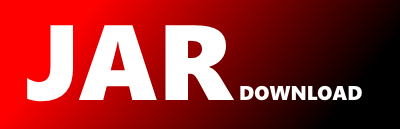
io.github.EdgeMetric.mammoth.MammothConnector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mammoth-java-sdk Show documentation
Show all versions of mammoth-java-sdk Show documentation
SDK for integrating with Mammoth App
package io.github.EdgeMetric.mammoth;
import static io.restassured.RestAssured.given;
import io.restassured.RestAssured;
import io.restassured.response.Response;
/**
* The main class for interacting with Mammoth Analytics API.
* @author Mammoth Analytics
*
*/
public class MammothConnector {
private Utils utils = new Utils();
private String token = null;
private String accountID = null;
/**
* Initializes the API URI and logs-in to Mammoth Account.
* @param email : The email of the user.
* @param password : The password of the user.
*/
public MammothConnector(String email, String password) {
RestAssured.baseURI = Constants.MAMMOTH_API_URI;
RestAssured.config().getRedirectConfig().followRedirects(true);
token = this.token(email, password);
accountID = this.accountID();
}
/**
* Gets account-id of the logged-in user.
* @return The id of the account for logged-in user.
*/
private String accountID() {
Response resp = given()
.header(Constants.contentType, Constants.appJson)
.header(Constants.xAPIKey, getToken())
.and()
.when()
.get("/accounts");
return resp.jsonPath().get("accounts[0].id").toString();
}
/**
* Gets token for the session.
* @param email : The email of the user.
* @param password : The password of the user.
* @return The token for the session.
*/
private String token(String email, String password) {
String body = "{\"email\":\"" + email + "\",\"password\":\"" + password + "\"}";
Response resp = given()
.header(Constants.contentType, Constants.appJson)
.and()
.body(body)
.when()
.post("/login");
return resp.jsonPath().get("token").toString();
}
/**
* Gets user id.
* @return A user-id.
*/
public String getUserID() {
Response resp = utils.commonHeader(getToken(), getAccountID())
.and()
.when()
.get("/accounts/" + getAccountID() + "/users");
return resp.jsonPath().get("users.id").toString();
}
public Response trackFutureRequest(Object future_id) {
Response resp = utils.commonHeader(getToken(), getAccountID())
.and()
.when()
.get("/future/" + future_id);
return resp;
}
public String getToken() {
return token;
}
public String getAccountID() {
return accountID;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy