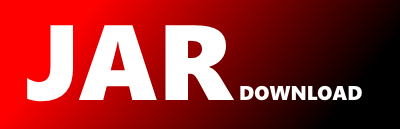
iosMain.com.estivensh4.firebase_crashlytics.FirebaseCrashlytics.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of firebase-crashlytics
Show all versions of firebase-crashlytics
FirebaseKMM is a Firebase Extension that supports cross-platform projects also based on Firebase Kotlin SDK, allowing you to directly from iOS and Android.
package com.estivensh4.firebase_crashlytics
import cocoapods.FirebaseCrashlytics.FIRCrashlytics
import com.estivensh4.firebase_app.Firebase
import kotlinx.cinterop.CPointer
import kotlinx.cinterop.ObjCObjectVar
import kotlinx.cinterop.alloc
import kotlinx.cinterop.convert
import kotlinx.cinterop.memScoped
import kotlinx.cinterop.pointed
import kotlinx.cinterop.ptr
import kotlinx.cinterop.value
import kotlinx.coroutines.CompletableDeferred
import platform.Foundation.NSError
import platform.Foundation.NSLocalizedDescriptionKey
import kotlin.native.concurrent.freeze
actual val Firebase.crashlytics
get() = FirebaseCrashlytics(FIRCrashlytics.crashlytics())
actual class FirebaseCrashlytics(private val iOS: FIRCrashlytics) {
/**
* Log.
*
* @param message Message
*/
actual fun log(message: String) {
iOS.log(message)
}
/**
* Delete unsent reports.
*/
actual fun deleteUnsentReports() {
iOS.deleteUnsentReports()
}
/**
* Set user id.
*
* @param userId User id
*/
actual fun setUserId(userId: String?) {
iOS.setUserID(userId)
}
/**
* Set custom key.
*
* @param key Key
* @param value Value
*/
actual fun setCustomKey(key: String, value: Any) {
iOS.setCustomValue(key, value.toString())
}
/**
* Set crashlytics collection enabled.
*
* @param enabled Enabled
*/
actual fun setCrashlyticsCollectionEnabled(enabled: Boolean) {
iOS.setCrashlyticsCollectionEnabled(enabled)
}
/**
* Set custom keys.
*
* @param customKeys Custom keys
*/
@Suppress("UNCHECKED_CAST")
actual fun setCustomKeys(customKeys: Map) {
iOS.setCustomKeysAndValues(customKeys as Map)
}
/**
* Record exception.
*
* @param exception Exception
*/
actual fun recordException(exception: Throwable) {
iOS.recordError(exception.asNSError())
}
/**
* Did crash on previous execution.
*
* @return
*/
actual fun didCrashOnPreviousExecution() = iOS.didCrashDuringPreviousExecution()
}
@OptIn(FreezingIsDeprecated::class)
internal fun Throwable.asNSError(): NSError {
val userInfo = mutableMapOf()
userInfo["KotlinException"] = this.freeze()
val message = message
if (message != null) {
userInfo[NSLocalizedDescriptionKey] = message
}
return NSError.errorWithDomain("KotlinException", 0.convert(), userInfo)
}
private suspend inline fun T.awaitResult(
function: T.(callback: (R?, NSError?) -> Unit) -> Unit
): R {
val job = CompletableDeferred()
function { result, error ->
if (error == null) {
job.complete(result)
} else {
job.completeExceptionally(Error(""))
}
}
return job.await() as R
}
suspend inline fun await(function: (callback: (NSError?) -> Unit) -> T): T {
val job = CompletableDeferred()
val result = function { error ->
if (error == null) {
job.complete(Unit)
} else {
job.completeExceptionally(Error(""))
}
}
job.await()
return result
}
internal fun T.throwError(block: T.(errorPointer: CPointer>) -> R): R {
memScoped {
val errorPointer: CPointer> = alloc>().ptr
val result = block(errorPointer)
val error: NSError? = errorPointer.pointed.value
if (error != null) {
throw Exception("")
}
return result
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy