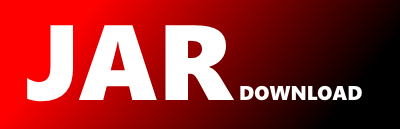
io.github.devsecops.engine.domain.resolver.strategy.impl.PropertyFileResolverStrategy Maven / Gradle / Ivy
package io.github.devsecops.engine.domain.resolver.strategy.impl;
import io.github.devsecops.engine.domain.resolver.model.PathList;
import io.github.devsecops.engine.domain.resolver.strategy.ResolverStrategy;
import org.apache.commons.io.FileUtils;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.Paths;
import java.util.Map;
import java.util.Optional;
import java.util.Properties;
import java.util.Set;
import java.util.stream.Collectors;
public class PropertyFileResolverStrategy implements ResolverStrategy {
private final Properties properties = new Properties();
public PropertyFileResolverStrategy(PathList pathList) throws IOException {
//TODO zero properties
pathList.getPaths().forEach(path -> {
if (path.isResource()) {
try (final InputStream resource = FileUtils.class.getResourceAsStream(path.getPath())){
properties.load(resource);
} catch (Exception e) {
System.out.println("Couldn't load file from resource " + path.getPath());
}
} else {
try (InputStream envStream = new FileInputStream(Paths.get(path.getPath()).toFile())) {
Properties envProperties = new Properties();
envProperties.load(envStream);
properties.putAll(envProperties);
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
});
propertiesReload();
}
private void propertiesReload() {
Map map = getAll();
Set keySet = map.keySet();
keySet.forEach(key -> {
String value = map.get(key);
Set secondKeySet = map.keySet();
secondKeySet.forEach(secondKey -> {
String regex = "${" + key + "}";
String secondValue = map.get(secondKey);
if (secondValue.contains(regex)) {
String replacedValue = secondValue.replace(regex, value);
map.replace(secondKey, replacedValue);
}
});
});
properties.clear();
properties.putAll(map);
}
@Override
public Optional resolve(String propertyName) {
return Optional.ofNullable(properties.getProperty(propertyName));
}
private Map getAll() {
return properties.entrySet().stream().collect(
Collectors.toMap(
k -> k.getKey().toString(),
v -> v.getValue().toString()
)
);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy