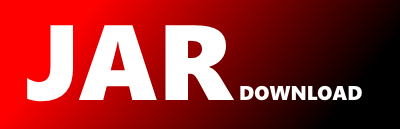
io.github.devsecops.engine.mojos.artifact.DeployArtifactFactory Maven / Gradle / Ivy
package io.github.devsecops.engine.mojos.artifact;
import io.github.devsecops.engine.core.CommandInvoker;
import io.github.devsecops.engine.core.Log;
import io.github.devsecops.engine.core.contract.Command;
import io.github.devsecops.engine.core.contract.Factory;
import io.github.devsecops.engine.core.contract.Invoker;
import io.github.devsecops.engine.domain.artifact.model.ArtifactRepositoryType;
import io.github.devsecops.engine.core.model.BuildParam;
import io.github.devsecops.engine.core.model.Environment;
import io.github.devsecops.engine.domain.resolver.path.PathResolverBuilder;
import io.github.devsecops.engine.domain.resolver.strategy.Resolver;
import io.github.devsecops.engine.domain.artifact.commands.DeployArtifactCommand;
import io.github.devsecops.engine.domain.git.commands.GitCommitAndPushPomCommand;
import io.github.devsecops.engine.domain.pom.commands.PomIncreaseVersionCommand;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Stack;
@Service
public class DeployArtifactFactory implements Factory {
private static final List RELEASES_ENV = Arrays.asList(Environment.UAT);
@Autowired
private Log logger;
@Autowired
private PathResolverBuilder pathResolverFactory;
@Autowired
private DeployArtifactCommand deployArtifactCommand;
@Autowired
private PomIncreaseVersionCommand pomIncreaseVersionCommand;
@Autowired
private GitCommitAndPushPomCommand gitCommitAndPushPomCommand;
@Override
public Invoker build(Map parameters) throws Exception {
final Resolver resolver = pathResolverFactory.build(parameters);
ArtifactRepositoryType repositoryType = getRepositoryType(parameters);
deployArtifactCommand.setRepositoryType(repositoryType);
deployArtifactCommand.setResolver(resolver);
Stack commands = new Stack<>();
if (Boolean.TRUE.equals(isToIncreasePom(parameters))) {
gitCommitAndPushPomCommand.setResolver(resolver);
commands.push(gitCommitAndPushPomCommand);
commands.push(pomIncreaseVersionCommand);
}
commands.push(deployArtifactCommand);
return new CommandInvoker(commands, logger);
}
private Boolean isToIncreasePom(Map parameters) {
String increaseVersion = parameters.get(BuildParam.INCREASE_VERSION.name());
return increaseVersion != null ? Boolean.valueOf(increaseVersion) : Boolean.FALSE;
}
private ArtifactRepositoryType getRepositoryType(Map parameters) {
Environment env = Environment.valueOf(parameters.get(BuildParam.ENV.name()).toUpperCase());
return RELEASES_ENV.contains(env) ? ArtifactRepositoryType.RELEASE : ArtifactRepositoryType.SNAPSHOT;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy