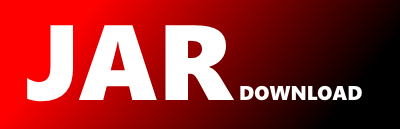
io.github.ericdriggs.reportcard.model.TestResultModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reportcard-model Show documentation
Show all versions of reportcard-model Show documentation
test report metrics and trend analysis reporting :: reportcard-model
package io.github.ericdriggs.reportcard.model;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSetter;
import io.github.ericdriggs.reportcard.mappers.SharedObjectMappers;
import io.github.ericdriggs.reportcard.xml.IsEmptyUtil;
import io.github.ericdriggs.reportcard.xml.ResultCount;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.*;
@JsonInclude(JsonInclude.Include.NON_NULL)
public class TestResultModel extends io.github.ericdriggs.reportcard.dto.TestResult {
private List testSuites = new ArrayList<>();
@JsonProperty("testSuites")
public List getTestSuites() {
return testSuites;
}
@JsonProperty("testCases")
public TestResultModel setTestSuites(List testSuites) {
this.testSuites = testSuites;
this.updateTotalsFromTestSuites();
return this;
}
@JsonIgnore
public static String asJson(TestResultModel testResultModel) {
if(testResultModel == null) {
return null;
}
try {
return mapper.writeValueAsString(testResultModel);
} catch (JsonProcessingException e) {
log.error("failed to parse json for testResultModel: {}", testResultModel, e);
}
return null;
}
@JsonIgnore
final static Logger log = LoggerFactory.getLogger(TestResultModel.class);
@JsonIgnore
final static ObjectMapper mapper = SharedObjectMappers.ignoreUnknownObjectMapper;
@JsonIgnore
public TestResultModel addTestSuite(TestSuiteModel testSuite) {
this.testSuites.add(testSuite);
return this;
}
@JsonIgnore
public TestResultModel() {
}
@JsonIgnore
public TestResultModel(List from) {
this.testSuites = new ArrayList<>(from);
this.updateTotalsFromTestSuites();
}
@JsonIgnore
public TestResultModel copy() {
return new TestResultModel(this.getTestSuites());
}
@JsonIgnore
public void updateTotalsFromTestSuites() {
ResultCount resultCount = getResultCount();
this.setError(resultCount.getErrors());
this.setFailure(resultCount.getFailures());
this.setSkipped(resultCount.getSkipped());
this.setTests(resultCount.getTests());
this.setTime(resultCount.getTime());
this.setHasSkip(resultCount.getSkipped() > 0);
this.setIsSuccess(resultCount.getErrors() == 0 && resultCount.getFailures() == 0 );
}
@JsonIgnore
public TestResultModel setExternalLinksMap(Map externalLinksMap) {
this.setExternalLinks(getExternalLinksJson(externalLinksMap));
return this;
}
/**
* Gets value from super or calculates it (super may be null
if row just inserted)
* @return whether was successful
*/
@Override
@JsonIgnore
public Boolean getIsSuccess() {
if (super.getIsSuccess() != null) {
return super.getIsSuccess();
} else {
int failure = Objects.requireNonNullElse(super.getFailure(), 0);
int error = Objects.requireNonNullElse(super.getError(), 0);
int skipped = Objects.requireNonNullElse(super.getSkipped(), 0);
return failure + error + skipped == 0;
}
}
/**
* Gets value from super or calculates it (super may be null
if row just inserted)
* @return whether has skip
*/
@Override
@JsonIgnore
public Boolean getHasSkip() {
if (super.getHasSkip() != null) {
return super.getHasSkip();
} else {
int skipped = Objects.requireNonNullElse(super.getSkipped(), 0);
return skipped > 0;
}
}
@JsonIgnore
protected String getExternalLinksJson(Map externalLinksMap) {
if (externalLinksMap == null) {
return null;
}
try {
return mapper.writerWithDefaultPrettyPrinter()
.writeValueAsString(externalLinksMap);
} catch (JsonProcessingException e) {
throw new RuntimeException(e);
}
}
@JsonIgnore
public ResultCount getResultCount() {
ResultCount resultCount = ResultCount.builder().build();
for (TestSuiteModel testSuite : testSuites) {
ResultCount testSuiteResultCount = testSuite.getResultCount();
resultCount = ResultCount.add(resultCount, testSuiteResultCount);
}
return resultCount;
}
@JsonIgnore
public TestResultModel add(TestResultModel that) {
if (that == null ) {
throw new NullPointerException("that");
}
if (that.getTestSuites() == null) {
throw new NullPointerException("that.getTestSuites()");
}
List combined = new ArrayList<>(this.getTestSuites());
combined.addAll(that.getTestSuites());
return new TestResultModel(combined);
}
@JsonIgnore
public Map> getTestCasesSkipped() {
Map> matched = new TreeMap<>(ModelComparators.TEST_SUITE_CASE_INSENSITIVE_ORDER);
for(TestSuiteModel testSuite : testSuites) {
for (TestCaseModel testCase : testSuite.getTestCases()) {
if (testCase.getTestStatus().isSkipped()) {
matched.computeIfAbsent(testSuite, k -> new ArrayList<>());
matched.get(testSuite).add(testCase);
}
}
}
return matched;
}
@JsonIgnore
public Map> getTestCasesErrorOrFailure() {
Map> matched = new TreeMap<>(ModelComparators.TEST_SUITE_CASE_INSENSITIVE_ORDER);
for(TestSuiteModel testSuite : testSuites) {
for (TestCaseModel testCase : testSuite.getTestCases()) {
if (testCase.getTestStatus().isErrorOrFailure()) {
matched.computeIfAbsent(testSuite, k -> new ArrayList<>());
matched.get(testSuite).add(testCase);
}
}
}
return matched;
}
@JsonIgnore
public Map> getTestCasesWithFaults() {
Map> matched = new TreeMap<>(ModelComparators.TEST_SUITE_CASE_INSENSITIVE_ORDER);
for(TestSuiteModel testSuite : testSuites) {
for (TestCaseModel testCase : testSuite.getTestCases()) {
if (IsEmptyUtil.isCollectionEmpty(testCase.getTestCaseFaults())) {
matched.computeIfAbsent(testSuite, k -> new ArrayList<>());
matched.get(testSuite).add(testCase);
}
}
}
return matched;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy