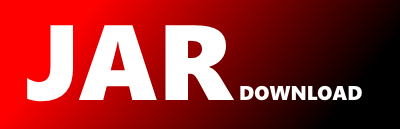
org.checkerframework.dataflow.cfg.node.SynchronizedNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of checker Show documentation
Show all versions of checker Show documentation
The Checker Framework enhances Java's type system to
make it more powerful and useful. This lets software developers
detect and prevent errors in their Java programs.
The Checker Framework includes compiler plug-ins ("checkers")
that find bugs or verify their absence. It also permits you to
write your own compiler plug-ins.
package org.checkerframework.dataflow.cfg.node;
import com.sun.source.tree.SynchronizedTree;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.checkerframework.dataflow.qual.SideEffectFree;
import java.util.Collection;
import java.util.Collections;
import java.util.Objects;
import javax.lang.model.type.TypeKind;
import javax.lang.model.util.Types;
/**
* This represents the start and end of a synchronized code block. If startOfBlock == true it is the
* node preceding a synchronized code block. Otherwise it is the node immediately after a
* synchronized code block.
*/
public class SynchronizedNode extends Node {
protected final SynchronizedTree tree;
protected final Node expression;
protected final boolean startOfBlock;
public SynchronizedNode(
SynchronizedTree tree, Node expression, boolean startOfBlock, Types types) {
super(types.getNoType(TypeKind.NONE));
this.tree = tree;
this.expression = expression;
this.startOfBlock = startOfBlock;
}
@Override
public SynchronizedTree getTree() {
return tree;
}
public Node getExpression() {
return expression;
}
public boolean getIsStartOfBlock() {
return startOfBlock;
}
@Override
public R accept(NodeVisitor visitor, P p) {
return visitor.visitSynchronized(this, p);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("synchronized (");
sb.append(expression);
sb.append(")");
return sb.toString();
}
@Override
public boolean equals(@Nullable Object obj) {
if (!(obj instanceof SynchronizedNode)) {
return false;
}
SynchronizedNode other = (SynchronizedNode) obj;
return Objects.equals(getTree(), other.getTree())
&& getExpression().equals(other.getExpression())
&& startOfBlock == other.startOfBlock;
}
@Override
public int hashCode() {
return Objects.hash(tree, startOfBlock, getExpression());
}
@Override
@SideEffectFree
public Collection getOperands() {
return Collections.emptyList();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy