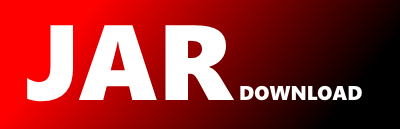
org.checkerframework.checker.optional.util.OptionalUtil Maven / Gradle / Ivy
Show all versions of checker Show documentation
package org.checkerframework.checker.optional.util;
import org.checkerframework.checker.optional.qual.EnsuresPresent;
import org.checkerframework.checker.optional.qual.MaybePresent;
import org.checkerframework.checker.optional.qual.Present;
import org.checkerframework.framework.qual.AnnotatedFor;
import java.util.Optional;
/**
* This is a utility class for the Optional Checker.
*
* To avoid the need to write the OptionalUtil class name, do:
*
*
import static org.checkerframework.checker.optional.util.OptionalUtil.castPresent;
*
* or
*
* import static org.checkerframework.checker.optional.util.OptionalUtil.*;
*
* Runtime Dependency: If you use this class, you must distribute (or link to) {@code
* checker-qual.jar}, along with your binaries. Or, you can copy this class into your own project.
*/
@SuppressWarnings({
"optional", // Optional utilities are trusted regarding the Optional type.
"cast" // Casts look redundant if Optional Checker is not run.
})
@AnnotatedFor("optional")
public final class OptionalUtil {
/** The OptionalUtil class should not be instantiated. */
private OptionalUtil() {
throw new AssertionError("do not instantiate");
}
/**
* A method that suppresses warnings from the Optional Checker.
*
*
The method takes a possibly-empty Optional reference, unsafely casts it to have
* the @Present type qualifier, and returns it. The Optional Checker considers both the return
* value, and also the argument, to be present after the method call. Therefore, the {@code
* castPresent} method can be used either as a cast expression or as a statement.
*
*
* // one way to use as a cast:
* {@literal @}Present String s = castPresent(possiblyEmpty1);
*
* // another way to use as a cast:
* castPresent(possiblyEmpty2).toString();
*
* // one way to use as a statement:
* castPresent(possiblyEmpty3);
* possiblyEmpty3.toString();
*
*
* The {@code castPresent} method is intended to be used in situations where the programmer
* definitively knows that a given Optional reference is present, but the type system is unable
* to make this deduction. It is not intended for defensive programming, in which a programmer
* cannot prove that the value is not empty but wishes to have an earlier indication if it is.
* See the Checker Framework Manual for further discussion.
*
* The method throws {@link AssertionError} if Java assertions are enabled and the argument
* is empty. If the exception is ever thrown, then that indicates that the programmer misused
* the method by using it in a circumstance where its argument can be empty.
*
* @param the type of content of the Optional
* @param ref an Optional reference of @MaybePresent type, that is present at run time
* @return the argument, casted to have the type qualifier @Present
*/
@EnsuresPresent("#1")
public static @Present Optional castPresent(
@MaybePresent Optional ref) {
assert ref.isPresent() : "Misuse of castPresent: called with an empty Optional";
return (@Present Optional) ref;
}
}