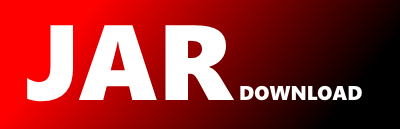
io.github.dengchen2020.message.dingtalk.DingTalkClientImpl Maven / Gradle / Ivy
package io.github.dengchen2020.message.dingtalk;
import com.fasterxml.jackson.databind.node.ArrayNode;
import com.fasterxml.jackson.databind.node.ObjectNode;
import io.github.dengchen2020.core.utils.JsonUtils;
import io.github.dengchen2020.core.utils.RestClientUtils;
import io.github.dengchen2020.message.config.MessageAutoConfiguration;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.MediaType;
import org.springframework.scheduling.annotation.Async;
import org.springframework.util.StringUtils;
import java.util.List;
/**
* 钉钉webhook推送默认实现
*
* @author xiaochen
* @since 2023/3/11
*/
@Async(MessageAutoConfiguration.MESSAGE_SEND_ASYNC_EXECUTOR)
public class DingTalkClientImpl implements DingTalkClient {
private static final Logger log = LoggerFactory.getLogger(DingTalkClientImpl.class);
private final String webhook;
public DingTalkClientImpl(String webhook) {
this.webhook = webhook;
}
@Override
public void sendText(String text) {
sendText(text, null, webhook);
}
@Override
public void sendText(String text, List phones) {
sendText(text, phones, webhook);
}
@Override
public void sendText(String text, String webhook) {
sendText(text, null, webhook);
}
@Override
public void sendText(String text, List phones, String webhook) {
if (!StringUtils.hasText(webhook)) {
log.warn("未配置钉钉webhook");
return;
}
try {
ObjectNode push = JsonUtils.getObjectMapper().createObjectNode();
ObjectNode textObj = JsonUtils.getObjectMapper().createObjectNode();
push.put("msgtype", "text");
push.set("text", textObj);
textObj.put("content", text);
if (phones != null && phones.isEmpty()) {
ObjectNode atObj = JsonUtils.getObjectMapper().createObjectNode();
push.set("at", atObj);
ArrayNode atMobile = JsonUtils.getObjectMapper().createArrayNode();
phones.forEach(atMobile::add);
atObj.set("atMobiles", atMobile);
}
RestClientUtils.post().uri(webhook)
.contentType(MediaType.APPLICATION_JSON)
.header("Host", "oapi.dingtalk.com")
.body(push).retrieve().toBodilessEntity();
} catch (Exception e) {
log.error("钉钉机器人发送信息{}失败:{}", text, e.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy