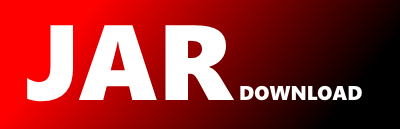
xjs.transform.JsonCollectors Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xjs-core Show documentation
Show all versions of xjs-core Show documentation
Core data layer and serlializers for XJS, an elegant JSON superset.
package xjs.transform;
import xjs.core.Json;
import xjs.core.JsonArray;
import xjs.core.JsonContainer;
import xjs.core.JsonObject;
import xjs.core.JsonReference;
import xjs.core.JsonValue;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collector;
import java.util.stream.Stream;
/**
* A series of {@link Collector collectors} used for generating regular
* {@link JsonContainer JSON containers} from {@link JsonValue JSON values}
* and other miscellaneous data.
*
* For example, to collect a {@link Stream} of integers into a {@link JsonArray}:
*
*
{@code
* Stream.of(1, 2, 3).collect(JsonCollectors.any())
* }
*
* Or to convert a regular {@link Map} into a {@link JsonObject};
*
*
{@code
* Map.of(
* Days.MONDAY, 1,
* Days.TUESDAY, 2,
* Days.WEDNESDAY, 3)
* .entrySet()
* .stream()
* .collect(
* JsonCollectors.toObject(
* Days::name,
* Json::any);
* }
*
* Or simply rely on automatic conversions:
*
*
{@code
* Map.of(
* "a", 1,
* "b", 2,
* "c", 3)
* .entrySet()
* .stream()
* .collect(JsonCollectors.toObject());
* }
*
* Finally, these collectors may be used to transform between type of containers.
* For example, to collect the values from an object into an array:
*
*
{@code}
* final JsonArray collected =
* object.values()
* .stream()
* .collect(JsonCollectors.value());
* }
*/
public final class JsonCollectors {
private JsonCollectors() {}
/**
* Generates a {@link Collector} collecting {@link JsonValue values} into
* {@link JsonArray arrays}.
*
* For example, to filter the values in an array into a new array:
*
*
{@code
* final JsonArray collected =
* array.stream()
* .filter(JsonValue::isNumber)
* .collect(Collectors.value());
* }
*
* @return The collector.
*/
public static Collector value() {
return Collector.of(
JsonArray::new,
JsonArray::add,
(left, right) -> { left.addAll(right); return left; },
Collector.Characteristics.IDENTITY_FINISH
);
}
/**
* Generates a {@link Collector} collecting {@link JsonReference references}
* into {@link JsonArray arrays}.
*
* @return The collector.
*/
public static Collector reference() {
return Collector.of(
JsonArray::new,
JsonArray::addReference,
(left, right) -> { left.addAll(right); return left; },
Collector.Characteristics.IDENTITY_FINISH
);
}
/**
* Generates a {@link Collector} collecting {@link JsonArray.Element elements}
* into {@link JsonArray arrays}.
*
* @return The collector.
*/
public static Collector element() {
return Collector.of(
JsonArray::new,
(array, element) -> array.addReference(element.getReference()),
(left, right) -> { left.addAll(right); return left; },
Collector.Characteristics.IDENTITY_FINISH
);
}
/**
* Generates a {@link Collector} collecting any simple values into
* {@link JsonArray arrays}.
*
* @return The collector.
* @see Json#any(Object)
*/
public static Collector
© 2015 - 2024 Weber Informatics LLC | Privacy Policy