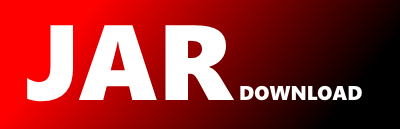
io.github.freya022.botcommands.internal.commands.application.autobuilder.SlashCommandAutoBuilder.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of BotCommands Show documentation
Show all versions of BotCommands Show documentation
A Kotlin-first (and Java) framework that makes creating Discord bots a piece of cake, using the JDA library.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy