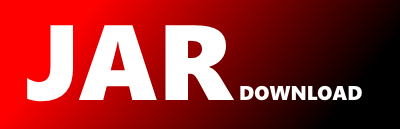
org.openea.eap.module.system.service.notify.NotifySendServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eap-module-system-biz Show documentation
Show all versions of eap-module-system-biz Show documentation
system 模块下,我们放通用业务,支撑上层的核心业务。
例如说:用户、部门、权限、数据字典等等
The newest version!
package org.openea.eap.module.system.service.notify;
import org.openea.eap.framework.common.enums.CommonStatusEnum;
import org.openea.eap.framework.common.enums.UserTypeEnum;
import org.openea.eap.module.system.dal.dataobject.notify.NotifyTemplateDO;
import com.google.common.annotations.VisibleForTesting;
import lombok.extern.slf4j.Slf4j;
import org.springframework.stereotype.Service;
import org.springframework.validation.annotation.Validated;
import javax.annotation.Resource;
import java.util.Map;
import java.util.Objects;
import static org.openea.eap.framework.common.exception.util.ServiceExceptionUtil.exception;
import static org.openea.eap.module.system.enums.ErrorCodeConstants.NOTICE_NOT_FOUND;
import static org.openea.eap.module.system.enums.ErrorCodeConstants.NOTIFY_SEND_TEMPLATE_PARAM_MISS;
/**
* 站内信发送 Service 实现类
*
* @author xrcoder
*/
@Service
@Validated
@Slf4j
public class NotifySendServiceImpl implements NotifySendService {
@Resource
private NotifyTemplateService notifyTemplateService;
@Resource
private NotifyMessageService notifyMessageService;
@Override
public Long sendSingleNotifyToAdmin(Long userId, String templateCode, Map templateParams) {
return sendSingleNotify(userId, UserTypeEnum.ADMIN.getValue(), templateCode, templateParams);
}
@Override
public Long sendSingleNotifyToMember(Long userId, String templateCode, Map templateParams) {
return sendSingleNotify(userId, UserTypeEnum.MEMBER.getValue(), templateCode, templateParams);
}
@Override
public Long sendSingleNotify(Long userId, Integer userType, String templateCode, Map templateParams) {
// 校验模版
NotifyTemplateDO template = validateNotifyTemplate(templateCode);
if (Objects.equals(template.getStatus(), CommonStatusEnum.DISABLE.getStatus())) {
log.info("[sendSingleNotify][模版({})已经关闭,无法给用户({}/{})发送]", templateCode, userId, userType);
return null;
}
// 校验参数
validateTemplateParams(template, templateParams);
// 发送站内信
String content = notifyTemplateService.formatNotifyTemplateContent(template.getContent(), templateParams);
return notifyMessageService.createNotifyMessage(userId, userType, template, content, templateParams);
}
@VisibleForTesting
public NotifyTemplateDO validateNotifyTemplate(String templateCode) {
// 获得站内信模板。考虑到效率,从缓存中获取
NotifyTemplateDO template = notifyTemplateService.getNotifyTemplateByCodeFromCache(templateCode);
// 站内信模板不存在
if (template == null) {
throw exception(NOTICE_NOT_FOUND);
}
return template;
}
/**
* 校验站内信模版参数是否确实
*
* @param template 邮箱模板
* @param templateParams 参数列表
*/
@VisibleForTesting
public void validateTemplateParams(NotifyTemplateDO template, Map templateParams) {
template.getParams().forEach(key -> {
Object value = templateParams.get(key);
if (value == null) {
throw exception(NOTIFY_SEND_TEMPLATE_PARAM_MISS, key);
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy