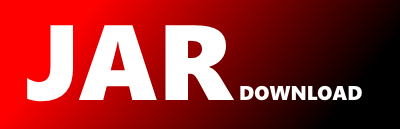
plus.extvos.restlet.service.BaseService Maven / Gradle / Ivy
The newest version!
package plus.extvos.restlet.service;
import com.baomidou.mybatisplus.core.conditions.Wrapper;
import com.baomidou.mybatisplus.core.toolkit.Constants;
import org.apache.ibatis.annotations.Param;
import plus.extvos.restlet.QuerySet;
import plus.extvos.common.exception.ResultException;
import java.io.Serializable;
import java.util.Collection;
import java.util.List;
import java.util.Map;
/**
* @author Mingcai SHEN
*/
public interface BaseService {
/**
* Insert a new entity
*
* @param entity object
* @return inserted num
* @throws ResultException for failures
*/
int insert(T entity) throws ResultException;
/**
* Insert multiple entities
*
* @param entities list of objects
* @return inserted num
* @throws ResultException for failures
*/
int insert(List entities) throws ResultException;
/**
* Replace entity: insert new on missing or update on exists
* @param entity of object
* @return inserted of updated num
* @throws ResultException for failure
*/
int replace(T entity) throws ResultException;
/**
* Replace entities: insert new on missing or update on exists
* @param entities of objects
* @return inserted of updated num
* @throws ResultException for failure
*/
int replace(List entities) throws ResultException;
/**
* Delete by id
*
* @param id as pk
* @return deleted num
* @throws ResultException for failures
*/
int deleteById(Serializable id) throws ResultException;
/**
* Delete by query map
*
* @param querySet of queries
* @return deleted num
* @throws ResultException for failures
*/
int deleteByMap(QuerySet querySet) throws ResultException;
/**
* Delete by map
*
* @param columnMap of query
* @return num of deleted
* @throws ResultException if error
*/
int deleteByMap(Map columnMap) throws ResultException;
/**
* Delete by wrapper
*
* @param queryWrapper of query
* @return num of deleted
* @throws ResultException if error
*/
int deleteByWrapper(Wrapper queryWrapper) throws ResultException;
/**
* Delete by ids,
*
* @param idList of id
* @return num of deleted
* @throws ResultException if error
*/
int deleteByIds(Collection extends Serializable> idList) throws ResultException;
/**
* update by id
*
* @param id as pk
* @param entity of object
* @return updated num
* @throws ResultException for failures
*/
int updateById(Serializable id, T entity) throws ResultException;
/**
* update by query map
*
* @param querySet as queries
* @param entity update fields
* @return updated num
* @throws ResultException for failures
*/
int updateByMap(QuerySet querySet, T entity) throws ResultException;
/**
* Update by map
*
* @param columnMap of query
* @param entity of object
* @return num ofupdated
* @throws ResultException when error
*/
int updateByMap(Map columnMap, T entity) throws ResultException;
/**
* Update by wrapper
*
* @param entity object
* @param updateWrapper of query
* @return updated records
* @throws ResultException if error
*/
int updateByWrapper(T entity, Wrapper updateWrapper) throws ResultException;
/**
* select by id
*
* @param querySet as queries
* @param id as pk
* @return entity
* @throws ResultException for failures
*/
T selectById(QuerySet querySet, Serializable id) throws ResultException;
/**
* select by id
*
* @param id as pk
* @return entity
* @throws ResultException for failures
*/
T selectById(Serializable id) throws ResultException;
/**
* select by queries
*
* @param querySet of queries
* @return list of entity
* @throws ResultException for failures
*/
List selectByMap(QuerySet querySet) throws ResultException;
/**
* Select by map
*
* @param columnMap of query
* @return list
* @throws ResultException for failures
*/
List selectByMap(Map columnMap) throws ResultException;
/**
* Select by wrapper
*
* @param queryWrapper pf query
* @return list
* @throws ResultException for failures
*/
List selectByWrapper(Wrapper queryWrapper) throws ResultException;
/**
* get count of by queries
*
* @param querySet of queries
* @return num
* @throws ResultException for failures
*/
Long countByMap(QuerySet querySet) throws ResultException;
/**
* Count by wrapper
*
* @param queryWrapper of query
* @return num
* @throws ResultException for failures
*/
Long countByWrapper(@Param(Constants.WRAPPER) Wrapper queryWrapper) throws ResultException;
/**
* select one entity by queries
*
* @param querySet of queries
* @return an entity
* @throws ResultException for failures
*/
T selectOne(QuerySet querySet) throws ResultException;
/**
* Select one by wrapper
*
* @param queryWrapper of query
* @return null of object
* @throws ResultException for failures
*/
T selectOne(Wrapper queryWrapper) throws ResultException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy