equals("short")) {
value = new Short(this.resultSet().getShort(aColumnName));
} else if (typeName.equals("float")) {
value = new Float(this.resultSet().getFloat(aColumnName));
} else if (typeName.equals("double")) {
value = new Double(this.resultSet().getDouble(aColumnName));
} else if (typeName.equals("byte")) {
value = new Byte(this.resultSet().getByte(aColumnName));
} else if (typeName.equals("char")) {
String charStr = this.resultSet().getString(aColumnName);
if (charStr == null) {
value = new Character((char) 0);
} else {
value = charStr.charAt(0);
}
}
} else {
if (typeName.equals("java.lang.String")) {
value = this.resultSet().getString(aColumnName);
} else if (typeName.equals("java.lang.Integer")) {
tempStr = this.resultSet().getString(aColumnName);
value = tempStr == null ? null: Integer.parseInt(tempStr);
} else if (typeName.equals("java.lang.Long")) {
tempStr = this.resultSet().getString(aColumnName);
value = tempStr == null ? null : Long.parseLong(tempStr);
} else if (typeName.equals("java.lang.Boolean")) {
int oneOrZero = this.resultSet().getInt(aColumnName);
value = oneOrZero == 1 ? Boolean.TRUE : Boolean.FALSE;
} else if (typeName.equals("java.util.Date")) {
java.sql.Timestamp timestamp = this.resultSet().getTimestamp(aColumnName);
if (timestamp != null) {
value = new java.util.Date(timestamp.getTime() + timestamp.getNanos());
}
} else if (typeName.equals("java.lang.Short")) {
tempStr = this.resultSet().getString(aColumnName);
value = tempStr == null ? null: Short.parseShort(tempStr);
} else if (typeName.equals("java.lang.Float")) {
tempStr = this.resultSet().getString(aColumnName);
value = tempStr == null ? null : Float.parseFloat(tempStr);
} else if (typeName.equals("java.lang.Double")) {
tempStr = this.resultSet().getString(aColumnName);
value = tempStr == null ? null : Double.parseDouble(tempStr);
}
}
} catch (Exception e) {
throw new IllegalArgumentException(
"Cannot map "
+ aColumnName
+ " because: "
+ e.getMessage(),
e);
}
return value;
}
private Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy