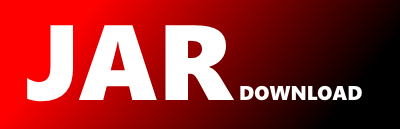
org.checkerframework.dataflow.cfg.node.SynchronizedNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dataflow-shaded Show documentation
Show all versions of dataflow-shaded Show documentation
dataflow-shaded is a dataflow framework based on the javac compiler.
It differs from the org.checkerframework:dataflow artifact in two ways.
First, the packages in this artifact have been renamed to org.checkerframework.shaded.*.
Second, unlike the dataflow artifact, this artifact contains the dependencies it requires.
package org.checkerframework.dataflow.cfg.node;
import com.sun.source.tree.SynchronizedTree;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.checkerframework.dataflow.qual.SideEffectFree;
import java.util.Collection;
import java.util.Collections;
import java.util.Objects;
import javax.lang.model.type.TypeKind;
import javax.lang.model.util.Types;
/**
* This represents the start and end of a synchronized code block. If startOfBlock == true it is the
* node preceding a synchronized code block. Otherwise it is the node immediately after a
* synchronized code block.
*/
public class SynchronizedNode extends Node {
protected final SynchronizedTree tree;
protected final Node expression;
protected final boolean startOfBlock;
public SynchronizedNode(
SynchronizedTree tree, Node expression, boolean startOfBlock, Types types) {
super(types.getNoType(TypeKind.NONE));
this.tree = tree;
this.expression = expression;
this.startOfBlock = startOfBlock;
}
@Override
public SynchronizedTree getTree() {
return tree;
}
public Node getExpression() {
return expression;
}
public boolean getIsStartOfBlock() {
return startOfBlock;
}
@Override
public R accept(NodeVisitor visitor, P p) {
return visitor.visitSynchronized(this, p);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("synchronized (");
sb.append(expression);
sb.append(")");
return sb.toString();
}
@Override
public boolean equals(@Nullable Object obj) {
if (!(obj instanceof SynchronizedNode)) {
return false;
}
SynchronizedNode other = (SynchronizedNode) obj;
return Objects.equals(getTree(), other.getTree())
&& getExpression().equals(other.getExpression())
&& startOfBlock == other.startOfBlock;
}
@Override
public int hashCode() {
return Objects.hash(tree, startOfBlock, getExpression());
}
@Override
@SideEffectFree
public Collection getOperands() {
return Collections.emptyList();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy