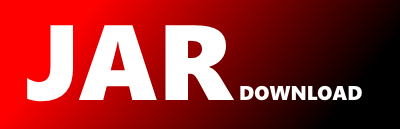
edu.uci.ics.jung.visualization.spatial.rtree.VerticalEdgeNodeComparator Maven / Gradle / Ivy
package edu.uci.ics.jung.visualization.spatial.rtree;
import java.awt.geom.Rectangle2D;
import java.util.Comparator;
import java.util.Map;
/**
* A comparator to compare along the y-axis, Nodes where the values are Rectangle2D First compare
* the min y values, then the max y values
*
* @author Tom Nelson
* @param
*/
public class VerticalEdgeNodeComparator implements Comparator> {
/**
* Compares its two arguments for order. Returns a negative integer, zero, or a positive integer
* as the first argument is less than, equal to, or greater than the second.
*
*
*
*
In the foregoing description, the notation sgn(expression)
* designates the mathematical signum function, which is defined to return one of
* -1, 0, or 1 according to whether the value of expression is
* negative, zero or positive.
*
*
*
*
The implementor must ensure that sgn(compare(x, y)) == -sgn(compare(y, x)) for all
* x and y. (This implies that compare(x, y) must throw an exception if
* and only if compare(y, x) throws an exception.)
*
*
*
*
The implementor must also ensure that the relation is transitive: ((compare(x, y)>0)
* && (compare(y, z)>0)) implies compare(x, z)>0.
*
*
*
*
Finally, the implementor must ensure that compare(x, y)==0 implies that
* sgn(compare(x, z))==sgn(compare(y, z)) for all z.
*
*
*
*
It is generally the case, but not strictly required that (compare(x, y)==0) ==
* (x.equals(y)). Generally speaking, any comparator that violates this condition should
* clearly indicate this fact. The recommended language is "Note: this comparator imposes
* orderings that are inconsistent with equals."
*
* @param left the first object to be compared.
* @param right the second object to be compared.
* @return a negative integer, zero, or a positive integer as the first argument is less than,
* equal to, or greater than the second.
* @throws NullPointerException if an argument is null and this comparator does not permit null
* arguments
* @throws ClassCastException if the arguments' types prevent them from being compared by this
* comparator.
*/
public int compare(Rectangle2D left, Rectangle2D right) {
if (left.getMinY() == right.getMinY()) {
if (left.getMaxY() == right.getMaxY()) return 0;
if (left.getMaxY() < right.getMaxY()) return -1;
else return 1;
} else {
if (left.getMinY() < right.getMinY()) return -1;
return 1;
}
}
public int compare(Map.Entry, Rectangle2D> leftNode, Map.Entry, Rectangle2D> rightNode) {
return compare(leftNode.getValue(), rightNode.getValue());
}
@Override
public int compare(Node leftNode, Node rightNode) {
return compare(leftNode.getBounds(), rightNode.getBounds());
}
}