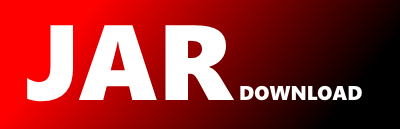
net.bioclipse.core.domain.StringMatrix Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2010 Egon Willighagen
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contact: http://www.bioclipse.net/
*******************************************************************************/
package net.bioclipse.core.domain;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.List;
import java.util.Map;
public class StringMatrix implements IStringMatrix {
private Map> matrix;
private int cols;
private int rows;
private Map rowHeaders;
private Map colHeaders;
public StringMatrix() {
cols = 0;
rows = 0;
}
private void checkDimensions(int row, int col) {
checkRows(row);
checkCols(col);
}
private void checkRows(int row) {
if (row < 0 || row > rows)
throw new ArrayIndexOutOfBoundsException(
"Incorrect row number: " + row
);
}
private void checkCols(int col) {
if (col < 0 || col > cols)
throw new ArrayIndexOutOfBoundsException(
"Incorrect column number: " + col
);
}
public String get(int row, int col) {
checkDimensions(row, col);
if (matrix == null) return "";
Map matrixRow = matrix.get(row);
if (matrixRow == null) return "";
return matrixRow.get(col);
}
public String get(int row, String col) {
checkRows(row);
return get(row, getColumnNumber(col));
}
public int getColumnCount() {
return this.cols;
}
public boolean hasColumn(String col) {
if (colHeaders == null) return false;
return colHeaders.containsValue(col);
}
public int getColumnNumber(String col) {
if (colHeaders != null) {
for (Integer colIndex : colHeaders.keySet()) {
String colname = colHeaders.get(colIndex);
if (colname != null && colname.equals(col))
return colIndex;
}
}
throw new IllegalAccessError(
"No column found with the label '" + col + "'."
);
}
public String getColumnName(int index) {
checkCols(index);
if (colHeaders == null) return "";
return colHeaders.get(index);
}
public int getRowCount() {
return this.rows;
}
public String getRowName(int index) {
checkRows(index);
if (rowHeaders == null) return "";
return rowHeaders.get(index);
}
public boolean hasColHeader() {
return colHeaders != null;
}
public boolean hasRowHeader() {
return rowHeaders != null;
}
public void set(int row, int col, String value) {
if (row > rows) rows = row;
if (col > cols) cols = col;
checkDimensions(row, col);
if (matrix == null)
matrix = new Hashtable>(row);
Map matrixRow = matrix.get(row);
if (matrixRow == null) {
matrixRow = new Hashtable(col);
matrix.put(row, matrixRow);
}
matrixRow.put(col, value);
}
public void setColumnName(int index, String name) {
if (index > cols) cols = index;
checkCols(index);
if (colHeaders == null)
colHeaders = new Hashtable();
colHeaders.put(index, name);
}
public void setRowName(int index, String name) {
checkRows(index);
if (rowHeaders == null)
rowHeaders = new Hashtable();
rowHeaders.put(index, name);
}
public void setSize(int row, int col) {
this.rows = row;
this.cols = col;
}
public void set(int row, String col, String value) {
checkRows(row);
set(row, getColumnNumber(col), value);
}
public List getRow(int row) {
checkRows(row);
int colCount = getColumnCount();
List results = new ArrayList(colCount);
for (int i=1; i<=colCount; i++) {
String result = get(row,i);
results.add(result == null ? "" : result);
}
return results;
}
public List getColumn(int col) {
checkCols(col);
int rowCount = getRowCount();
List results = new ArrayList(rowCount);
for (int i=1; i<=rowCount; i++) {
String result = get(i,col);
results.add(result == null ? "" : result);
}
return results;
}
public List getColumn(String col) {
int colNo = getColumnNumber(col);
return getColumn(colNo);
}
public String toString() {
StringBuffer buffer = new StringBuffer();
buffer.append('[');
if (hasColHeader()) {
buffer.append("[");
for (int col=1; col<=getColumnCount(); col++) {
buffer.append('"');
String result = getColumnName(col);
buffer.append(result == null ? "" : result);
buffer.append('"');
if (col getColumnNames() {
List names = new ArrayList(getColumnCount());
for (int i=1; i<=getColumnCount(); i++)
names.add(getColumnName(i));
return names;
}
@Override
public List getRowNames() {
List names = new ArrayList(getRowCount());
for (int i=1; i<=getRowCount(); i++)
names.add(getRowName(i));
return names;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy