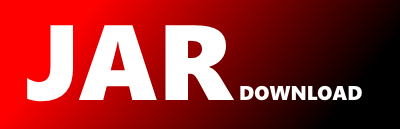
io.github.EdgeMetric.features.Workspaces Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mammoth-java-sdk Show documentation
Show all versions of mammoth-java-sdk Show documentation
SDK for integrating with Mammoth App
package io.github.EdgeMetric.features;
import java.util.List;
import io.github.EdgeMetric.mammoth.MammothConnector;
import io.github.EdgeMetric.mammoth.Utils;
import io.restassured.response.Response;
public class Workspaces {
private Utils utils = new Utils();
private MammothConnector mc;
/**
* APIs to handle workspaces in Mammoth.
* @param mc : MammothConnector object.
*/
public Workspaces(MammothConnector mc) {
this.mc = mc;
}
/**
* Gets specific workspace id.
* @param ds_id : The data-set id.
* @param index : Position of view to be enquired (starting from 0).
* @return A workspace id.
*/
public Object getWorkspaceID(Object ds_id, int index) {
List wksp_list = utils.commonHeader(mc.getToken(), mc.getAccountID())
.and()
.when()
.get("/datasets/" + ds_id + "/workspaces").jsonPath().get("workspaces.id");
return wksp_list.get(index);
}
/**
* Gets specific workspace data.
* @param wksp_id : The workspace id.
* @param index : Position of view to be enquired (starting from 0).
* @return Workspace data.
*/
public Response getWorkspaceData(Object wksp_id, int index) {
Response resp = utils.commonHeader(mc.getToken(), mc.getAccountID())
.and()
.when()
.get("/workspaces/" + wksp_id );
int futureID = resp.jsonPath().getInt("future_id");
Response final_resp = mc.trackFutureRequest(futureID);
return final_resp;
}
/**
* Adds specified task in the pipeline.
* @param body : task parameter, e.g label and rule as JSON.
* @param wksp_id : workspace id
*/
public void addTask(String body, Object wksp_id) {
utils.commonHeader(mc.getToken(), mc.getAccountID())
.and()
.body(body)
.when()
.post("/workspaces/" + wksp_id + "/tasks");
}
/**
* Adds actions and show in the pipeline.
* @param body : action parameter, e.g branch out.
* @param wksp_id : workspace id.
*/
public void addAction(String body, Object wksp_id) {
utils.commonHeader(mc.getToken(), mc.getAccountID())
.and()
.body(body)
.when()
.post("/workspaces/" + wksp_id + "/actions");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy