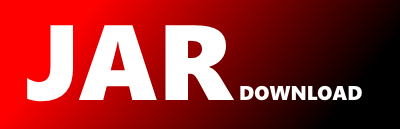
io.github.freya022.botcommands.internal.commands.text.TextCommandVariation.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of BotCommands Show documentation
Show all versions of BotCommands Show documentation
A Kotlin-first (and Java) framework that makes creating Discord bots a piece of cake, using the JDA library.
package io.github.freya022.botcommands.internal.commands.text
import io.github.freya022.botcommands.api.commands.ratelimit.CancellableRateLimit
import io.github.freya022.botcommands.api.commands.text.BaseCommandEvent
import io.github.freya022.botcommands.api.commands.text.CommandEvent
import io.github.freya022.botcommands.api.commands.text.TextCommandFilter
import io.github.freya022.botcommands.api.commands.text.builder.TextCommandVariationBuilder
import io.github.freya022.botcommands.api.core.BContext
import io.github.freya022.botcommands.api.core.Filter
import io.github.freya022.botcommands.api.core.utils.isSubclassOf
import io.github.freya022.botcommands.api.core.utils.simpleNestedName
import io.github.freya022.botcommands.internal.IExecutableInteractionInfo
import io.github.freya022.botcommands.internal.commands.application.slash.SlashUtils.getCheckedDefaultValue
import io.github.freya022.botcommands.internal.core.options.Option
import io.github.freya022.botcommands.internal.core.options.OptionType
import io.github.freya022.botcommands.internal.core.reflection.toMemberParamFunction
import io.github.freya022.botcommands.internal.parameters.CustomMethodOption
import io.github.freya022.botcommands.internal.transform
import io.github.freya022.botcommands.internal.utils.*
import io.github.oshai.kotlinlogging.KotlinLogging
import net.dv8tion.jda.api.events.message.MessageReceivedEvent
import kotlin.reflect.full.callSuspendBy
import kotlin.reflect.jvm.jvmErasure
private val logger = KotlinLogging.logger { }
class TextCommandVariation internal constructor(
private val context: BContext,
val info: TextCommandInfo,
builder: TextCommandVariationBuilder
) : IExecutableInteractionInfo {
override val eventFunction = builder.toMemberParamFunction(context)
override val parameters: List
val filters: List> = builder.filters.onEach { filter ->
require(!filter.global) {
"Global filter ${filter.javaClass.simpleNestedName} cannot be used explicitly, see ${Filter::global.reference}"
}
}
val description: String? = builder.description
val usage: String? = builder.usage
val example: String? = builder.example
val completePattern: Regex?
private val useTokenizedEvent: Boolean
init {
useTokenizedEvent = eventFunction.firstParameter.type.jvmErasure.isSubclassOf()
parameters = builder.optionAggregateBuilders.transform {
TextCommandParameter(context, it)
}
completePattern = when {
parameters.flatMap { it.allOptions }.any { it.optionType == OptionType.OPTION } -> CommandPattern.of(this)
else -> null
}
completePattern?.let {
logger.trace { "Registered text command variation '$it': ${function.shortSignature}" }
}
}
internal suspend fun createEvent(jdaEvent: MessageReceivedEvent, args: String, cancellableRateLimit: CancellableRateLimit): BaseCommandEvent = when {
useTokenizedEvent -> CommandEventImpl.create(context, jdaEvent, args, cancellableRateLimit)
else -> BaseCommandEventImpl(context, jdaEvent, args, cancellableRateLimit)
}
internal suspend fun tryParseOptionValues(event: BaseCommandEvent, args: String, matchResult: MatchResult?): Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy