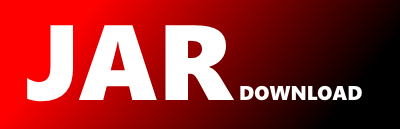
com.biz.verification.configuration.BizXVerificationConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of biz-all Show documentation
Show all versions of biz-all Show documentation
BizX 是一个灵活而高效的业务开发框架, 其中也有很多为业务开发所需要的工具类的提供。
The newest version!
package com.biz.verification.configuration;
import com.biz.verification.AbstractBizXCheckParameter;
import com.biz.verification.condition.CheckScanPackageCondition;
import com.biz.verification.factory.CheckParameterFactory;
import com.biz.verification.handler.*;
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.DependsOn;
/**
* BizX 校验入参配置类。
* 根据配置文件中的属性自动配置 {@link AbstractBizXCheckParameter} Bean。
*
* 该配置类在配置文件中存在 biz.verification.enabled=true
属性时才会被加载。
*
*
* 示例配置:
* biz.verification.enabled=true
*
*
* @author francis
* @version 1.0
* @see AbstractBizXCheckParameter
* @see CheckParameterFactory
* @see CheckScanPackageCondition
* @since 1.0.1
*/
@ConditionalOnProperty(prefix = "biz.verification", name = "enabled", havingValue = "true")
@Configuration
public class BizXVerificationConfiguration {
/**
* 创建并配置 {@link CheckParameterCollectionIsEmptyHandler} 校验处理器。
*
* @return {@link CheckParameterCollectionIsEmptyHandler} 实例
*/
@Bean
public CheckParameterCollectionIsEmptyHandler checkParameterCollectionIsEmptyHandler() {
return new CheckParameterCollectionIsEmptyHandler();
}
/**
* 创建并配置 {@link CheckParameterDateTimeFormatHandler} 校验处理器。
*
* @return {@link CheckParameterDateTimeFormatHandler} 实例
*/
@Bean
public CheckParameterDateTimeFormatHandler checkParameterDateTimeHandler() {
return new CheckParameterDateTimeFormatHandler();
}
/**
* 创建并配置 {@link CheckParameterDoubleMaxHandler} 校验处理器。
*
* @return {@link CheckParameterDoubleMaxHandler} 实例
*/
@Bean
public CheckParameterDoubleMaxHandler checkParameterDoubleMaxHandler() {
return new CheckParameterDoubleMaxHandler();
}
/**
* 创建并配置 {@link CheckParameterDoubleMinHandler} 校验处理器。
*
* @return {@link CheckParameterDoubleMinHandler} 实例
*/
@Bean
public CheckParameterDoubleMinHandler checkParameterDoubleMinHandler() {
return new CheckParameterDoubleMinHandler();
}
/**
* 创建并配置 {@link CheckParameterFloatMaxHandler} 校验处理器。
*
* @return {@link CheckParameterFloatMaxHandler} 实例
*/
@Bean
public CheckParameterFloatMaxHandler checkParameterFloatMaxHandler() {
return new CheckParameterFloatMaxHandler();
}
/**
* 创建并配置 {@link CheckParameterFloatMinHandler} 校验处理器。
*
* @return {@link CheckParameterFloatMinHandler} 实例
*/
@Bean
public CheckParameterFloatMinHandler checkParameterFloatMinHandler() {
return new CheckParameterFloatMinHandler();
}
/**
* 创建并配置 {@link CheckParameterIntegerMaxHandler} 校验处理器。
*
* @return {@link CheckParameterIntegerMaxHandler} 实例
*/
@Bean
public CheckParameterIntegerMaxHandler checkParameterIntegerMaxHandler() {
return new CheckParameterIntegerMaxHandler();
}
/**
* 创建并配置 {@link CheckParameterIntegerMinHandler} 校验处理器。
*
* @return {@link CheckParameterIntegerMinHandler} 实例
*/
@Bean
public CheckParameterIntegerMinHandler checkParameterIntegerMinHandler() {
return new CheckParameterIntegerMinHandler();
}
/**
* 创建并配置 {@link CheckParameterIsNullHandler} 校验处理器。
*
* @return {@link CheckParameterIsNullHandler} 实例
*/
@Bean
public CheckParameterIsNullHandler checkParameterIsNullHandler() {
return new CheckParameterIsNullHandler();
}
/**
* 创建并配置 {@link CheckParameterLongMaxHandler} 校验处理器。
*
* @return {@link CheckParameterLongMaxHandler} 实例
*/
@Bean
public CheckParameterLongMaxHandler checkParameterLongMaxHandler() {
return new CheckParameterLongMaxHandler();
}
/**
* 创建并配置 {@link CheckParameterLongMinHandler} 校验处理器。
*
* @return {@link CheckParameterLongMinHandler} 实例
*/
@Bean
public CheckParameterLongMinHandler checkParameterLongMinHandler() {
return new CheckParameterLongMinHandler();
}
/**
* 创建并配置 {@link CheckParameterShortMaxHandler} 校验处理器。
*
* @return {@link CheckParameterShortMaxHandler} 实例
*/
@Bean
public CheckParameterShortMaxHandler checkParameterShortMaxHandler() {
return new CheckParameterShortMaxHandler();
}
/**
* 创建并配置 {@link CheckParameterShortMinHandler} 校验处理器。
*
* @return {@link CheckParameterShortMinHandler} 实例
*/
@Bean
public CheckParameterShortMinHandler checkParameterShortMinHandler() {
return new CheckParameterShortMinHandler();
}
/**
* 创建并配置 {@link CheckParameterSizeHandler} 校验处理器。
*
* @return {@link CheckParameterSizeHandler} 实例
*/
@Bean
public CheckParameterSizeHandler checkParameterSizeHandler() {
return new CheckParameterSizeHandler();
}
/**
* 创建并配置 {@link CheckParameterFactory} 校验参数工厂 Bean。
*
* @return {@link CheckParameterFactory} 实例
*/
@Bean
@DependsOn({
"checkParameterCollectionIsEmptyHandler",
"checkParameterDateTimeHandler",
"checkParameterDoubleMaxHandler",
"checkParameterDoubleMinHandler",
"checkParameterFloatMaxHandler",
"checkParameterFloatMinHandler",
"checkParameterIntegerMaxHandler",
"checkParameterIntegerMinHandler",
"checkParameterIsNullHandler",
"checkParameterLongMaxHandler",
"checkParameterLongMinHandler",
"checkParameterShortMaxHandler",
"checkParameterShortMinHandler",
"checkParameterSizeHandler"
})
public CheckParameterFactory checkParameterFactory() {
return new CheckParameterFactory();
}
/**
* 创建并配置 {@link AbstractBizXCheckParameter} Bean。
*
* @return {@link AbstractBizXCheckParameter} 实例
*/
@Bean
public AbstractBizXCheckParameter bizXCheckParameter(CheckParameterFactory checkParameterFactory) {
return new AbstractBizXCheckParameter(checkParameterFactory);
}
/**
* 创建并配置 {@link CheckScanPackageCondition} 校验扫描包条件 Bean。
*
* @return {@link CheckScanPackageCondition} 实例
*/
@Bean
public CheckScanPackageCondition checkScanPackageCondition() {
return new CheckScanPackageCondition();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy