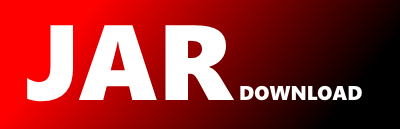
org.openea.eap.module.system.service.mail.MailAccountServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eap-module-system-biz Show documentation
Show all versions of eap-module-system-biz Show documentation
system 模块下,我们放通用业务,支撑上层的核心业务。
例如说:用户、部门、权限、数据字典等等
The newest version!
package org.openea.eap.module.system.service.mail;
import org.openea.eap.framework.common.pojo.PageResult;
import org.openea.eap.framework.common.util.object.BeanUtils;
import org.openea.eap.module.system.controller.admin.mail.vo.account.MailAccountPageReqVO;
import org.openea.eap.module.system.controller.admin.mail.vo.account.MailAccountSaveReqVO;
import org.openea.eap.module.system.dal.dataobject.mail.MailAccountDO;
import org.openea.eap.module.system.dal.mysql.mail.MailAccountMapper;
import org.openea.eap.module.system.dal.redis.RedisKeyConstants;
import lombok.extern.slf4j.Slf4j;
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
import org.springframework.validation.annotation.Validated;
import javax.annotation.Resource;
import java.util.List;
import static org.openea.eap.framework.common.exception.util.ServiceExceptionUtil.exception;
import static org.openea.eap.module.system.enums.ErrorCodeConstants.MAIL_ACCOUNT_NOT_EXISTS;
import static org.openea.eap.module.system.enums.ErrorCodeConstants.MAIL_ACCOUNT_RELATE_TEMPLATE_EXISTS;
/**
* 邮箱账号 Service 实现类
*
* @author wangjingyi
* @since 2022-03-21
*/
@Service
@Validated
@Slf4j
public class MailAccountServiceImpl implements MailAccountService {
@Resource
private MailAccountMapper mailAccountMapper;
@Resource
private MailTemplateService mailTemplateService;
@Override
public Long createMailAccount(MailAccountSaveReqVO createReqVO) {
MailAccountDO account = BeanUtils.toBean(createReqVO, MailAccountDO.class);
mailAccountMapper.insert(account);
return account.getId();
}
@Override
@CacheEvict(value = RedisKeyConstants.MAIL_ACCOUNT, key = "#updateReqVO.id")
public void updateMailAccount(MailAccountSaveReqVO updateReqVO) {
// 校验是否存在
validateMailAccountExists(updateReqVO.getId());
// 更新
MailAccountDO updateObj = BeanUtils.toBean(updateReqVO, MailAccountDO.class);
mailAccountMapper.updateById(updateObj);
}
@Override
@CacheEvict(value = RedisKeyConstants.MAIL_ACCOUNT, key = "#id")
public void deleteMailAccount(Long id) {
// 校验是否存在账号
validateMailAccountExists(id);
// 校验是否存在关联模版
if (mailTemplateService.getMailTemplateCountByAccountId(id) > 0) {
throw exception(MAIL_ACCOUNT_RELATE_TEMPLATE_EXISTS);
}
// 删除
mailAccountMapper.deleteById(id);
}
private void validateMailAccountExists(Long id) {
if (mailAccountMapper.selectById(id) == null) {
throw exception(MAIL_ACCOUNT_NOT_EXISTS);
}
}
@Override
public MailAccountDO getMailAccount(Long id) {
return mailAccountMapper.selectById(id);
}
@Override
@Cacheable(value = RedisKeyConstants.MAIL_ACCOUNT, key = "#id", unless = "#result == null")
public MailAccountDO getMailAccountFromCache(Long id) {
return getMailAccount(id);
}
@Override
public PageResult getMailAccountPage(MailAccountPageReqVO pageReqVO) {
return mailAccountMapper.selectPage(pageReqVO);
}
@Override
public List getMailAccountList() {
return mailAccountMapper.selectList();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy