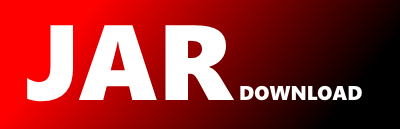
edu.uci.ics.jung.visualization.spatial.rtree.Node Maven / Gradle / Ivy
package edu.uci.ics.jung.visualization.spatial.rtree;
import edu.uci.ics.jung.visualization.spatial.TreeNode;
import java.awt.*;
import java.awt.geom.Point2D;
import java.awt.geom.Rectangle2D;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Interface for R-Tree nodes Includes static functions for area, union, margin, overlap
*
* @author Tom Nelson
*/
public interface Node extends TreeNode, Bounded {
Logger log = LoggerFactory.getLogger(Node.class);
public static final int M = 10;
public static final int m = (int) (M * .4); // m is 40% of M
String asString(String margin);
T getPickedObject(Point2D p);
int size();
void setParent(Node node);
Optional> getParent();
Node add(SplitterContext splitterContext, T element, Rectangle2D bounds);
boolean isLeafChildren();
int count();
Set> getContainingLeafs(Set> containingLeafs, double x, double y);
Set> getContainingLeafs(Set> containingLeafs, Point2D p);
LeafNode getContainingLeaf(T element);
Node remove(T element);
Node recalculateBounds();
Collection collectGrids(Collection list);
Set getVisibleElements(Set visibleElements, Shape shape);
static String asString(List rectangles) {
StringBuilder sb = new StringBuilder();
for (Shape r : rectangles) {
sb.append(asString(r.getBounds()));
sb.append("\n");
}
return sb.toString();
}
static String asString(Rectangle2D r) {
return "["
+ (int) r.getX()
+ ","
+ (int) r.getY()
+ ","
+ (int) r.getWidth()
+ ","
+ (int) r.getHeight()
+ "]";
}
static String asString(Map.Entry entry) {
return entry.getKey() + "->" + asString(entry.getValue());
}
static String asString(Node node, String margin) {
StringBuilder s = new StringBuilder();
s.append(margin);
s.append("bounds=");
s.append(asString(node.getBounds()));
s.append('\n');
s.append(node.asString(margin + marginIncrement));
return s.toString();
}
String marginIncrement = " ";
static Rectangle2D entryBoundingBox(Collection> entries) {
Rectangle2D boundingBox = null;
for (Map.Entry entry : entries) {
Rectangle2D rectangle = entry.getValue();
if (boundingBox == null) {
boundingBox = rectangle;
} else {
boundingBox = boundingBox.createUnion(rectangle);
}
}
return boundingBox;
}
static Rectangle2D nodeBoundingBox(Collection> nodes) {
Rectangle2D boundingBox = null;
for (Node node : nodes) {
Rectangle2D rectangle = node.getBounds();
if (boundingBox == null) {
boundingBox = rectangle;
} else {
boundingBox = boundingBox.createUnion(rectangle);
}
}
return boundingBox;
}
static Rectangle2D boundingBox(Collection rectangles) {
Rectangle2D boundingBox = null;
for (Rectangle2D rectangle : rectangles) {
if (boundingBox == null) {
boundingBox = rectangle;
} else {
boundingBox = boundingBox.createUnion(rectangle);
}
}
return boundingBox;
}
static double area(Collection rectangles) {
return area(boundingBox(rectangles));
}
static double nodeArea(Collection> nodes) {
return area(nodeBoundingBox(nodes));
}
static double entryArea(Collection> entries) {
return area(entryBoundingBox(entries));
}
static double entryArea(
Collection> left, Collection> right) {
return entryArea(left) + entryArea(right);
}
static double nodeArea(Collection> left, Collection> right) {
return nodeArea(left) + nodeArea(right);
}
static double area(Collection left, Collection right) {
return area(left) + area(right);
}
static double area(Rectangle2D r) {
double area = r.getWidth() * r.getHeight();
return area < 0 ? -area : area;
}
static double area(Rectangle2D left, Rectangle2D right) {
return area(left) + area(right);
}
static double margin(Collection rectangles) {
return margin(boundingBox(rectangles));
}
static double margin(Rectangle2D r) {
double width = r.getMaxX() - r.getMinX();
double height = r.getMaxY() - r.getMinY();
return 2 * (width + height);
}
static double margin(Rectangle2D left, Rectangle2D right) {
return margin(left) + margin(right);
}
static double margin(Collection left, Collection right) {
return margin(left) + margin(right);
}
static double nodeMargin(Collection> left, Collection> right) {
return margin(nodeBoundingBox(left)) + margin(nodeBoundingBox(right));
}
static double entryMargin(
Collection> left, Collection> right) {
return margin(entryBoundingBox(left)) + margin(entryBoundingBox(right));
}
static double entryOverlap(
Collection> left, Collection> right) {
return overlap(entryBoundingBox(left), entryBoundingBox(right));
}
static double nodeOverlap(Collection> left, Collection> right) {
return overlap(nodeBoundingBox(left), nodeBoundingBox(right));
}
static double overlap(Collection left, Collection right) {
return overlap(boundingBox(left), boundingBox(right));
}
static double overlap(Rectangle2D left, Rectangle2D right) {
return area(left.createIntersection(right));
}
static Rectangle2D union(Collection extends Bounded> boundedItems) {
Rectangle2D union = null;
for (Bounded r : boundedItems) {
if (union == null) {
union = r.getBounds();
} else {
union = r.getBounds().createUnion(union);
}
}
return union;
}
static double width(Collection extends Bounded> boundedItems) {
double min = 600;
double max = 0;
for (Bounded b : boundedItems) {
min = Math.min(b.getBounds().getMinX(), min);
max = Math.max(b.getBounds().getMaxX(), max);
}
return max - min;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy