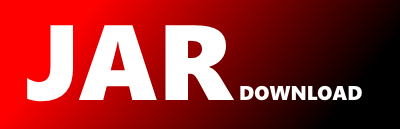
org.openea.eap.module.system.service.permission.MenuServiceImpl Maven / Gradle / Ivy
Show all versions of eap-module-system-biz Show documentation
package org.openea.eap.module.system.service.permission;
import cn.hutool.core.collection.CollUtil;
import cn.hutool.core.util.ObjUtil;
import cn.hutool.core.util.ObjectUtil;
import cn.hutool.core.util.StrUtil;
import cn.hutool.json.JSONObject;
import org.openea.eap.framework.common.enums.CommonStatusEnum;
import org.openea.eap.framework.common.util.object.BeanUtils;
import org.openea.eap.framework.i18n.core.I18nUtil;
import org.openea.eap.module.system.controller.admin.permission.vo.menu.MenuListReqVO;
import org.openea.eap.module.system.controller.admin.permission.vo.menu.MenuSaveVO;
import org.openea.eap.module.system.dal.dataobject.permission.MenuDO;
import org.openea.eap.module.system.dal.mysql.permission.MenuMapper;
import org.openea.eap.module.system.dal.redis.RedisKeyConstants;
import org.openea.eap.module.system.enums.permission.MenuTypeEnum;
import org.openea.eap.module.system.service.language.I18nDataService;
import org.openea.eap.module.system.service.language.I18nJsonDataService;
import org.openea.eap.module.system.service.tenant.TenantService;
import com.google.common.annotations.VisibleForTesting;
import com.google.common.collect.Lists;
import lombok.extern.slf4j.Slf4j;
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.context.annotation.Lazy;
import org.springframework.context.i18n.LocaleContextHolder;
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import javax.annotation.Resource;
import java.util.*;
import static org.openea.eap.framework.common.exception.util.ServiceExceptionUtil.exception;
import static org.openea.eap.framework.common.util.collection.CollectionUtils.convertList;
import static org.openea.eap.framework.common.util.collection.CollectionUtils.convertMap;
import static org.openea.eap.module.system.dal.dataobject.permission.MenuDO.ID_ROOT;
import static org.openea.eap.module.system.enums.ErrorCodeConstants.*;
/**
* 菜单 Service 实现
*
*/
@Service
@Slf4j
public class MenuServiceImpl implements MenuService {
@Resource
private MenuMapper menuMapper;
@Resource
private PermissionService permissionService;
@Resource
@Lazy // 延迟,避免循环依赖报错
private TenantService tenantService;
@Resource
@Lazy // 延迟,避免循环依赖报错
private I18nDataService i18nDataService;
@Resource
@Lazy // 延迟,避免循环依赖报错
private I18nJsonDataService i18nJsonDataService;
@Override
@CacheEvict(value = RedisKeyConstants.PERMISSION_MENU_ID_LIST, key = "#createReqVO.permission",
condition = "#createReqVO.permission != null")
public Long createMenu(MenuSaveVO createReqVO) {
// 校验父菜单存在
validateParentMenu(createReqVO.getParentId(), null);
// 校验菜单(自己)
validateMenu(createReqVO.getParentId(), createReqVO.getName(), null);
// 插入数据库
MenuDO menu = BeanUtils.toBean(createReqVO, MenuDO.class);
initMenuProperty(menu);
menuMapper.insert(menu);
// check i18n
checkMenuI18n(menu);
// 返回
return menu.getId();
}
@Override
@CacheEvict(value = RedisKeyConstants.PERMISSION_MENU_ID_LIST,
allEntries = true) // allEntries 清空所有缓存,因为 permission 如果变更,涉及到新老两个 permission。直接清理,简单有效
public void updateMenu(MenuSaveVO updateReqVO) {
// 校验更新的菜单是否存在
if (menuMapper.selectById(updateReqVO.getId()) == null) {
throw exception(MENU_NOT_EXISTS);
}
// 校验父菜单存在
validateParentMenu(updateReqVO.getParentId(), updateReqVO.getId());
// 校验菜单(自己)
validateMenu(updateReqVO.getParentId(), updateReqVO.getName(), updateReqVO.getId());
// 更新到数据库
MenuDO updateObj = BeanUtils.toBean(updateReqVO, MenuDO.class);
initMenuProperty(updateObj);
menuMapper.updateById(updateObj);
// check i18n
checkMenuI18n(updateObj);
}
@Override
@Transactional(rollbackFor = Exception.class)
@CacheEvict(value = RedisKeyConstants.PERMISSION_MENU_ID_LIST,
allEntries = true) // allEntries 清空所有缓存,因为此时不知道 id 对应的 permission 是多少。直接清理,简单有效
public void deleteMenu(Long id) {
// 校验是否还有子菜单
if (menuMapper.selectCountByParentId(id) > 0) {
throw exception(MENU_EXISTS_CHILDREN);
}
// 校验删除的菜单是否存在
if (menuMapper.selectById(id) == null) {
throw exception(MENU_NOT_EXISTS);
}
// 标记删除
menuMapper.deleteById(id);
// 删除授予给角色的权限
permissionService.processMenuDeleted(id);
}
@Override
public List getMenuList() {
return menuMapper.selectList();
}
@Override
public List getMenuListByTenant(MenuListReqVO reqVO) {
// 查询所有菜单,并过滤掉关闭的节点
List menus = getMenuList(reqVO);
// 开启多租户的情况下,需要过滤掉未开通的菜单
tenantService.handleTenantMenu(menuIds -> menus.removeIf(menu -> !CollUtil.contains(menuIds, menu.getId())));
return menus;
}
@Override
public List filterDisableMenus(List menuList) {
if (CollUtil.isEmpty(menuList)){
return Collections.emptyList();
}
Map menuMap = convertMap(menuList, MenuDO::getId);
// 遍历 menu 菜单,查找不是禁用的菜单,添加到 enabledMenus 结果
List enabledMenus = new ArrayList<>();
Set disabledMenuCache = new HashSet<>(); // 存下递归搜索过被禁用的菜单,防止重复的搜索
for (MenuDO menu : menuList) {
if (isMenuDisabled(menu, menuMap, disabledMenuCache)) {
continue;
}
enabledMenus.add(menu);
}
return enabledMenus;
}
private boolean isMenuDisabled(MenuDO node, Map menuMap, Set disabledMenuCache) {
// 如果已经判定是禁用的节点,直接结束
if (disabledMenuCache.contains(node.getId())) {
return true;
}
// 1. 遍历到 parentId 为根节点,则无需判断
Long parentId = node.getParentId();
if (ObjUtil.equal(parentId, ID_ROOT)) {
if (CommonStatusEnum.isDisable(node.getStatus())) {
disabledMenuCache.add(node.getId());
return true;
}
return false;
}
// 2. 继续遍历 parent 节点
MenuDO parent = menuMap.get(parentId);
if (parent == null || isMenuDisabled(parent, menuMap, disabledMenuCache)) {
disabledMenuCache.add(node.getId());
return true;
}
return false;
}
@Override
public List getMenuList(MenuListReqVO reqVO) {
return menuMapper.selectList(reqVO);
}
@Override
@Cacheable(value = RedisKeyConstants.PERMISSION_MENU_ID_LIST, key = "#permission")
public List getMenuIdListByPermissionFromCache(String permission) {
List menus = menuMapper.selectListByPermission(permission);
return convertList(menus, MenuDO::getId);
}
@Override
public MenuDO getMenu(Long id) {
MenuDO menu = menuMapper.selectById(id);
// query i18n
String i18nKey = getI18nKey(menu);
if(ObjectUtil.isNotEmpty(i18nKey)){
JSONObject json = i18nDataService.getI18nJsonByKey(i18nKey);
if(json!=null){
menu.setI18nJson(json.toString());
}
}
return menu;
}
@Override
public List getMenuList(Collection ids) {
// 当 ids 为空时,返回一个空的实例对象
if (CollUtil.isEmpty(ids)) {
return Lists.newArrayList();
}
return menuMapper.selectBatchIds(ids);
}
@Override
public List toI18n(List menus) {
if(!I18nUtil.enableI18n() || menus==null){
return menus;
}
for(MenuDO menu: menus){
String i18nKey = getI18nKey(menu);
String i18nLabel = I18nUtil.t(i18nKey, menu.getName());
checkMissI18nKey(menu, i18nLabel);
if(StrUtil.isNotEmpty(i18nLabel) && !i18nLabel.equals(menu.getName())){
menu.setName(i18nLabel);
}
}
return menus;
}
protected void checkMenuI18n(MenuDO menuDO){
if(!I18nUtil.enableI18n()){
return;
}
String i18nKey = getI18nKey(menuDO);
// 检查翻译是否已存在
if(i18nJsonDataService.checkI18nExist("menu", i18nKey)){
return;
}
try{
i18nJsonDataService.createI18nItem("menu", i18nKey, "menu-"+menuDO.getName(), menuDO.getName());
}catch (Exception e){
log.warn("create menu i18n(key="+i18nKey+") fail: " +e.getMessage());
}
}
@Override
@Async
public Integer updateMenuI18n() {
int count = 0;
// 1. prepare will i18n menus
// 范围包含db中菜单以及缺少翻译的菜单mapMissI18nMenu,两者有重复
List menuList = this.getMenuList();
Map mapCheckI18n = new HashMap<>();
for(MenuDO menu: menuList){
String alias = menu.getAlias();
if(ObjectUtil.isEmpty(alias)){
alias = checkAlias(menu);
if(ObjectUtil.isEmpty(alias)) {
alias = i18nDataService.convertEnKey(menu.getName(), "menu");
}
if(ObjectUtil.isNotEmpty(alias)) {
menu.setAlias(alias);
menuMapper.updateById(menu);
}
}
if(ObjectUtil.isNotEmpty(alias)) {
alias = menu.getType() + "__" + alias;
if(!mapCheckI18n.containsKey(alias)){
mapCheckI18n.put(alias, menu);
}
}
}
for(String key: mapMissI18nMenu.keySet()){
if(!mapCheckI18n.containsKey(key)){
mapCheckI18n.put(key, mapMissI18nMenu.get(key));
}
}
// 2. check i18n
i18nDataService.translateMenu(mapCheckI18n.values());
return count;
}
// 本地存储,如集群部署可存储到redis中
private Map mapMissI18nMenu = new Hashtable<>();
/**
* 国际化翻译补漏机制 - 检查是否需要补翻译
*
* 保持未翻译菜单由定时任务或其他操作执行翻译
*
* @param menu
* @param i18nLabel
*/
private void checkMissI18nKey(MenuDO menu, String i18nLabel){
// 排除默认语言,默认为中文
if("zh".equalsIgnoreCase(LocaleContextHolder.getLocale().getLanguage())){
return;
}
// 已有不同的翻译,无需处理
if(i18nLabel !=null && !i18nLabel.equals(menu.getName())){
return;
}
String alias = menu.getAlias();
if(ObjectUtil.isNotEmpty(alias)) {
alias = menu.getType() + "__" + alias;
if(!mapMissI18nMenu.containsKey(alias)){
mapMissI18nMenu.put(alias, menu);
}
}
}
public static String getI18nKey(MenuDO menu){
String i18nKey = null;
if(MenuTypeEnum.BUTTON.getType().equals(menu.getType())){
i18nKey = "button.";
}else{
i18nKey = "menu.";
}
String alias = checkAlias(menu);
if(ObjectUtil.isEmpty(alias) || "null".equalsIgnoreCase(alias)){
alias = null;
}
if(ObjectUtil.isNotEmpty(alias)){
i18nKey += alias;
}else{
i18nKey = null;
}
return i18nKey;
}
/**
* 校验父菜单是否合法
*
* 1. 不能设置自己为父菜单
* 2. 父菜单不存在
* 3. 父菜单必须是 {@link MenuTypeEnum#MENU} 菜单类型
*
* @param parentId 父菜单编号
* @param childId 当前菜单编号
*/
@VisibleForTesting
void validateParentMenu(Long parentId, Long childId) {
if (parentId == null || ID_ROOT.equals(parentId)) {
return;
}
// 不能设置自己为父菜单
if (parentId.equals(childId)) {
throw exception(MENU_PARENT_ERROR);
}
MenuDO menu = menuMapper.selectById(parentId);
// 父菜单不存在
if (menu == null) {
throw exception(MENU_PARENT_NOT_EXISTS);
}
// 父菜单必须是目录或者菜单类型
if (!MenuTypeEnum.DIR.getType().equals(menu.getType())
&& !MenuTypeEnum.MENU.getType().equals(menu.getType())) {
throw exception(MENU_PARENT_NOT_DIR_OR_MENU);
}
}
/**
* 校验菜单是否合法
*
* 1. 校验相同父菜单编号下,是否存在相同的菜单名
*
* @param name 菜单名字
* @param parentId 父菜单编号
* @param id 菜单编号
*/
@VisibleForTesting
void validateMenu(Long parentId, String name, Long id) {
MenuDO menu = menuMapper.selectByParentIdAndName(parentId, name);
if (menu == null) {
return;
}
// 如果 id 为空,说明不用比较是否为相同 id 的菜单
if (id == null) {
throw exception(MENU_NAME_DUPLICATE);
}
if (!menu.getId().equals(id)) {
throw exception(MENU_NAME_DUPLICATE);
}
}
/**
* 初始化菜单的通用属性。
*
* 例如说,只有目录或者菜单类型的菜单,才设置 icon
*
* @param menu 菜单
*/
private void initMenuProperty(MenuDO menu) {
// 菜单为按钮类型时,无需 component、icon、path 属性,进行置空
if (MenuTypeEnum.BUTTON.getType().equals(menu.getType())) {
menu.setComponent("");
menu.setComponentName("");
menu.setIcon("");
menu.setPath("");
}
// init alias for i18n
checkAlias(menu);
}
private static String checkAlias(MenuDO menu){
String alias = menu.getAlias();
if("null".equalsIgnoreCase(alias)){
alias = null;
}
if(ObjectUtil.isNotEmpty(alias)){
return alias;
}
if (MenuTypeEnum.BUTTON.getType().equals(menu.getType())) {
if(ObjectUtil.isNotEmpty(menu.getPermission()) ){
// 转驼峰格式, 只保留后面2部分
// system:user:query => userQuery
// infra:api-access-log:query => apiAccessLogQuery
alias = menu.getPermission();
int last = 0;
last = alias.lastIndexOf(":");
if(last>0){
last = alias.substring(0, last-1).lastIndexOf(":");
if(last>0){
alias = alias.substring(last+1, alias.length());
}
}
alias = alias.replaceAll(":","-");
alias = StrUtil.toCamelCase(alias,'-');
}
}
if(ObjectUtil.isEmpty(alias) && ObjectUtil.isNotEmpty(menu.getPath())){
if(!menu.getPath().startsWith("http")
&& !menu.getPath().startsWith("#")){
// /system 去首/
alias = menu.getPath();
if(alias.startsWith("/")){
alias = alias.substring(1, alias.length());
}
// 去除参数?
if(alias.indexOf("?")>0){
alias = alias.substring(0, alias.indexOf("?"));
}
// 转驼峰格式
alias = alias.replaceAll("/","-");
alias = StrUtil.toCamelCase(alias,'-');
}
}
if(ObjectUtil.isEmpty(alias)
&& MenuTypeEnum.MENU.getType().equals(menu.getType())){
// menu
if(ObjectUtil.isNotEmpty(menu.getComponentName()) ){
// 首字母改小写
alias = StrUtil.lowerFirst(menu.getComponentName());
}else if(ObjectUtil.isNotEmpty(menu.getComponent()) ) {
// 转驼峰格式 infra/job/index => infraJob
alias = menu.getComponent();
if(alias.endsWith("/index")){
alias = alias.substring(0, alias.length()-6);
}
alias = StrUtil.toCamelCase(alias,'/');
}
}
if(ObjectUtil.isNotEmpty(alias)){
menu.setAlias(alias);
}
return alias;
}
}