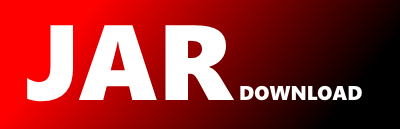
io.github.freya022.botcommands.internal.commands.application.SimpleCommandMap.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of BotCommands Show documentation
Show all versions of BotCommands Show documentation
A Kotlin-first (and Java) framework that makes creating Discord bots a piece of cake, using the JDA library.
package io.github.freya022.botcommands.internal.commands.application
import io.github.freya022.botcommands.api.commands.builder.IBuilderFunctionHolder
import io.github.freya022.botcommands.internal.IExecutableInteractionInfo
import io.github.freya022.botcommands.internal.commands.mixins.INamedCommand
import io.github.freya022.botcommands.internal.utils.shortSignature
import io.github.freya022.botcommands.internal.utils.throwUser
import java.util.*
import kotlin.reflect.KFunction
internal class SimpleCommandMap(private val functionSupplier: ((T) -> KFunction<*>)?) {
private val mutableMap: MutableMap = hashMapOf()
val map: Map
get() = Collections.unmodifiableMap(mutableMap)
fun putNewCommand(newCommand: T) {
mutableMap.putIfAbsent(newCommand.name, newCommand)?.let { oldCommand ->
throwUser(
"""
Command '${newCommand.path.fullPath}' is already defined
Existing command: ${functionSupplier?.invoke(oldCommand)?.shortSignature}
Current command: ${functionSupplier?.invoke(newCommand)?.shortSignature}
""".trimIndent()
)
}
}
fun isEmpty(): Boolean = mutableMap.isEmpty()
companion object {
fun ofBuilders(): SimpleCommandMap where T : INamedCommand,
T : IBuilderFunctionHolder<*> {
return SimpleCommandMap(IBuilderFunctionHolder<*>::function)
}
fun ofInfos(): SimpleCommandMap where T : INamedCommand,
T : IExecutableInteractionInfo {
return SimpleCommandMap(IExecutableInteractionInfo::function)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy