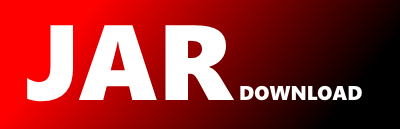
io.github.eb4j.pdic.DictionaryData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pdic4j Show documentation
Show all versions of pdic4j Show documentation
PDIC access library for java
/*
* DSL4J, a parser library for LingoDSL format.
* Copyright (C) 2021 Hiroshi Miura.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package io.github.eb4j.pdic;
import org.trie4j.MapTrie;
import org.trie4j.doublearray.MapDoubleArray;
import java.util.AbstractMap;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map.Entry;
/**
* A class that encapsulates the storage and retrieval of string-keyed data.
* Usage:
*
* - Retrieve data with {@link #lookUp(String)}
*
*
* @author Aaron Madlon-Kay
*
* @param
* The type of data stored
*/
public final class DictionaryData {
private final MapDoubleArray
© 2015 - 2024 Weber Informatics LLC | Privacy Policy