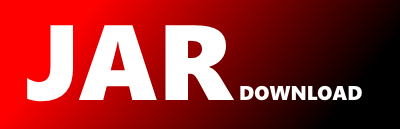
org.checkerframework.dataflow.cfg.block.RegularBlockImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of checker Show documentation
Show all versions of checker Show documentation
The Checker Framework enhances Java's type system to
make it more powerful and useful. This lets software developers
detect and prevent errors in their Java programs.
The Checker Framework includes compiler plug-ins ("checkers")
that find bugs or verify their absence. It also permits you to
write your own compiler plug-ins.
package org.checkerframework.dataflow.cfg.block;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.checkerframework.dataflow.cfg.node.Node;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/** Implementation of a regular basic block. */
public class RegularBlockImpl extends SingleSuccessorBlockImpl implements RegularBlock {
/** Internal representation of the contents. */
protected final List contents;
/**
* Initialize an empty basic block to be filled with contents and linked to other basic blocks
* later.
*/
public RegularBlockImpl() {
super(BlockType.REGULAR_BLOCK);
contents = new ArrayList<>();
}
/** Add a node to the contents of this basic block. */
public void addNode(Node t) {
contents.add(t);
t.setBlock(this);
}
/** Add multiple nodes to the contents of this basic block. */
public void addNodes(List extends Node> ts) {
for (Node t : ts) {
addNode(t);
}
}
/**
* {@inheritDoc}
*
* This implementation returns an non-empty list.
*/
@Override
public List getNodes() {
return Collections.unmodifiableList(contents);
}
@Override
public @Nullable Node getLastNode() {
return contents.get(contents.size() - 1);
}
@Override
public @Nullable BlockImpl getRegularSuccessor() {
return successor;
}
@Override
public String toString() {
return "RegularBlock(" + contents + ")";
}
@Override
public boolean isEmpty() {
return contents.isEmpty();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy