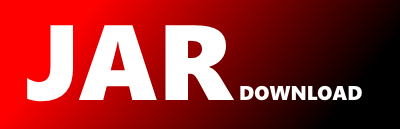
scala.com.amazonaws.kinesis.SubscribeToShardEventStream.scala Maven / Gradle / Ivy
Show all versions of kinesis4cats-smithy4s-client_sjs1_3 Show documentation
package com.amazonaws.kinesis
import smithy4s.Hints
import smithy4s.Schema
import smithy4s.ShapeId
import smithy4s.ShapeTag
import smithy4s.schema.Schema.bijection
import smithy4s.schema.Schema.union
/** This is a tagged union for all of the types of events an enhanced fan-out consumer can
* receive over HTTP/2 after a call to SubscribeToShard.
*/
sealed trait SubscribeToShardEventStream extends scala.Product with scala.Serializable {
@inline final def widen: SubscribeToShardEventStream = this
}
object SubscribeToShardEventStream extends ShapeTag.Companion[SubscribeToShardEventStream] {
val id: ShapeId = ShapeId("com.amazonaws.kinesis", "SubscribeToShardEventStream")
val hints: Hints = Hints(
smithy.api.Documentation("This is a tagged union for all of the types of events an enhanced fan-out consumer can\n receive over HTTP/2 after a call to SubscribeToShard.
"),
smithy.api.Streaming(),
)
/** After you call SubscribeToShard, Kinesis Data Streams sends events
* of this type to your consumer. For an example of how to handle these events, see Enhanced Fan-Out
* Using the Kinesis Data Streams API.
*/
case class SubscribeToShardEventCase(subscribeToShardEvent: SubscribeToShardEvent) extends SubscribeToShardEventStream
case class ResourceNotFoundExceptionCase(resourceNotFoundException: ResourceNotFoundException) extends SubscribeToShardEventStream
case class ResourceInUseExceptionCase(resourceInUseException: ResourceInUseException) extends SubscribeToShardEventStream
case class KMSDisabledExceptionCase(kMSDisabledException: KMSDisabledException) extends SubscribeToShardEventStream
case class KMSInvalidStateExceptionCase(kMSInvalidStateException: KMSInvalidStateException) extends SubscribeToShardEventStream
case class KMSAccessDeniedExceptionCase(kMSAccessDeniedException: KMSAccessDeniedException) extends SubscribeToShardEventStream
case class KMSNotFoundExceptionCase(kMSNotFoundException: KMSNotFoundException) extends SubscribeToShardEventStream
case class KMSOptInRequiredCase(kMSOptInRequired: KMSOptInRequired) extends SubscribeToShardEventStream
case class KMSThrottlingExceptionCase(kMSThrottlingException: KMSThrottlingException) extends SubscribeToShardEventStream
/** The processing of the request failed because of an unknown error, exception, or
* failure.
*/
case class InternalFailureExceptionCase(internalFailureException: InternalFailureException) extends SubscribeToShardEventStream
object SubscribeToShardEventCase {
val hints: Hints = Hints(
smithy.api.Documentation("After you call SubscribeToShard, Kinesis Data Streams sends events\n of this type to your consumer. For an example of how to handle these events, see Enhanced Fan-Out\n Using the Kinesis Data Streams API.
"),
)
val schema: Schema[SubscribeToShardEventCase] = bijection(SubscribeToShardEvent.schema.addHints(hints), SubscribeToShardEventCase(_), _.subscribeToShardEvent)
val alt = schema.oneOf[SubscribeToShardEventStream]("SubscribeToShardEvent")
}
object ResourceNotFoundExceptionCase {
val hints: Hints = Hints.empty
val schema: Schema[ResourceNotFoundExceptionCase] = bijection(ResourceNotFoundException.schema.addHints(hints), ResourceNotFoundExceptionCase(_), _.resourceNotFoundException)
val alt = schema.oneOf[SubscribeToShardEventStream]("ResourceNotFoundException")
}
object ResourceInUseExceptionCase {
val hints: Hints = Hints.empty
val schema: Schema[ResourceInUseExceptionCase] = bijection(ResourceInUseException.schema.addHints(hints), ResourceInUseExceptionCase(_), _.resourceInUseException)
val alt = schema.oneOf[SubscribeToShardEventStream]("ResourceInUseException")
}
object KMSDisabledExceptionCase {
val hints: Hints = Hints.empty
val schema: Schema[KMSDisabledExceptionCase] = bijection(KMSDisabledException.schema.addHints(hints), KMSDisabledExceptionCase(_), _.kMSDisabledException)
val alt = schema.oneOf[SubscribeToShardEventStream]("KMSDisabledException")
}
object KMSInvalidStateExceptionCase {
val hints: Hints = Hints.empty
val schema: Schema[KMSInvalidStateExceptionCase] = bijection(KMSInvalidStateException.schema.addHints(hints), KMSInvalidStateExceptionCase(_), _.kMSInvalidStateException)
val alt = schema.oneOf[SubscribeToShardEventStream]("KMSInvalidStateException")
}
object KMSAccessDeniedExceptionCase {
val hints: Hints = Hints.empty
val schema: Schema[KMSAccessDeniedExceptionCase] = bijection(KMSAccessDeniedException.schema.addHints(hints), KMSAccessDeniedExceptionCase(_), _.kMSAccessDeniedException)
val alt = schema.oneOf[SubscribeToShardEventStream]("KMSAccessDeniedException")
}
object KMSNotFoundExceptionCase {
val hints: Hints = Hints.empty
val schema: Schema[KMSNotFoundExceptionCase] = bijection(KMSNotFoundException.schema.addHints(hints), KMSNotFoundExceptionCase(_), _.kMSNotFoundException)
val alt = schema.oneOf[SubscribeToShardEventStream]("KMSNotFoundException")
}
object KMSOptInRequiredCase {
val hints: Hints = Hints.empty
val schema: Schema[KMSOptInRequiredCase] = bijection(KMSOptInRequired.schema.addHints(hints), KMSOptInRequiredCase(_), _.kMSOptInRequired)
val alt = schema.oneOf[SubscribeToShardEventStream]("KMSOptInRequired")
}
object KMSThrottlingExceptionCase {
val hints: Hints = Hints.empty
val schema: Schema[KMSThrottlingExceptionCase] = bijection(KMSThrottlingException.schema.addHints(hints), KMSThrottlingExceptionCase(_), _.kMSThrottlingException)
val alt = schema.oneOf[SubscribeToShardEventStream]("KMSThrottlingException")
}
object InternalFailureExceptionCase {
val hints: Hints = Hints(
smithy.api.Documentation("The processing of the request failed because of an unknown error, exception, or\n failure.
"),
)
val schema: Schema[InternalFailureExceptionCase] = bijection(InternalFailureException.schema.addHints(hints), InternalFailureExceptionCase(_), _.internalFailureException)
val alt = schema.oneOf[SubscribeToShardEventStream]("InternalFailureException")
}
implicit val schema: Schema[SubscribeToShardEventStream] = union(
SubscribeToShardEventCase.alt,
ResourceNotFoundExceptionCase.alt,
ResourceInUseExceptionCase.alt,
KMSDisabledExceptionCase.alt,
KMSInvalidStateExceptionCase.alt,
KMSAccessDeniedExceptionCase.alt,
KMSNotFoundExceptionCase.alt,
KMSOptInRequiredCase.alt,
KMSThrottlingExceptionCase.alt,
InternalFailureExceptionCase.alt,
){
case c: SubscribeToShardEventCase => SubscribeToShardEventCase.alt(c)
case c: ResourceNotFoundExceptionCase => ResourceNotFoundExceptionCase.alt(c)
case c: ResourceInUseExceptionCase => ResourceInUseExceptionCase.alt(c)
case c: KMSDisabledExceptionCase => KMSDisabledExceptionCase.alt(c)
case c: KMSInvalidStateExceptionCase => KMSInvalidStateExceptionCase.alt(c)
case c: KMSAccessDeniedExceptionCase => KMSAccessDeniedExceptionCase.alt(c)
case c: KMSNotFoundExceptionCase => KMSNotFoundExceptionCase.alt(c)
case c: KMSOptInRequiredCase => KMSOptInRequiredCase.alt(c)
case c: KMSThrottlingExceptionCase => KMSThrottlingExceptionCase.alt(c)
case c: InternalFailureExceptionCase => InternalFailureExceptionCase.alt(c)
}.withId(id).addHints(hints)
}