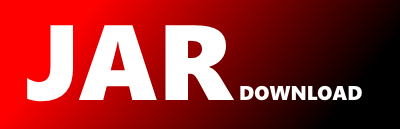
io.github.devsecops.engine.domain.sonar.model.Metric Maven / Gradle / Ivy
package io.github.devsecops.engine.domain.sonar.model;
import lombok.AllArgsConstructor;
import lombok.Getter;
import static io.github.devsecops.engine.domain.sonar.model.MetricTargetType.AT_LEAST;
import static io.github.devsecops.engine.domain.sonar.model.MetricTargetType.AT_MOST;
import static io.github.devsecops.engine.domain.sonar.model.MetricType.DOUBLE;
import static io.github.devsecops.engine.domain.sonar.model.MetricType.INTEGER;
import static io.github.devsecops.engine.domain.sonar.model.MetricType.RATING;
import static io.github.devsecops.engine.domain.sonar.model.SonarVariables.SONAR_MAX_BUGS;
import static io.github.devsecops.engine.domain.sonar.model.SonarVariables.SONAR_MAX_CODE_SMELLS;
import static io.github.devsecops.engine.domain.sonar.model.SonarVariables.SONAR_MAX_DUPLICATIONS;
import static io.github.devsecops.engine.domain.sonar.model.SonarVariables.SONAR_MAX_MAINTAINABILITY_RATING;
import static io.github.devsecops.engine.domain.sonar.model.SonarVariables.SONAR_MAX_RELIABILITY_RATING;
import static io.github.devsecops.engine.domain.sonar.model.SonarVariables.SONAR_MAX_VULNERABILITIES;
import static io.github.devsecops.engine.domain.sonar.model.SonarVariables.SONAR_MIN_COVERAGE;
import static io.github.devsecops.engine.domain.sonar.model.SonarVariables.SONAR_SECURITY_RATING;
@AllArgsConstructor
public enum Metric {
RELIABILITY_RATING("reliability_rating", RATING, AT_MOST, SONAR_MAX_RELIABILITY_RATING),
BUGS("bugs", INTEGER, AT_MOST, SONAR_MAX_BUGS),
SECURITY_RATING("security_rating", RATING, AT_MOST, SONAR_SECURITY_RATING),
VULNERABILITIES("vulnerabilities", INTEGER, AT_MOST, SONAR_MAX_VULNERABILITIES),
MAINTAINABILITY_RATING("sqale_rating", RATING, AT_MOST, SONAR_MAX_MAINTAINABILITY_RATING),
CODE_SMELLS("code_smells", INTEGER, AT_MOST, SONAR_MAX_CODE_SMELLS),
COVERAGE("coverage", DOUBLE, AT_LEAST, SONAR_MIN_COVERAGE),
DUPLICATIONS("duplicated_blocks", INTEGER, AT_MOST, SONAR_MAX_DUPLICATIONS);
@Getter
private String name;
@Getter
private MetricType type;
@Getter
private MetricTargetType targetType;
@Getter
private SonarVariables targetValueProperty;
public MetricReport evaluate(Integer value, Integer target) {
switch (targetType) {
case AT_LEAST:
return shouldBeAtLeast(value, target);
case AT_MOST:
default:
return shouldBeAtMost(value, target);
}
}
private MetricReport shouldBeAtMost(Integer value, Integer target) {
if (value <= target) {
return new MetricReport(true, String.format("'%s', the most allowed '%s'", value, target));
} else {
return new MetricReport(false, String.format("'%s' should be less than target '%s'", value, target));
}
}
private MetricReport shouldBeAtLeast(Integer value, Integer target) {
if (value >= target) {
return new MetricReport(true, String.format("'%s', the least allowed is '%s'", value, target));
} else {
return new MetricReport(false, String.format("'%s' should be at least as the target '%s'", value, target));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy